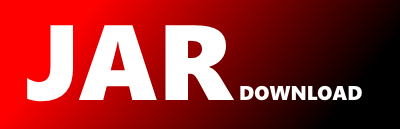
jvmMain.base.event.testCase.EventMatchers.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2019. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package jetbrains.datalore.base.event.testCase
import jetbrains.datalore.base.observable.event.EventHandler
import jetbrains.datalore.base.observable.event.EventSource
import jetbrains.datalore.base.observable.property.PropertyChangeEvent
import org.hamcrest.CoreMatchers
import org.hamcrest.Description
import org.hamcrest.Matcher
import org.hamcrest.TypeSafeDiagnosingMatcher
object EventMatchers {
fun setTestHandler(source: EventSource): MatchingHandler {
val handler = MatchingHandler()
source.addHandler(handler)
return handler
}
fun noEvents(): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: MatchingHandler, mismatchDescription: Description): Boolean {
if (item.events.isEmpty()) {
return true
} else {
mismatchDescription.appendText("events happened: " + item.events)
return false
}
}
override fun describeTo(description: Description) {
description.appendText("no events")
}
}
}
fun anyEvents(): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: MatchingHandler, mismatchDescription: Description): Boolean {
if (item.events.isEmpty()) {
mismatchDescription.appendText("no events happened")
return false
} else {
return true
}
}
override fun describeTo(description: Description) {
description.appendText("any events")
}
}
}
fun singleEvent(valueMatcher: Matcher): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: MatchingHandler, mismatchDescription: Description): Boolean {
if (item.events.isEmpty()) {
mismatchDescription.appendText("no events happened")
return false
} else if (item.events.size == 1) {
val value = item.events[0]
if (valueMatcher.matches(value)) {
return true
} else {
mismatchDescription.appendText("value ")
valueMatcher.describeMismatch(value, mismatchDescription)
return false
}
} else {
mismatchDescription.appendText("few events happened: " + item.events)
return false
}
}
override fun describeTo(description: Description) {
description.appendText("only event ").appendDescriptionOf(valueMatcher)
}
}
}
fun lastEvent(valueMatcher: Matcher): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: MatchingHandler, mismatchDescription: Description): Boolean {
if (item.events.isEmpty()) {
mismatchDescription.appendText("no events happened")
return false
} else {
val value = item.events[item.events.size - 1]
if (valueMatcher.matches(value)) {
return true
} else {
mismatchDescription.appendText("last value ")
valueMatcher.describeMismatch(value, mismatchDescription)
return false
}
}
}
override fun describeTo(description: Description) {
description.appendText("last event ").appendDescriptionOf(valueMatcher)
}
}
}
fun allEvents(valuesMatcher: Matcher>): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: MatchingHandler, mismatchDescription: Description): Boolean {
if (valuesMatcher.matches(item.events)) {
return true
} else {
mismatchDescription.appendText("handled events ")
valuesMatcher.describeMismatch(item.events, mismatchDescription)
return false
}
}
override fun describeTo(description: Description) {
description.appendText("events ").appendDescriptionOf(valuesMatcher)
}
}
}
fun newValue(valueMatcher: Matcher): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: PropertyChangeEvent, mismatchDescription: Description): Boolean {
if (valueMatcher.matches(item.newValue)) {
return true
} else {
mismatchDescription.appendText("new value ")
valueMatcher.describeMismatch(item.newValue, mismatchDescription)
return false
}
}
override fun describeTo(description: Description) {
description.appendText("new value ").appendDescriptionOf(valueMatcher)
}
}
}
fun newValueIs(value: ValueT): Matcher> {
return newValue(CoreMatchers.`is`(value))
}
fun oldValue(valueMatcher: Matcher): Matcher> {
return object : TypeSafeDiagnosingMatcher>() {
override fun matchesSafely(item: PropertyChangeEvent, mismatchDescription: Description): Boolean {
if (valueMatcher.matches(item.oldValue)) {
return true
} else {
mismatchDescription.appendText("old value ")
valueMatcher.describeMismatch(item.oldValue, mismatchDescription)
return false
}
}
override fun describeTo(description: Description) {
description.appendText("old value ").appendDescriptionOf(valueMatcher)
}
}
}
fun oldValueIs(value: ValueT): Matcher> {
return oldValue(CoreMatchers.`is`(value))
}
class MatchingHandler : EventHandler {
internal val events = ArrayList()
override fun onEvent(event: EventT) {
events.add(event)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy