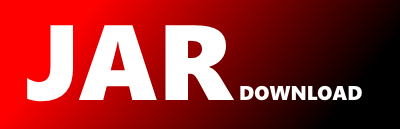
org.jfleet.csv.CsvConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfleet Show documentation
Show all versions of jfleet Show documentation
JFleet loads data mapped as JPA into DB with alternate and fast way from JPA
/**
* Copyright 2017 Jerónimo López Bezanilla
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jfleet.csv;
import java.nio.charset.Charset;
import org.jfleet.EntityInfo;
import org.jfleet.common.TypeSerializer;
public class CsvConfiguration {
private Class clazz;
private EntityInfo entityInfo;
private Charset charset = Charset.forName("UTF-8");
private TypeSerializer typeSerializer = new CsvTypeSerializer();
private boolean header = true;
private char fieldSeparator = ',';
private char textDelimiter = '"';
private boolean alwaysDelimitText = false;
private String lineDelimiter = System.lineSeparator();
public CsvConfiguration(Class clazz) {
this.clazz = clazz;
}
@SuppressWarnings("unchecked")
public CsvConfiguration(EntityInfo entityInfo) {
this.entityInfo = entityInfo;
this.clazz = (Class) entityInfo.getEntityClass();
}
public Class getClazz() {
return clazz;
}
public EntityInfo getEntityInfo() {
return entityInfo;
}
public Charset getCharset() {
return charset;
}
public TypeSerializer getTypeSerializer() {
return typeSerializer;
}
public boolean isHeader() {
return header;
}
public char getFieldSeparator() {
return fieldSeparator;
}
public char getTextDelimiter() {
return textDelimiter;
}
public boolean isAlwaysDelimitText() {
return alwaysDelimitText;
}
public String getLineDelimiter() {
return lineDelimiter;
}
public static class Builder {
private Class clazz;
private EntityInfo entityInfo;
private Charset charset = Charset.forName("UTF-8");
private TypeSerializer typeSerializer = new CsvTypeSerializer();
private boolean header = true;
private char fieldSeparator = ',';
private char textDelimiter = '"';
private boolean alwaysDelimitText = false;
private String lineDelimiter = System.lineSeparator();
public Builder(Class clazz) {
this.clazz = clazz;
}
@SuppressWarnings("unchecked")
public Builder(EntityInfo entityInfo) {
this.clazz = (Class) entityInfo.getEntityClass();
this.entityInfo = entityInfo;
}
public Builder entityInfo(EntityInfo entityInfo) {
this.entityInfo = entityInfo;
return this;
}
public Builder charset(Charset charset) {
this.charset = charset;
return this;
}
public Builder typeSerializer(TypeSerializer typeSerializer) {
this.typeSerializer = typeSerializer;
return this;
}
public Builder header(boolean header) {
this.header = header;
return this;
}
public Builder fieldSeparator(char fieldSeparator) {
this.fieldSeparator = fieldSeparator;
return this;
}
public Builder textDelimiter(char textDelimiter) {
this.textDelimiter = textDelimiter;
return this;
}
public Builder alwaysDelimitText(boolean alwaysDelimitText) {
this.alwaysDelimitText = alwaysDelimitText;
return this;
}
public Builder lineDelimiter(String lineDelimiter) {
this.lineDelimiter = lineDelimiter;
return this;
}
public CsvConfiguration build() {
CsvConfiguration config = new CsvConfiguration<>(clazz);
config.entityInfo = entityInfo;
config.charset = charset;
config.typeSerializer = typeSerializer;
config.header = header;
config.fieldSeparator = fieldSeparator;
config.textDelimiter = textDelimiter;
config.alwaysDelimitText = alwaysDelimitText;
config.lineDelimiter = lineDelimiter;
return config;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy