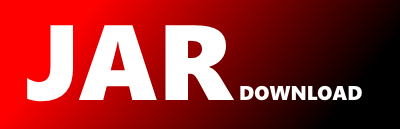
jvmMain.base.edt.RunnableWithAsync.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2019. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package jetbrains.datalore.base.edt
import jetbrains.datalore.base.async.Async
import jetbrains.datalore.base.function.Consumer
import jetbrains.datalore.base.function.Runnable
import jetbrains.datalore.base.function.Supplier
import jetbrains.datalore.base.registration.Registration
actual class RunnableWithAsync
private actual constructor(
action: Runnable,
async: SafeAsync) :
Runnable, Async {
actual companion object {
actual fun fromRunnable(r: Runnable): RunnableWithAsync {
val s = object : Supplier {
override fun get(): Unit {
r.run()
// return null
}
}
return fromSupplier(s)
}
actual fun fromSupplier(s: Supplier): RunnableWithAsync {
val safeAsync = SafeAsync()
return RunnableWithAsync(successFromPlain(s, safeAsync), safeAsync)
}
actual fun fromAsyncSupplier(s: Supplier>): RunnableWithAsync {
val safeAsync = SafeAsync()
return RunnableWithAsync(successFromAsync(s, safeAsync), safeAsync)
}
private fun successFromPlain(s: Supplier, safeAsync: SafeAsync): Runnable {
return object : Runnable {
override fun run() {
safeAsync.success(s.get())
}
}
}
private fun successFromAsync(s: Supplier>, safeAsync: SafeAsync): Runnable {
return object : Runnable {
override fun run() {
safeAsync.delegate(s.get())
}
}
}
}
private val myAction = action
private val myAsync = async
override fun run() {
try {
myAction.run()
} catch (t: Throwable) {
myAsync.fail(t)
throw t
}
}
override fun onSuccess(successHandler: Consumer): Registration {
return myAsync.onSuccess(successHandler)
}
override fun onResult(successHandler: Consumer, failureHandler: Consumer): Registration {
return myAsync.onResult(successHandler, failureHandler)
}
override fun onFailure(failureHandler: Consumer): Registration {
return myAsync.onFailure(failureHandler)
}
override fun map(success: (ItemT) -> ResultT): Async {
return myAsync.map(success)
}
override fun flatMap(success: (ItemT) -> Async?): Async {
return myAsync.flatMap(success)
}
fun fail() {
myAsync.fail(EdtException(RuntimeException("Intentionally failed")))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy