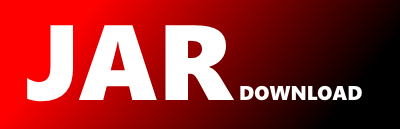
commonMain.jetbrains.datalore.base.gcommon.collect.Iterables.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2019 JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*
* This file has been modified by JetBrains : Java code has been converted to Kotlin code.
*
* THE FOLLOWING IS THE COPYRIGHT OF THE ORIGINAL DOCUMENT:
*
* Copyright (C) 2007 The Guava Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package jetbrains.datalore.base.gcommon.collect
import jetbrains.datalore.base.function.Predicate
object Iterables {
private fun checkNonNegative(position: Int) {
if (position < 0) {
throw IndexOutOfBoundsException(position.toString())
}
}
fun toList(iterable: Iterable): List {
return iterable.toList()
}
fun size(iterable: Iterable<*>): Int {
return iterable.count()
}
fun isEmpty(iterable: Iterable<*>): Boolean {
return (iterable as? Collection<*>)?.isEmpty() ?: !iterable.iterator().hasNext()
}
fun filter(unfiltered: Iterable, retainIfTrue: Predicate): Iterable {
return unfiltered.filter(retainIfTrue)
}
fun all(iterable: Iterable, predicate: Predicate): Boolean {
return iterable.all(predicate)
}
fun concat(a: Iterable, b: Iterable): Iterable {
return a + b
}
operator fun get(iterable: Iterable, position: Int): T {
checkNonNegative(position)
if (iterable is List<*>) {
return (iterable as List)[position]
}
val it = iterable.iterator()
for (i in 0..position) {
if (i == position) {
return it.next()
}
it.next()
}
throw IndexOutOfBoundsException(position.toString())
}
operator fun get(iterable: Iterable, position: Int, defaultValue: T): T {
checkNonNegative(position)
if (iterable is List<*>) {
val list = iterable as List
return if (position < list.size) list[position] else defaultValue
}
val it = iterable.iterator()
var i = 0
while (i <= position && it.hasNext()) {
if (i == position) {
return it.next()
}
it.next()
i++
}
return defaultValue
}
fun find(iterable: Iterable, predicate: Predicate, defaultValue: T): T {
return iterable.find(predicate) ?: defaultValue
}
fun getLast(iterable: Iterable): T {
return iterable.last()
}
internal fun toArray(iterable: Iterable<*>): Array<*> {
val collection: Collection<*>
if (iterable is Collection<*>) {
collection = iterable
} else {
collection = iterable.toList()
}
return collection.toTypedArray()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy