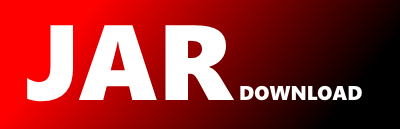
commonMain.jetbrains.datalore.plot.common.geometry.PolylineSimplifier.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2019. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package jetbrains.datalore.plot.common.geometry
import jetbrains.datalore.base.geometry.DoubleVector
class PolylineSimplifier private constructor(private val myPoints: List, strategy: RankingStrategy) {
private val myWeights: List
private var myWeightLimit = Double.NaN
private var myCountLimit = -1
val points: List
get() =
indices.map { myPoints[it] }
val indices: List
get() {
val sorted = (0 until myPoints.size)
.map { i -> Pair(i, myWeights[i]) }
.filter { p -> !getWeight(p).isNaN() }
.sortedWith(compareBy> { this.getWeight(it) }.reversed())
val filtered: Collection>
if (isWeightLimitSet) {
filtered = sorted.filter { p -> getWeight(p) > myWeightLimit }
} else {
filtered = sorted.take(myCountLimit)
}
return filtered
.map { this.getIndex(it) }
.sorted()
}
private val isWeightLimitSet: Boolean
get() = !myWeightLimit.isNaN()
init {
myWeights = strategy.getWeights(myPoints)
}
fun setWeightLimit(weightLimit: Double): PolylineSimplifier {
myWeightLimit = weightLimit
myCountLimit = -1
return this
}
fun setCountLimit(countLimit: Int): PolylineSimplifier {
myWeightLimit = Double.NaN
myCountLimit = countLimit
return this
}
private fun getWeight(p: Pair): Double {
return p.second
}
private fun getIndex(p: Pair): Int {
return p.first
}
interface RankingStrategy {
fun getWeights(points: List): List
}
companion object {
fun visvalingamWhyatt(points: List): PolylineSimplifier {
return PolylineSimplifier(
points,
VisvalingamWhyattSimplification()
)
}
fun douglasPeucker(points: List): PolylineSimplifier {
return PolylineSimplifier(
points,
DouglasPeuckerSimplification()
)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy