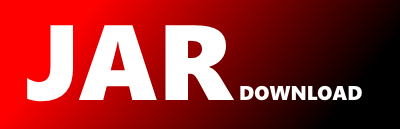
commonMain.jetbrains.datalore.base.function.Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lets-plot-common Show documentation
Show all versions of lets-plot-common Show documentation
Lets-Plot JVM package without rendering part
/*
* Copyright (c) 2019. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package jetbrains.datalore.base.function
object Functions {
private val TRUE_PREDICATE: Predicate = {
true
}
private val FALSE_PREDICATE: Predicate = {
false
}
private val NULL_PREDICATE: Predicate = {
it == null
}
private val NOT_NULL_PREDICATE: Predicate = {
it != null
}
fun constantSupplier(value: ItemT): Supplier {
return object : Supplier {
override fun get(): ItemT {
return value
}
}
}
fun memorize(supplier: Supplier): Supplier {
return Memo(supplier)
}
fun alwaysTrue(): Predicate {
return TRUE_PREDICATE
}
fun alwaysFalse(): Predicate {
return FALSE_PREDICATE
}
fun constant(result: ResultT): (ArgT) -> ResultT {
return {
result
}
}
fun isNull(): Predicate {
return NULL_PREDICATE
}
fun isNotNull(): Predicate {
return NOT_NULL_PREDICATE
}
fun identity(): (ValueT) -> ValueT {
return { it }
}
fun same(value: Any?): Predicate {
return {
it === value
}
}
fun funcOf(lambda: (ArgT) -> ResultT): Function {
return object : Function {
override fun apply(value: ArgT): ResultT {
return lambda(value)
}
}
}
private class Memo internal constructor(private val mySupplier: Supplier) : Supplier {
private var myCachedValue: ItemT? = null
private var myCached = false
override fun get(): ItemT {
if (!myCached) {
myCachedValue = mySupplier.get()
myCached = true
}
return myCachedValue!!
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy