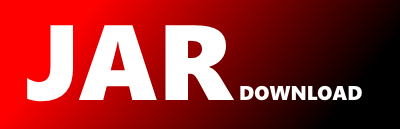
kotlinx.reflect.lite.KProperty.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx.reflect.lite Show documentation
Show all versions of kotlinx.reflect.lite Show documentation
Experimental lightweight library that replaces existing 'kotlin-reflect' implementation
The newest version!
/*
* Copyright 2016-2022 JetBrains s.r.o. Use of this source code is governed by the Apache 2.0 license.
*/
package kotlinx.reflect.lite
import kotlinx.reflect.lite.misc.*
/**
* Represents a property, such as a named `val` or `var` declaration.
*
* @param V the type of the property value.
*/
public interface KProperty : KCallable {
/**
* `true` if this property is `lateinit`.
* See the [Kotlin language documentation](https://kotlinlang.org/docs/reference/properties.html#late-initialized-properties)
* for more information.
*/
@SinceKotlin("1.1")
public val isLateInit: Boolean
/**
* `true` if this property is `const`.
* See the [Kotlin language documentation](https://kotlinlang.org/docs/reference/properties.html#compile-time-constants)
* for more information.
*/
@SinceKotlin("1.1")
public val isConst: Boolean
/** The getter of this property, used to obtain the value of the property. */
public val getter: Getter
/**
* Represents a property accessor, which is a `get` or `set` method declared alongside the property.
* See the [Kotlin language documentation](https://kotlinlang.org/docs/reference/properties.html#getters-and-setters)
* for more information.
*
* @param V the type of the property, which it is an accessor of.
*/
public interface Accessor {
/** The property which this accessor is originated from. */
public val property: KProperty
}
/**
* Getter of the property is a `get` method declared alongside the property.
*/
public interface Getter : Accessor, KFunction
}
/**
* Represents a property declared as a `var`.
*/
public interface KMutableProperty : KProperty {
/** The setter of this mutable property, used to change the value of the property. */
public val setter: Setter
/**
* Setter of the property is a `set` method declared alongside the property.
*/
public interface Setter : KProperty.Accessor, KFunction
}
/**
* Represents a property without any kind of receiver.
* Such property is either originally declared in a receiverless context such as a package,
* or has the receiver bound to it.
*/
public interface KProperty0 : KProperty, () -> V {
/**
* Returns the current value of the property.
*/
public fun get(): V
/**
* Returns the value of the delegate if this is a delegated property, or `null` if this property is not delegated.
* See the [Kotlin language documentation](https://kotlinlang.org/docs/reference/delegated-properties.html)
* for more information.
*/
@SinceKotlin("1.1")
private fun getDelegate(): Any? = notImplemented()
override val getter: Getter
/**
* Getter of the property is a `get` method declared alongside the property.
*
* Can be used as a function that takes 0 arguments and returns the value of the property type [V].
*/
public interface Getter : KProperty.Getter, () -> V
}
/**
* Represents a `var`-property without any kind of receiver.
*/
public interface KMutableProperty0 : KProperty0, KMutableProperty {
/**
* Modifies the value of the property.
*
* @param value the new value to be assigned to this property.
*/
public fun set(value: V)
override val setter: Setter
/**
* Setter of the property is a `set` method declared alongside the property.
*
* Can be used as a function that takes new property value as an argument and returns [Unit].
*/
public interface Setter : KMutableProperty.Setter, (V) -> Unit
}
/**
* Represents a property, operations on which take one receiver as a parameter.
*
* @param T the type of the receiver which should be used to obtain the value of the property.
* @param V the type of the property value.
*/
public interface KProperty1 : KProperty, (T) -> V {
/**
* Returns the current value of the property.
*
* @param receiver the receiver which is used to obtain the value of the property.
* For example, it should be a class instance if this is a member property of that class,
* or an extension receiver if this is a top level extension property.
*/
public fun get(receiver: T): V
/**
* Returns the value of the delegate if this is a delegated property, or `null` if this property is not delegated.
* See the [Kotlin language documentation](https://kotlinlang.org/docs/reference/delegated-properties.html)
* for more information.
*
* Note that for a top level **extension** property, the delegate is the same for all extension receivers,
* so the actual [receiver] instance passed in is not going to make any difference, it must only be a value of [T].
*
* @param receiver the receiver which is used to obtain the value of the property delegate.
* For example, it should be a class instance if this is a member property of that class,
* or an extension receiver if this is a top level extension property.
*
* @see [kotlin.reflect.full.getExtensionDelegate] // [KProperty1.getExtensionDelegate]
*/
@SinceKotlin("1.1")
private fun getDelegate(receiver: T): Any? = notImplemented()
override val getter: Getter
/**
* Getter of the property is a `get` method declared alongside the property.
*
* Can be used as a function that takes an argument of type [T] (the receiver) and returns the value of the property type [V].
*/
public interface Getter : KProperty.Getter, (T) -> V
}
/**
* Represents a `var`-property, operations on which take one receiver as a parameter.
*/
public interface KMutableProperty1 : KProperty1, KMutableProperty {
/**
* Modifies the value of the property.
*
* @param receiver the receiver which is used to modify the value of the property.
* For example, it should be a class instance if this is a member property of that class,
* or an extension receiver if this is a top level extension property.
* @param value the new value to be assigned to this property.
*/
public fun set(receiver: T, value: V)
override val setter: Setter
/**
* Setter of the property is a `set` method declared alongside the property.
*
* Can be used as a function that takes the receiver and the new property value as arguments and returns [Unit].
*/
public interface Setter : KMutableProperty.Setter, (T, V) -> Unit
}
/**
* Represents a property, operations on which take two receivers as parameters,
* such as an extension property declared in a class.
*
* @param D the type of the first receiver. In case of the extension property in a class this is
* the type of the declaring class of the property, or any subclass of that class.
* @param E the type of the second receiver. In case of the extension property in a class this is
* the type of the extension receiver.
* @param V the type of the property value.
*/
public interface KProperty2 : KProperty, (D, E) -> V {
/**
* Returns the current value of the property. In case of the extension property in a class,
* the instance of the class should be passed first and the instance of the extension receiver second.
*
* @param receiver1 the instance of the first receiver.
* @param receiver2 the instance of the second receiver.
*/
public fun get(receiver1: D, receiver2: E): V
/**
* Returns the value of the delegate if this is a delegated property, or `null` if this property is not delegated.
* See the [Kotlin language documentation](https://kotlinlang.org/docs/reference/delegated-properties.html)
* for more information.
*
* In case of the extension property in a class, the instance of the class should be passed first
* and the instance of the extension receiver second.
*
* @param receiver1 the instance of the first receiver.
* @param receiver2 the instance of the second receiver.
*
* @see [kotlin.reflect.full.getExtensionDelegate] // [KProperty2.getExtensionDelegate]
*/
@SinceKotlin("1.1")
private fun getDelegate(receiver1: D, receiver2: E): Any? = notImplemented()
override val getter: Getter
/**
* Getter of the property is a `get` method declared alongside the property.
*
* Can be used as a function that takes an argument of type [D] (the first receiver), an argument of type [E] (the second receiver)
* and returns the value of the property type [V].
*/
public interface Getter : KProperty.Getter, (D, E) -> V
}
/**
* Represents a `var`-property, operations on which take two receivers as parameters.
*/
public interface KMutableProperty2 : KProperty2, KMutableProperty {
/**
* Modifies the value of the property.
*
* @param receiver1 the instance of the first receiver.
* @param receiver2 the instance of the second receiver.
* @param value the new value to be assigned to this property.
*/
public fun set(receiver1: D, receiver2: E, value: V)
override val setter: Setter
/**
* Setter of the property is a `set` method declared alongside the property.
*
* Can be used as a function that takes an argument of type [D] (the first receiver), an argument of type [E] (the second receiver),
* and the new property value and returns [Unit].
*/
public interface Setter : KMutableProperty.Setter, (D, E, V) -> Unit
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy