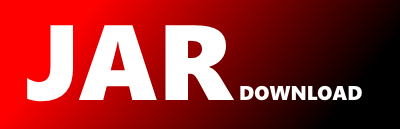
ngmf.ui.mms.MmsParamsReader Maven / Gradle / Ivy
/*
* MmsParamsReader.java
*
* Created on June 23, 2005, 4:46 PM
*
* To change this template, choose Tools | Options and locate the template under
* the Source Creation and Management node. Right-click the template and choose
* Open. You can then make changes to the template in the Source Editor.
*/
package ngmf.ui.mms;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.StringTokenizer;
import java.util.logging.Logger;
public class MmsParamsReader {
private FileReader file;
private Logger log;
private String fileName;
private ParameterSet mps;
public MmsParamsReader(String fileName) throws IOException {
this(new FileReader(fileName), Logger.getLogger(MmsParamsReader.class.getName()));
this.fileName = fileName;
}
public MmsParamsReader(FileReader file) throws IOException {
this (file, Logger.getLogger(MmsParamsReader.class.getName()));
}
public MmsParamsReader(FileReader file, Logger log) throws IOException {
this.file = file;
this.log = log;
}
public ParameterSet read() throws IOException {
mps = new MmsParameterSet();
mps.setFileName(fileName);
/*
* Read dimensions
*/
String line;
BufferedReader in = null;
try {
in = new BufferedReader(file);
mps.setDescription(in.readLine());
mps.setVersion(in.readLine());
line = in.readLine();
/*
* Read Dimensions
*/
while (line != null) {
if (line.equals("** Parameters **")) {
break;
} else if (line.startsWith(" 1) {
dims[1] = (MmsDimension)(mps.getDimension(dim2));
}
mps.addParameter(new MmsParameter(name, width, dims, type_class, vals));
line = in.readLine();
line = in.readLine();
}
} catch (IOException ex) {
log.severe("Problem reading parameters");
ex.printStackTrace();
throw ex;
} catch (NumberFormatException ex) {
log.severe("NumberFormatException while reading parameters");
} finally {
try {
if (in!= null) {
in.close();
in = null;
}
} catch (IOException E) {}
}
if (mps.getDims().isEmpty() && mps.getParams().isEmpty()) {
mps = null;
throw (new IOException ("Invalid MMS parameter file."));
}
return mps;
}
public static void main(String arg[]) {
try {
MmsParamsReader mp = new MmsParamsReader(new FileReader(arg[0]));
ParameterSet ps = mp.read();
System.out.println ("Dimensions = " + ps.getDims());
System.out.println ("Parameters = " + ps.getParams());
} catch (java.io.FileNotFoundException e) {
System.out.println(arg[0] + " not found");
} catch (IOException e) {
System.out.println(arg[0] + " io exception");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy