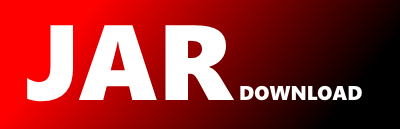
org.jfxvnc.net.rfb.render.DefaultProtocolConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfxvnc-net Show documentation
Show all versions of jfxvnc-net Show documentation
RFB/VNC protocol codec and handler based on netty
package org.jfxvnc.net.rfb.render;
import org.jfxvnc.net.rfb.codec.PixelFormat;
import org.jfxvnc.net.rfb.codec.ProtocolVersion;
import org.jfxvnc.net.rfb.codec.security.SecurityType;
/*
* #%L
* jfxvnc-net
* %%
* Copyright (C) 2015 comtel2000
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import javafx.beans.property.BooleanProperty;
import javafx.beans.property.IntegerProperty;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.SimpleBooleanProperty;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
public class DefaultProtocolConfiguration implements ProtocolConfiguration {
private final ObjectProperty versionProperty = new SimpleObjectProperty<>(ProtocolVersion.RFB_3_8);
private final ObjectProperty clientPixelFormatProperty = new SimpleObjectProperty<>(PixelFormat.RGB_888);
private final ObjectProperty securityProperty = new SimpleObjectProperty<>(SecurityType.VNC_Auth);
private final StringProperty hostProperty = new SimpleStringProperty("127.0.0.1");
private final IntegerProperty portProperty = new SimpleIntegerProperty(5900);
private final StringProperty passwordProperty = new SimpleStringProperty();
private final BooleanProperty sharedProperty = new SimpleBooleanProperty(true);
private final BooleanProperty sslProperty = new SimpleBooleanProperty(false);
private final BooleanProperty rawEncProperty = new SimpleBooleanProperty(true);
private final BooleanProperty copyRectEncProperty = new SimpleBooleanProperty(true);
private final BooleanProperty hextileEncProperty = new SimpleBooleanProperty(false);
private final BooleanProperty zlibEncProperty = new SimpleBooleanProperty(false);
private final BooleanProperty clientCursorProperty = new SimpleBooleanProperty(false);
private final BooleanProperty desktopSizeProperty = new SimpleBooleanProperty(true);
@Override
public StringProperty hostProperty() {
return hostProperty;
}
@Override
public IntegerProperty portProperty() {
return portProperty;
}
@Override
public StringProperty passwordProperty() {
return passwordProperty;
}
@Override
public BooleanProperty sslProperty() {
return sslProperty;
}
@Override
public ObjectProperty securityProperty() {
return securityProperty;
}
@Override
public BooleanProperty sharedProperty() {
return sharedProperty;
}
@Override
public ObjectProperty versionProperty() {
return versionProperty;
}
@Override
public ObjectProperty clientPixelFormatProperty() {
return clientPixelFormatProperty;
}
@Override
public BooleanProperty rawEncProperty() {
return rawEncProperty;
}
@Override
public BooleanProperty copyRectEncProperty() {
return copyRectEncProperty;
}
@Override
public BooleanProperty hextileEncProperty() {
return hextileEncProperty;
}
@Override
public BooleanProperty clientCursorProperty() {
return clientCursorProperty;
}
@Override
public BooleanProperty desktopSizeProperty() {
return desktopSizeProperty;
}
@Override
public BooleanProperty zlibEncProperty() {
return zlibEncProperty;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy