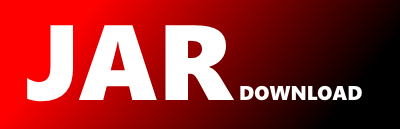
kotlinx.reflect.lite.impl.ReflectionLiteImpl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx.reflect.lite Show documentation
Show all versions of kotlinx.reflect.lite Show documentation
Experimental lightweight library that replaces existing 'kotlin-reflect' implementation
The newest version!
/*
* Copyright 2016-2022 JetBrains s.r.o. Use of this source code is governed by the Apache 2.0 license.
*/
package kotlinx.reflect.lite.impl
import kotlin.metadata.jvm.*
import kotlinx.reflect.lite.*
import kotlinx.reflect.lite.descriptors.ClassDescriptor
import kotlinx.reflect.lite.descriptors.PackageDescriptor
import kotlinx.reflect.lite.descriptors.impl.*
import kotlinx.reflect.lite.jvm.internal.*
import kotlinx.reflect.lite.jvm.internal.createCache
import kotlinx.reflect.lite.misc.*
internal object ReflectionLiteImpl {
private val kClassCache = createCache { createKotlinClass(it) }
fun createKotlinDeclarationContainer(jClass: Class): KDeclarationContainer {
return when (jClass.getMetadataAnnotation()?.kind) {
null, KotlinClassMetadata.CLASS_KIND -> getOrCreateKotlinClass(jClass)
KotlinClassMetadata.FILE_FACADE_KIND, KotlinClassMetadata.MULTI_FILE_CLASS_PART_KIND -> createKotlinPackage(jClass)
else -> throw KotlinReflectionInternalError("Can not load class metadata for $jClass")
}
}
@Suppress("UNCHECKED_CAST")
public fun getOrCreateKotlinClass(jClass: Class): KClass = kClassCache.get(jClass) as KClass
private fun createKotlinClass(jClass: Class): KClass {
val kind = jClass.getMetadataAnnotation()?.kind
return KClassImpl(createClassDescriptor(jClass, kind))
}
fun createKotlinPackage(jClass: Class): KPackage {
val kind = jClass.getMetadataAnnotation()?.kind
return KPackageImpl(createPackageDescriptor(jClass, kind))
}
private fun createClassDescriptor(jClass: Class, kind: Int?): ClassDescriptor =
when (kind) {
null -> {
if (RuntimeTypeMapper.getKotlinBuiltInClassId(jClass) != null) {
ClassDescriptorImpl(jClass)
} else {
JavaClassDescriptor(jClass)
}
}
KotlinClassMetadata.CLASS_KIND -> {
ClassDescriptorImpl(jClass)
}
else -> throw KotlinReflectionInternalError("Could not create an instance of KClass from $this, the class file kind equals $kind")
}
private fun createPackageDescriptor(jClass: Class, kind: Int?): PackageDescriptor =
when (kind) {
// we can extract KmPackage only from these kinds of kotlin class files: FILE_FACADE_KIND, MULTI_FILE_CLASS_PART_KIND
KotlinClassMetadata.FILE_FACADE_KIND, KotlinClassMetadata.MULTI_FILE_CLASS_PART_KIND -> PackageDescriptorImpl(jClass)
else -> throw KotlinReflectionInternalError("Could not create an instance of KPackage from $jClass, the class file kind equals $kind")
}
private fun Class.getMetadataAnnotation() = getAnnotation(Metadata::class.java)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy