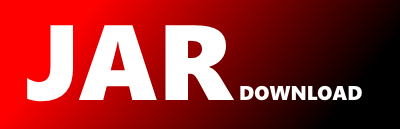
org.jfree.chart.util.PaintUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfreechart Show documentation
Show all versions of jfreechart Show documentation
JFreeChart is a class library, written in Java, for generating charts.
Utilising the Java2D API, it supports a wide range of chart types including
bar charts, pie charts, line charts, XY-plots, time series plots, Sankey charts
and more.
/* ===========================================================
* JFreeChart : a free chart library for the Java(tm) platform
* ===========================================================
*
* (C) Copyright 2000-2017, by Object Refinery Limited and Contributors.
*
* Project Info: http://www.jfree.org/jfreechart/index.html
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License, or
* (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301,
* USA.
*
* [Oracle and Java are registered trademarks of Oracle and/or its affiliates.
* Other names may be trademarks of their respective owners.]
*
*/
package org.jfree.chart.util;
import java.awt.Color;
import java.awt.GradientPaint;
import java.awt.LinearGradientPaint;
import java.awt.Paint;
import java.awt.RadialGradientPaint;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Arrays;
/**
* Utility code that relates to {@code Paint} objects.
*/
public class PaintUtils {
/**
* Private constructor prevents object creation.
*/
private PaintUtils() {
}
/**
* Returns {@code true} if the two {@code Paint} objects are equal
* OR both {@code null}. This method handles
* {@code GradientPaint}, {@code LinearGradientPaint} and
* {@code RadialGradientPaint} as a special cases, since those classes do
* not override the {@code equals()} method.
*
* @param p1 paint 1 ({@code null} permitted).
* @param p2 paint 2 ({@code null} permitted).
*
* @return A boolean.
*/
public static boolean equal(Paint p1, Paint p2) {
if (p1 == p2) {
return true;
}
// handle cases where either or both arguments are null
if (p1 == null) {
return (p2 == null);
}
if (p2 == null) {
return false;
}
// handle GradientPaint as a special case...
if (p1 instanceof GradientPaint && p2 instanceof GradientPaint) {
GradientPaint gp1 = (GradientPaint) p1;
GradientPaint gp2 = (GradientPaint) p2;
return gp1.getColor1().equals(gp2.getColor1())
&& gp1.getColor2().equals(gp2.getColor2())
&& gp1.getPoint1().equals(gp2.getPoint1())
&& gp1.getPoint2().equals(gp2.getPoint2())
&& gp1.isCyclic() == gp2.isCyclic()
&& gp1.getTransparency() == gp1.getTransparency();
} else if (p1 instanceof LinearGradientPaint
&& p2 instanceof LinearGradientPaint) {
LinearGradientPaint lgp1 = (LinearGradientPaint) p1;
LinearGradientPaint lgp2 = (LinearGradientPaint) p2;
return lgp1.getStartPoint().equals(lgp2.getStartPoint())
&& lgp1.getEndPoint().equals(lgp2.getEndPoint())
&& Arrays.equals(lgp1.getFractions(), lgp2.getFractions())
&& Arrays.equals(lgp1.getColors(), lgp2.getColors())
&& lgp1.getCycleMethod() == lgp2.getCycleMethod()
&& lgp1.getColorSpace() == lgp2.getColorSpace()
&& lgp1.getTransform().equals(lgp2.getTransform());
} else if (p1 instanceof RadialGradientPaint
&& p2 instanceof RadialGradientPaint) {
RadialGradientPaint rgp1 = (RadialGradientPaint) p1;
RadialGradientPaint rgp2 = (RadialGradientPaint) p2;
return rgp1.getCenterPoint().equals(rgp2.getCenterPoint())
&& rgp1.getRadius() == rgp2.getRadius()
&& rgp1.getFocusPoint().equals(rgp2.getFocusPoint())
&& Arrays.equals(rgp1.getFractions(), rgp2.getFractions())
&& Arrays.equals(rgp1.getColors(), rgp2.getColors())
&& rgp1.getCycleMethod() == rgp2.getCycleMethod()
&& rgp1.getColorSpace() == rgp2.getColorSpace()
&& rgp1.getTransform().equals(rgp2.getTransform());
} else {
return p1.equals(p2);
}
}
/**
* Converts a color into a string. If the color is equal to one of the
* defined constant colors, that name is returned instead. Otherwise the
* color is returned as hex-string.
*
* @param c the color.
* @return the string for this color.
*/
public static String colorToString(Color c) {
try {
Field[] fields = Color.class.getFields();
for (int i = 0; i < fields.length; i++) {
Field f = fields[i];
if (Modifier.isPublic(f.getModifiers())
&& Modifier.isFinal(f.getModifiers())
&& Modifier.isStatic(f.getModifiers())) {
final String name = f.getName();
final Object oColor = f.get(null);
if (oColor instanceof Color) {
if (c.equals(oColor)) {
return name;
}
}
}
}
} catch (Exception e) {
//
}
// no defined constant color, so this must be a user defined color
final String color = Integer.toHexString(c.getRGB() & 0x00ffffff);
final StringBuffer retval = new StringBuffer(7);
retval.append("#");
final int fillUp = 6 - color.length();
for (int i = 0; i < fillUp; i++) {
retval.append("0");
}
retval.append(color);
return retval.toString();
}
/**
* Converts a given string into a color.
*
* @param value the string, either a name or a hex-string.
* @return the color.
*/
public static Color stringToColor(String value) {
if (value == null) {
return Color.BLACK;
}
try {
// get color by hex or octal value
return Color.decode(value);
} catch (NumberFormatException nfe) {
// if we can't decode lets try to get it by name
try {
// try to get a color by name using reflection
final Field f = Color.class.getField(value);
return (Color) f.get(null);
} catch (Exception ce) {
// if we can't get any color return black
return Color.BLACK;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy