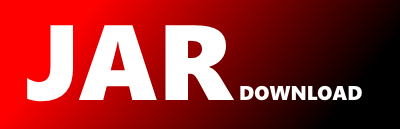
commonMain.jetbrains.datalore.plot.builder.PlotContainerPortable.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2020. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package jetbrains.datalore.plot.builder
import jetbrains.datalore.base.geometry.DoubleVector
import jetbrains.datalore.base.registration.CompositeRegistration
import jetbrains.datalore.base.registration.Registration
import jetbrains.datalore.base.values.SomeFig
import jetbrains.datalore.plot.builder.presentation.Style
import jetbrains.datalore.plot.builder.presentation.Style.PLOT_BACKDROP
import jetbrains.datalore.vis.svg.SvgCssResource
import jetbrains.datalore.vis.svg.SvgRectElement
import jetbrains.datalore.vis.svg.SvgSvgElement
/**
* This class only handles static SVG. (no interactions)
*/
open class PlotContainerPortable(
protected val plot: PlotSvgComponent,
plotSize: DoubleVector
) {
val svg: SvgSvgElement = SvgSvgElement()
val liveMapFigures: List
get() = plot.liveMapFigures
val isLiveMap: Boolean
get() = plot.liveMapFigures.isNotEmpty()
private var myContentBuilt: Boolean = false
private var myRegistrations = CompositeRegistration()
init {
svg.addClass(Style.PLOT_CONTAINER)
setSvgSize(plotSize)
plot.resize(plotSize)
}
fun ensureContentBuilt() {
if (!myContentBuilt) {
buildContent()
}
}
fun resize(plotSize: DoubleVector) {
if (plotSize.x <= 0 || plotSize.y <= 0) return
if (plotSize == plot.plotSize) return
// Invalidate
clearContent()
setSvgSize(plotSize)
plot.resize(plotSize)
}
// private fun revalidateContent() {
// if (myContentBuilt) {
// clearContent()
// buildContent()
// }
// }
protected open fun buildContent() {
check(!myContentBuilt)
myContentBuilt = true
svg.setStyle(object : SvgCssResource {
override fun css(): String {
return Style.css
}
})
// Add Plot background.
// Batik doesn't seem to support any styling (via 'style' element or 'style' attribute)
// of root
© 2015 - 2025 Weber Informatics LLC | Privacy Policy