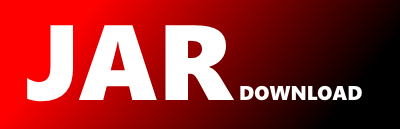
org.jfxtras.AbstractJavaFxMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javafx-maven-plugin Show documentation
Show all versions of javafx-maven-plugin Show documentation
This plugin offers a possibility to deploy the JavaFX SDK jars to your local repository.
The newest version!
/**
* Copyright (c) 2008-2010, JFXtras Group
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of JFXtras nor the names of its contributors may be used
* to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.jfxtras;
import org.apache.maven.artifact.factory.ArtifactFactory;
import org.apache.maven.artifact.installer.ArtifactInstallationException;
import org.apache.maven.artifact.installer.ArtifactInstaller;
import org.apache.maven.artifact.repository.ArtifactRepository;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.model.Model;
import org.apache.maven.model.io.xpp3.MavenXpp3Writer;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.sonatype.plexus.component.bundlepublisher.model.BundleDescriptor;
import java.io.BufferedInputStream;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.net.URL;
import java.util.Properties;
/**
*
*/
public abstract class AbstractJavaFxMojo extends AbstractMojo {
/**
* The Maven Session Object
*
* @parameter expression="${session}"
* @required
* @readonly
*/
protected MavenSession session;
/**
* Used to look up Artifacts in the remote repository.
*
* @component role="org.apache.maven.artifact.factory.ArtifactFactory"
* @required
* @readonly
*/
protected ArtifactFactory factory;
/**
* Location of the local repository.
*
* @parameter expression="${localRepository}"
* @readonly
* @required
*/
protected ArtifactRepository localRepository;
/**
* Location of the local repository.
*
* @component
*/
protected ArtifactInstaller installer;
/**
* Location of the javafx sdk home.
*
* @parameter expression="${javafx.home}"
*/
protected File javaFxHomeDir;
/**
* execute
*
* @throws org.apache.maven.plugin.MojoExecutionException
* if any.
*/
public void execute() throws MojoExecutionException {
File sdkInstallDir = getSdkDir();
if ( !sdkInstallDir.exists() ) {
throw new MojoExecutionException( "JavaFX SDK not found at <" + sdkInstallDir.getAbsolutePath() + ">" );
}
String version = getVersion( sdkInstallDir );
getLog().info( "JavaFX SDK (" + version + ") located at <" + sdkInstallDir.getAbsolutePath() + ">" );
URL mapping = getClass().getResource( version + ".xml" );
if ( mapping == null ) {
throw new MojoExecutionException( "No mapping file found for version <" + version + ">" );
}
BundleDescriptor descriptor;
try {
descriptor = BundleDescriptor.read( mapping.openStream() );
} catch ( Exception e ) {
throw new MojoExecutionException( "Cannot read mapping file", e );
}
try {
execute( sdkInstallDir, descriptor, version );
} catch ( MojoExecutionException e ) {
throw e;
} catch ( Exception e ) {
throw new MojoExecutionException( "Could not install artifact", e );
}
}
protected abstract void execute( File sdkInstallDir, BundleDescriptor descriptor, String version ) throws MojoExecutionException, IOException, ArtifactInstallationException, Exception;
protected String getVersion( File sdkInstallDir ) throws MojoExecutionException {
File invoice = new File( sdkInstallDir, "invoice.properties" );
try {
Properties properties = new Properties();
BufferedInputStream in = new BufferedInputStream( new FileInputStream( invoice ) );
try {
properties.load( in );
return properties.getProperty( "jfx.release.version" );
} finally {
in.close();
}
} catch ( IOException e ) {
throw new MojoExecutionException( "Could not parse version from <" + invoice.getAbsolutePath() + ">" );
}
}
protected File getSdkDir() throws MojoExecutionException {
getLog().info( "Search for JavaFX SDK installation dir" );
if ( javaFxHomeDir != null ) {
if ( !javaFxHomeDir.isDirectory() ) {
throw new MojoExecutionException( "Invalid {javafx.home} property. Nothing found at <" + javaFxHomeDir.getAbsolutePath() + ">" );
}
getLog().info( "Found SDK using ${javafx.home} at " + javaFxHomeDir.getAbsolutePath() );
return javaFxHomeDir;
}
try {
return getDirBySystemProperty( "JAVAFX_HOME" );
} catch ( IllegalArgumentException ignore ) {
}
try {
return getDirBySystemProperty( "FX_HOME" );
} catch ( IllegalArgumentException ignore ) {
}
//Try the default locations
try { //Windows
return verifyGuessedDir( "C:\\Program Files\\JavaFX\\javafx-sdk1.3" );
} catch ( IllegalArgumentException ignore ) {
}
try { //Mac
return verifyGuessedDir( "/Library/JavaFX/Home" );
} catch ( IllegalArgumentException ignore ) {
}
try { //Linux
return verifyGuessedDir( "/opt/javafx-sdk1.3" );
} catch ( IllegalArgumentException ignore ) {
}
throw new MojoExecutionException( "No JavaFX SDK found" );
}
private File verifyGuessedDir( String guessedPath ) throws IllegalArgumentException {
File guessedDir = new File( guessedPath );
if ( !guessedDir.isDirectory() ) {
throw new IllegalArgumentException( "Is not a directory" );
}
getLog().info( "Found SDK at default location: " + guessedDir.getAbsolutePath() );
return guessedDir;
}
private File getDirBySystemProperty( String property ) throws IllegalArgumentException {
String fxHome = session.getExecutionProperties().getProperty( property );
if ( fxHome == null ) {
throw new IllegalArgumentException( "No property found for <" + property + ">" );
}
getLog().info( "Found SDK using ${" + property + "} at " + fxHome );
return new File( fxHome );
}
protected File createTemporaryPom( Model pom ) throws IOException {
File file = File.createTempFile( "pom", ".xml" );
return writeModelToFile( pom, file );
}
protected File writeModelToFile( Model pom, File file ) throws IOException {
Writer out = new BufferedWriter( new FileWriter( file ) );
try {
new MavenXpp3Writer().write( out, pom );
} finally {
out.close();
}
return file;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy