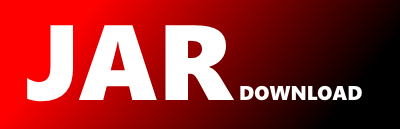
org.jibx.ota.destactivity.Travelers Maven / Gradle / Ivy
The newest version!
package org.jibx.ota.destactivity;
import java.util.ArrayList;
import java.util.List;
import org.jibx.ota.base.SpecialRequest;
import org.jibx.ota.base.TPAExtensions;
import org.jibx.ota.base.UnitsOfMeasureGroup;
import org.jibx.ota.profile.Profile;
/**
* A collection of Traveler objects, identifying the travelers.
*
* Schema fragment(s) for this class:
*
* <xs:complexType xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="TravelersType">
* <xs:sequence>
* <xs:element name="Traveler" maxOccurs="unbounded">
* <!-- Reference to inner class Traveler -->
* </xs:element>
* </xs:sequence>
* </xs:complexType>
*
*/
public class Travelers
{
private List travelerList = new ArrayList();
/**
* Get the list of 'Traveler' element items.
*
* @return list
*/
public List getTravelers() {
return travelerList;
}
/**
* Set the list of 'Traveler' element items.
*
* @param list
*/
public void setTravelers(List list) {
travelerList = list;
}
/**
* Get the number of 'Traveler' element items.
* @return count
*/
public int sizeTravelers() {
return travelerList.size();
}
/**
* Add a 'Traveler' element item.
* @param item
*/
public void addTraveler(Traveler item) {
travelerList.add(item);
}
/**
* Get 'Traveler' element item by position.
* @return item
* @param index
*/
public Traveler getTraveler(int index) {
return travelerList.get(index);
}
/**
* Remove all 'Traveler' element items.
*/
public void clearTravelers() {
travelerList.clear();
}
/**
* Contains the details of a traveler.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="Traveler" maxOccurs="unbounded">
* <xs:complexType>
* <xs:sequence>
* <xs:element type="ns:ProfileType" name="Profile" minOccurs="0"/>
* <xs:element name="TravelerCharacteristic" minOccurs="0" maxOccurs="2">
* <!-- Reference to inner class TravelerCharacteristic -->
* </xs:element>
* <xs:element type="ns:SpecialRequestType" name="SpecialRequests" minOccurs="0"/>
* <xs:element ref="ns:TPA_Extensions" minOccurs="0"/>
* </xs:sequence>
* <xs:attribute type="xs:string" use="optional" name="RPH"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class Traveler
{
private Profile profile;
private List travelerCharacteristicList = new ArrayList();
private SpecialRequest specialRequests;
private TPAExtensions TPAExtensions;
private String RPH;
/**
* Get the 'Profile' element value. Basic traveler profile.
*
* @return value
*/
public Profile getProfile() {
return profile;
}
/**
* Set the 'Profile' element value. Basic traveler profile.
*
* @param profile
*/
public void setProfile(Profile profile) {
this.profile = profile;
}
/**
* Get the list of 'TravelerCharacteristic' element items.
*
* @return list
*/
public List getTravelerCharacteristics() {
return travelerCharacteristicList;
}
/**
* Set the list of 'TravelerCharacteristic' element items.
*
* @param list
*/
public void setTravelerCharacteristics(List list) {
travelerCharacteristicList = list;
}
/**
* Get the number of 'TravelerCharacteristic' element items.
* @return count
*/
public int sizeTravelerCharacteristics() {
return travelerCharacteristicList.size();
}
/**
* Add a 'TravelerCharacteristic' element item.
* @param item
*/
public void addTravelerCharacteristic(TravelerCharacteristic item) {
travelerCharacteristicList.add(item);
}
/**
* Get 'TravelerCharacteristic' element item by position.
* @return item
* @param index
*/
public TravelerCharacteristic getTravelerCharacteristic(int index) {
return travelerCharacteristicList.get(index);
}
/**
* Remove all 'TravelerCharacteristic' element items.
*/
public void clearTravelerCharacteristics() {
travelerCharacteristicList.clear();
}
/**
* Get the 'SpecialRequests' element value. The SpecialRequest object indicates special requests for a particular traveler.
*
* @return value
*/
public SpecialRequest getSpecialRequests() {
return specialRequests;
}
/**
* Set the 'SpecialRequests' element value. The SpecialRequest object indicates special requests for a particular traveler.
*
* @param specialRequests
*/
public void setSpecialRequests(SpecialRequest specialRequests) {
this.specialRequests = specialRequests;
}
/**
* Get the 'TPA_Extensions' element value.
*
* @return value
*/
public TPAExtensions getTPAExtensions() {
return TPAExtensions;
}
/**
* Set the 'TPA_Extensions' element value.
*
* @param TPAExtensions
*/
public void setTPAExtensions(TPAExtensions TPAExtensions) {
this.TPAExtensions = TPAExtensions;
}
/**
* Get the 'RPH' attribute value. This is a reference placeholder, used as an index for this traveler in this reservation.
*
* @return value
*/
public String getRPH() {
return RPH;
}
/**
* Set the 'RPH' attribute value. This is a reference placeholder, used as an index for this traveler in this reservation.
*
* @param RPH
*/
public void setRPH(String RPH) {
this.RPH = RPH;
}
/**
* Used to define a traveler's physical characteristic.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="TravelerCharacteristic" minOccurs="0" maxOccurs="2">
* <xs:complexType>
* <xs:attribute use="optional" name="Type">
* <xs:simpleType>
* <!-- Reference to inner class Type -->
* </xs:simpleType>
* </xs:attribute>
* <xs:attributeGroup ref="ns:UnitsOfMeasureGroup"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class TravelerCharacteristic
{
private Type type;
private UnitsOfMeasureGroup unitsOfMeasureGroup;
/**
* Get the 'Type' attribute value. Specifies the traveler characteristic.
*
* @return value
*/
public Type getType() {
return type;
}
/**
* Set the 'Type' attribute value. Specifies the traveler characteristic.
*
* @param type
*/
public void setType(Type type) {
this.type = type;
}
/**
* Get the 'UnitsOfMeasureGroup' attributeGroup value. Used in conjunction with Type to quantify the traveler characteristic.
*
* @return value
*/
public UnitsOfMeasureGroup getUnitsOfMeasureGroup() {
return unitsOfMeasureGroup;
}
/**
* Set the 'UnitsOfMeasureGroup' attributeGroup value. Used in conjunction with Type to quantify the traveler characteristic.
*
* @param unitsOfMeasureGroup
*/
public void setUnitsOfMeasureGroup(
UnitsOfMeasureGroup unitsOfMeasureGroup) {
this.unitsOfMeasureGroup = unitsOfMeasureGroup;
}
/**
* Schema fragment(s) for this class:
*
* <xs:simpleType xmlns:xs="http://www.w3.org/2001/XMLSchema">
* <xs:restriction base="xs:string">
* <xs:enumeration value="Height"/>
* <xs:enumeration value="Weight"/>
* </xs:restriction>
* </xs:simpleType>
*
*/
public static enum Type {
HEIGHT("Height"), WEIGHT("Weight");
private final String value;
private Type(String value) {
this.value = value;
}
public String toString() {
return value;
}
public static Type convert(String value) {
for (Type inst : values()) {
if (inst.toString().equals(value)) {
return inst;
}
}
return null;
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy