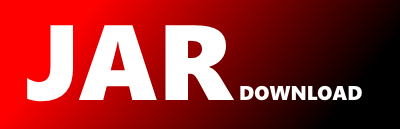
jfxtras.labs.util.NodeUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfxtras-labs Show documentation
Show all versions of jfxtras-labs Show documentation
Experimental components for JavaFX 2
/**
* NodeUtil.java
*
* Copyright (c) 2011-2015, JFXtras
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the organization nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package jfxtras.labs.util;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import javafx.scene.Group;
import javafx.scene.Node;
import javafx.scene.Parent;
import javafx.scene.layout.Pane;
/**
* Utility class that provides methods to simplify node handling. Possible use
* cases are searching for nodes at specific locations, adding/removing nodes
* to/from parents (Parent interface does not give write access to children).
*
* @author Michael Hoffer <[email protected]>
*/
public class NodeUtil {
// no instanciation allowed
private NodeUtil() {
throw new AssertionError(); // not in this class either!
}
/**
* Removes the specified node from its parent.
*
* @param n the node to remove
*
* @throws IllegalArgumentException if an unsupported parent class has been
* specified or the parent is null
*/
public static void removeFromParent(Node n) {
if (n.getParent() instanceof Group) {
((Group) n.getParent()).getChildren().remove(n);
} else if (n.getParent() instanceof Pane) {
((Pane) n.getParent()).getChildren().remove(n);
} else if (n.getParent() != null) { // we ignore if node has no parent
throw new IllegalArgumentException("Unsupported parent: " + n.getParent());
}
}
/**
* Adds the given node to the specified parent.
*
* @param p parent
* @param n node
*
* @throws IllegalArgumentException if an unsupported parent class has been
* specified or the parent is null
*/
public static void addToParent(Parent p, Node n) {
if (p instanceof Group) {
((Group) p).getChildren().add(n);
} else if (p instanceof Pane) {
((Pane) p).getChildren().add(n);
} else {
throw new IllegalArgumentException("Unsupported parent: " + p);
}
}
/**
* Returns the first node at the given location that is an instance of the
* specified class object. The search is performed recursively until either
* a node has been found or a leaf node is reached.
*
* @param p parent node
* @param sceneX x coordinate
* @param sceneY y coordinate
* @param nodeClass node class to search for
* @return a node that contains the specified screen coordinates and is an
* instance of the specified class or null
if no such node
* exist
*/
public static Node getNode(Parent p, double sceneX, double sceneY, Class> nodeClass) {
// dammit! javafx uses "wrong" children order.
List rightOrder = new ArrayList<>();
rightOrder.addAll(p.getChildrenUnmodifiable());
Collections.reverse(rightOrder);
for (Node n : rightOrder) {
boolean contains = n.contains(n.sceneToLocal(sceneX, sceneY));
if (contains) {
if (nodeClass.isAssignableFrom(n.getClass())) {
return n;
}
if (n instanceof Parent) {
return getNode((Parent) n, sceneX, sceneY, nodeClass);
}
}
}
return null;
}
public static List nodesWithParent(Parent p, List nodes) {
List result = new ArrayList<>();
for (Node n : nodes) {
if (p.equals(n.getParent())) {
result.add(n);
}
}
return result;
}
public static List nodesThatImplement(List nodes, Class> cls) {
List result = new ArrayList<>();
for (Node n : nodes) {
if (cls.isAssignableFrom(n.getClass())) {
result.add(n);
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy