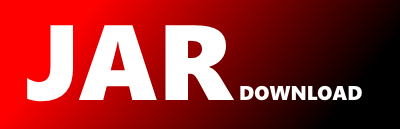
org.jgrapht.demo.HelloJGraphT Maven / Gradle / Ivy
/* ==========================================
* JGraphT : a free Java graph-theory library
* ==========================================
*
* Project Info: http://jgrapht.sourceforge.net/
* Project Creator: Barak Naveh (http://sourceforge.net/users/barak_naveh)
*
* (C) Copyright 2003-2008, by Barak Naveh and Contributors.
*
* This program and the accompanying materials are dual-licensed under
* either
*
* (a) the terms of the GNU Lesser General Public License version 2.1
* as published by the Free Software Foundation, or (at your option) any
* later version.
*
* or (per the licensee's choosing)
*
* (b) the terms of the Eclipse Public License v1.0 as published by
* the Eclipse Foundation.
*/
/* -----------------
* HelloJGraphT.java
* -----------------
* (C) Copyright 2003-2008, by Barak Naveh and Contributors.
*
* Original Author: Barak Naveh
* Contributor(s): -
*
* $Id$
*
* Changes
* -------
* 27-Jul-2003 : Initial revision (BN);
*
*/
package org.jgrapht.demo;
import java.net.*;
import org.jgrapht.*;
import org.jgrapht.graph.*;
/**
* A simple introduction to using JGraphT.
*
* @author Barak Naveh
* @since Jul 27, 2003
*/
public final class HelloJGraphT
{
private HelloJGraphT()
{
} // ensure non-instantiability.
/**
* The starting point for the demo.
*
* @param args ignored.
*/
public static void main(String [] args)
{
UndirectedGraph stringGraph = createStringGraph();
// note undirected edges are printed as: {,}
System.out.println(stringGraph.toString());
// create a graph based on URL objects
DirectedGraph hrefGraph = createHrefGraph();
// note directed edges are printed as: (,)
System.out.println(hrefGraph.toString());
}
/**
* Creates a toy directed graph based on URL objects that represents link
* structure.
*
* @return a graph based on URL objects.
*/
private static DirectedGraph createHrefGraph()
{
DirectedGraph g =
new DefaultDirectedGraph(DefaultEdge.class);
try {
URL amazon = new URL("http://www.amazon.com");
URL yahoo = new URL("http://www.yahoo.com");
URL ebay = new URL("http://www.ebay.com");
// add the vertices
g.addVertex(amazon);
g.addVertex(yahoo);
g.addVertex(ebay);
// add edges to create linking structure
g.addEdge(yahoo, amazon);
g.addEdge(yahoo, ebay);
} catch (MalformedURLException e) {
e.printStackTrace();
}
return g;
}
/**
* Create a toy graph based on String objects.
*
* @return a graph based on String objects.
*/
private static UndirectedGraph createStringGraph()
{
UndirectedGraph g =
new SimpleGraph(DefaultEdge.class);
String v1 = "v1";
String v2 = "v2";
String v3 = "v3";
String v4 = "v4";
// add the vertices
g.addVertex(v1);
g.addVertex(v2);
g.addVertex(v3);
g.addVertex(v4);
// add edges to create a circuit
g.addEdge(v1, v2);
g.addEdge(v2, v3);
g.addEdge(v3, v4);
g.addEdge(v4, v1);
return g;
}
}
// End HelloJGraphT.java
© 2015 - 2025 Weber Informatics LLC | Privacy Policy