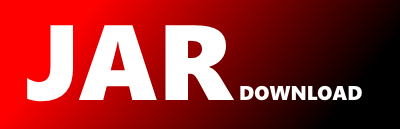
org.jgrapht.nio.EventDrivenImporter Maven / Gradle / Ivy
The newest version!
/*
* (C) Copyright 2019-2023, by Dimitrios Michail and Contributors.
*
* JGraphT : a free Java graph-theory library
*
* See the CONTRIBUTORS.md file distributed with this work for additional
* information regarding copyright ownership.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the
* GNU Lesser General Public License v2.1 or later
* which is available at
* http://www.gnu.org/licenses/old-licenses/lgpl-2.1-standalone.html.
*
* SPDX-License-Identifier: EPL-2.0 OR LGPL-2.1-or-later
*/
package org.jgrapht.nio;
import org.jgrapht.alg.util.*;
import java.io.*;
import java.nio.charset.*;
import java.util.Map;
import java.util.function.*;
/**
* Interface for an importer using consumers.
*
* @param the graph vertex type
* @param the graph edge type
*/
public interface EventDrivenImporter
{
/**
* Add an ImportEvent consumer.
*
* @param consumer the consumer
*/
void addImportEventConsumer(Consumer consumer);
/**
* Remove an ImportEvent consumer.
*
* @param consumer the consumer
*/
void removeImportEventConsumer(Consumer consumer);
/**
* Add a vertex count consumer.
*
* @param consumer the consumer
*/
void addVertexCountConsumer(Consumer consumer);
/**
* Remove a vertex count consumer.
*
* @param consumer the consumer
*/
void removeVertexCountConsumer(Consumer consumer);
/**
* Add an edge count consumer.
*
* @param consumer the consumer
*/
void addEdgeCountConsumer(Consumer consumer);
/**
* Remove an edge count consumer.
*
* @param consumer the consumer
*/
void removeEdgeCountConsumer(Consumer consumer);
/**
* Add a vertex consumer.
*
* @param consumer the consumer
*/
void addVertexConsumer(Consumer consumer);
/**
* Remove a vertex consumer.
*
* @param consumer the consumer
*/
void removeVertexConsumer(Consumer consumer);
/**
* Add a vertex with attributes consumer.
*
* @param consumer the consumer
*/
void addVertexWithAttributesConsumer(BiConsumer> consumer);
/**
* Remove a vertex with attributes consumer
*
* @param consumer the consumer
*/
void removeVertexWithAttributesConsumer(BiConsumer> consumer);
/**
* Add an edge consumer.
*
* @param consumer the consumer
*/
void addEdgeConsumer(Consumer consumer);
/**
* Remove an edge consumer.
*
* @param consumer the consumer
*/
void removeEdgeConsumer(Consumer consumer);
/**
* Add an edge with attributes consumer.
*
* @param consumer the consumer
*/
void addEdgeWithAttributesConsumer(BiConsumer> consumer);
/**
* Remove an edge with attributes consumer
*
* @param consumer the consumer
*/
void removeEdgeWithAttributesConsumer(BiConsumer> consumer);
/**
* Add a graph attribute consumer.
*
* @param consumer the consumer
*/
void addGraphAttributeConsumer(BiConsumer consumer);
/**
* Remove a graph attribute consumer.
*
* @param consumer the consumer
*/
void removeGraphAttributeConsumer(BiConsumer consumer);
/**
* Add a vertex attribute consumer.
*
* @param consumer the consumer
*/
void addVertexAttributeConsumer(BiConsumer, Attribute> consumer);
/**
* Remove a vertex attribute consumer.
*
* @param consumer the consumer
*/
void removeVertexAttributeConsumer(BiConsumer, Attribute> consumer);
/**
* Add an edge attribute consumer.
*
* @param consumer the consumer
*/
void addEdgeAttributeConsumer(BiConsumer, Attribute> consumer);
/**
* Remove an edge attribute consumer.
*
* @param consumer the consumer
*/
void removeEdgeAttributeConsumer(BiConsumer, Attribute> consumer);
/**
* Import a graph
*
* @param input the input reader
* @throws ImportException in case any error occurs, such as I/O or parse error
*/
void importInput(Reader input);
/**
* Import a graph
*
* @param in the input stream
* @throws ImportException in case any error occurs, such as I/O or parse error
*/
default void importInput(InputStream in)
{
importInput(new InputStreamReader(in, StandardCharsets.UTF_8));
}
/**
* Import a graph
*
* @param file the file to read from
* @throws ImportException in case any error occurs, such as I/O or parse error
*/
default void importInput(File file)
{
try {
importInput(new InputStreamReader(new FileInputStream(file), StandardCharsets.UTF_8));
} catch (IOException e) {
throw new ImportException(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy