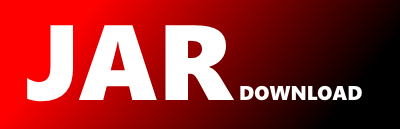
commonMain.jetbrains.datalore.plot.builder.layout.AxisLayoutInfo.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2020. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package jetbrains.datalore.plot.builder.layout
import jetbrains.datalore.base.gcommon.collect.ClosedRange
import jetbrains.datalore.base.geometry.DoubleRectangle
import jetbrains.datalore.base.geometry.DoubleVector
import jetbrains.datalore.plot.base.render.svg.TextLabel
import jetbrains.datalore.plot.base.scale.ScaleBreaks
class AxisLayoutInfo private constructor(b: Builder) {
val axisBreaks: ScaleBreaks?
val axisLength: Double
val orientation: jetbrains.datalore.plot.builder.guide.Orientation?
val axisDomain: ClosedRange?
val tickLabelsBounds: DoubleRectangle?
val tickLabelRotationAngle: Double
val tickLabelHorizontalAnchor: TextLabel.HorizontalAnchor? // optional
val tickLabelVerticalAnchor: TextLabel.VerticalAnchor? // optional
val tickLabelAdditionalOffsets: List? // optional
val tickLabelSmallFont: Boolean
private val tickLabelsBoundsMax: DoubleRectangle? // debug
init {
require(b.myAxisBreaks != null)
require(b.myOrientation != null)
require(b.myTickLabelsBounds != null)
require(b.myAxisDomain != null)
this.axisBreaks = b.myAxisBreaks
this.axisLength = b.myAxisLength
this.orientation = b.myOrientation
this.axisDomain = b.myAxisDomain
this.tickLabelsBounds = b.myTickLabelsBounds
this.tickLabelRotationAngle = b.myTickLabelRotationAngle
this.tickLabelHorizontalAnchor = b.myLabelHorizontalAnchor
this.tickLabelVerticalAnchor = b.myLabelVerticalAnchor
this.tickLabelAdditionalOffsets = b.myLabelAdditionalOffsets
this.tickLabelSmallFont = b.myTickLabelSmallFont
this.tickLabelsBoundsMax = b.myMaxTickLabelsBounds
}
fun withAxisLength(axisLength: Double): Builder {
//check(axisDomain != null)
val b = Builder()
b.myAxisBreaks = axisBreaks
b.myAxisLength = axisLength
b.myOrientation = this.orientation
b.myAxisDomain = this.axisDomain
b.myTickLabelsBounds = this.tickLabelsBounds
b.myTickLabelRotationAngle = this.tickLabelRotationAngle
b.myLabelHorizontalAnchor = this.tickLabelHorizontalAnchor
b.myLabelVerticalAnchor = this.tickLabelVerticalAnchor
b.myLabelAdditionalOffsets = this.tickLabelAdditionalOffsets
b.myTickLabelSmallFont = this.tickLabelSmallFont
b.myMaxTickLabelsBounds = this.tickLabelsBoundsMax
return b
}
fun axisBounds(): DoubleRectangle {
return tickLabelsBounds!!.union(DoubleRectangle(0.0, 0.0, 0.0, 0.0))
}
class Builder {
var myAxisLength: Double = 0.toDouble()
var myOrientation: jetbrains.datalore.plot.builder.guide.Orientation? = null
var myAxisDomain: ClosedRange? = null
var myMaxTickLabelsBounds: DoubleRectangle? = null
var myTickLabelSmallFont = false
var myLabelAdditionalOffsets: List? = null
var myLabelHorizontalAnchor: TextLabel.HorizontalAnchor? = null
var myLabelVerticalAnchor: TextLabel.VerticalAnchor? = null
var myTickLabelRotationAngle = 0.0
var myTickLabelsBounds: DoubleRectangle? = null
var myAxisBreaks: ScaleBreaks? = null
fun build(): AxisLayoutInfo {
return AxisLayoutInfo(this)
}
fun axisLength(d: Double): Builder {
myAxisLength = d
return this
}
fun orientation(o: jetbrains.datalore.plot.builder.guide.Orientation): Builder {
myOrientation = o
return this
}
fun axisDomain(r: ClosedRange): Builder {
myAxisDomain = r
return this
}
fun tickLabelsBoundsMax(r: DoubleRectangle?): Builder {
myMaxTickLabelsBounds = r
return this
}
fun tickLabelSmallFont(b: Boolean): Builder {
myTickLabelSmallFont = b
return this
}
fun tickLabelAdditionalOffsets(labelAdditionalOffsets: List?): Builder {
myLabelAdditionalOffsets = labelAdditionalOffsets
return this
}
fun tickLabelHorizontalAnchor(labelHorizontalAnchor: TextLabel.HorizontalAnchor?): Builder {
myLabelHorizontalAnchor = labelHorizontalAnchor
return this
}
fun tickLabelVerticalAnchor(labelVerticalAnchor: TextLabel.VerticalAnchor?): Builder {
myLabelVerticalAnchor = labelVerticalAnchor
return this
}
fun tickLabelRotationAngle(rotationAngle: Double): Builder {
myTickLabelRotationAngle = rotationAngle
return this
}
fun tickLabelsBounds(rectangle: DoubleRectangle?): Builder {
myTickLabelsBounds = rectangle
return this
}
fun axisBreaks(breaks: ScaleBreaks?): Builder {
myAxisBreaks = breaks
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy