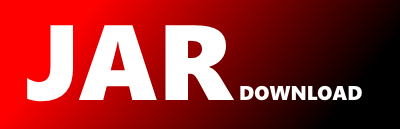
jfxtras.scene.layout.responsivepane.Layout Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2011-2021, JFXtras
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* Neither the name of the organization nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL JFXTRAS BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package jfxtras.scene.layout.responsivepane;
import javafx.beans.DefaultProperty;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.scene.Node;
/**
*
*/
@DefaultProperty("root")
public class Layout {
/**
* For FXML
*/
public Layout() {
}
/**
*
*/
public Layout(Size sizeAtLeast, Node root) {
setSizeAtLeast(sizeAtLeast);
setRoot(root);
}
/**
*
*/
public Layout(Size sizeAtLeast, Orientation orientation, Node root) {
setSizeAtLeast(sizeAtLeast);
setOrientation(orientation);
setRoot(root);
}
/** Root */
public ObjectProperty rootProperty() { return rootProperty; }
final private SimpleObjectProperty rootProperty = new SimpleObjectProperty<>(this, "root", null);
public Node getRoot() { return rootProperty.getValue(); }
public void setRoot(Node value) { rootProperty.setValue(value); }
public Layout withRoot(Node value) { setRoot(value); return this; }
/** sizeAtLeast */
public ObjectProperty sizeAtLeastProperty() { return sizeAtLeastProperty; }
final private SimpleObjectProperty sizeAtLeastProperty = new SimpleObjectProperty<>(this, "sizeAtLeast", Size.ZERO);
public Size getSizeAtLeast() { return sizeAtLeastProperty.getValue(); }
public void setSizeAtLeast(Size value) { sizeAtLeastProperty.setValue(value); }
public Layout withSizeAtLeast(Size value) { setSizeAtLeast(value); return this; }
/** Orientation */
public ObjectProperty orientationProperty() { return orientationProperty; }
final private SimpleObjectProperty orientationProperty = new SimpleObjectProperty<>(this, "orientation", null);
public Orientation getOrientation() { return orientationProperty.getValue(); }
public void setOrientation(Orientation value) { orientationProperty.setValue(value); }
public Layout withOrientation(Orientation value) { setOrientation(value); return this; }
public String describeSizeConstraints() {
return getSizeAtLeast() + (getOrientation() == null ? "" : "-" + getOrientation());
}
public String toString() {
return super.toString()
+ (getRoot() == null || getRoot().getId() == null ? "" : ", root-id=" + getRoot().getId())
+ (getRoot() == null? "" : ", root=" + getRoot())
;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy