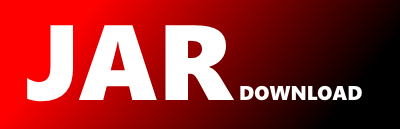
commonMain.org.jetbrains.letsPlot.geom.geom_area.kt Maven / Gradle / Ivy
/*
* Copyright (c) 2021. JetBrains s.r.o.
* Use of this source code is governed by the MIT license that can be found in the LICENSE file.
*/
package org.jetbrains.letsPlot.geom
import org.jetbrains.letsPlot.Geom.area
import org.jetbrains.letsPlot.Stat.identity
import org.jetbrains.letsPlot.core.spec.Option
import org.jetbrains.letsPlot.intern.Layer
import org.jetbrains.letsPlot.intern.Options
import org.jetbrains.letsPlot.intern.layer.PosOptions
import org.jetbrains.letsPlot.intern.layer.SamplingOptions
import org.jetbrains.letsPlot.intern.layer.StatOptions
import org.jetbrains.letsPlot.intern.layer.WithColorOption
import org.jetbrains.letsPlot.intern.layer.WithFillOption
import org.jetbrains.letsPlot.intern.layer.geom.AreaAesthetics
import org.jetbrains.letsPlot.intern.layer.geom.AreaMapping
import org.jetbrains.letsPlot.pos.positionStack
import org.jetbrains.letsPlot.tooltips.TooltipOptions
@Suppress("ClassName")
/**
* Displays the development of quantitative values over an interval. This is the continuous analog of `geomBar()`.
*
* ## Examples
*
* - [marginal_layers.ipynb](https://nbviewer.org/github/JetBrains/lets-plot-docs/blob/master/source/kotlin_examples/cookbook/marginal_layers.ipynb)
*
* - [tooltip_config.ipynb](https://nbviewer.org/github/JetBrains/lets-plot-docs/blob/master/source/kotlin_examples/cookbook/tooltip_config.ipynb)
*
* @param data The data to be displayed in this layer. If null, the default, the data
* is inherited from the plot data as specified in the call to [letsPlot][org.jetbrains.letsPlot.letsPlot].
* @param stat The statistical transformation to use on the data for this layer.
* Supported transformations: `Stat.identity`, `Stat.bin()`, `Stat.count()`, etc. see [Stat][org.jetbrains.letsPlot.Stat].
* @param position default = `positionStack()`. Position adjustment: `positionIdentity`,
* `positionStack()`, `positionDodge()`, etc. see [Position](https://lets-plot.org/kotlin/-lets--plot--kotlin/org.jetbrains.letsPlot.pos/).
* @param showLegend default = true.
* false - do not show legend for this layer.
* @param manualKey String or result of the call to the `layerKey()` function.
* The key to show in the manual legend. Specifies the text for the legend label or advanced settings using the `layerKey()` function.
* @param sampling Result of the call to the `samplingXxx()` function.
* To prevent any sampling for this layer pass value `samplingNone`.
* For more info see [sampling.html](https://lets-plot.org/kotlin/sampling.html).
* @param tooltips Result of the call to the `layerTooltips()` function.
* Specifies appearance, style and content.
* @param flat default = false.
* true - keeps a line straight (corresponding to a loxodrome in case of Mercator projection).
* false - allows a line to be reprojected, so it can become a curve.
* @param x X-axis coordinates.
* @param y Y-axis coordinates.
* @param alpha Transparency level of a layer.
* Understands numbers between 0 and 1.
* @param color Color of the geometry.
* For more info see: [aesthetics.html#color-and-fill](https://lets-plot.org/kotlin/aesthetics.html#color-and-fill).
* @param fill Fill color.
* For more info see: [aesthetics.html#color-and-fill](https://lets-plot.org/kotlin/aesthetics.html#color-and-fill).
* @param linetype Type of the line of border.
* Codes and names: 0 = "blank", 1 = "solid", 2 = "dashed", 3 = "dotted", 4 = "dotdash",
* 5 = "longdash", 6 = "twodash".
* For more info see: [aesthetics.html#line-types](https://lets-plot.org/kotlin/aesthetics.html#line-types).
* @param size Line width.
* @param colorBy default = "color" ("fill", "color", "paint_a", "paint_b", "paint_c").
* Defines the color aesthetic for the geometry.
* @param fillBy default = "fill" ("fill", "color", "paint_a", "paint_b", "paint_c").
* Defines the fill aesthetic for the geometry.
* @param mapping Set of aesthetic mappings.
* Aesthetic mappings describe the way that variables in the data are
* mapped to plot "aesthetics".
*/
class geomArea(
data: Map<*, *>? = null,
stat: StatOptions = identity,
position: PosOptions = positionStack(),
showLegend: Boolean = true,
manualKey: Any? = null,
sampling: SamplingOptions? = null,
tooltips: TooltipOptions? = null,
private val flat: Boolean? = null,
override val x: Number? = null,
override val y: Number? = null,
override val alpha: Number? = null,
override val color: Any? = null,
override val fill: Any? = null,
override val linetype: Any? = null,
override val size: Number? = null,
override val colorBy: String? = null,
override val fillBy: String? = null,
mapping: AreaMapping.() -> Unit = {}
) : AreaAesthetics,
WithColorOption,
WithFillOption,
Layer(
mapping = AreaMapping().apply(mapping).seal(),
data = data,
geom = area(),
stat = stat,
position = position,
showLegend = showLegend,
manualKey = manualKey,
sampling = sampling,
tooltips = tooltips
) {
override fun seal() = super.seal() +
super.seal() +
super.seal() +
Options.of(
Option.Geom.Area.FLAT to flat
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy