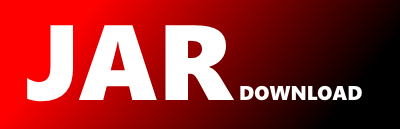
org.jibx.ota.loyalty.AccountInfo Maven / Gradle / Ivy
The newest version!
package org.jibx.ota.loyalty;
import java.util.ArrayList;
import java.util.List;
import org.jibx.ota.base.CompanyName;
import org.jibx.ota.base.ContactPerson;
import org.jibx.ota.base.PersonName;
import org.jibx.ota.base.PromotionCodeGroup;
/**
* Loyalty specific member profile information.
*
* Schema fragment(s) for this class:
*
* <xs:complexType xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="AccountInfoType">
* <xs:sequence>
* <xs:element type="ns:ContactPersonType" name="MemberInfo"/>
* <xs:element name="MemberPreferences" minOccurs="0">
* <!-- Reference to inner class MemberPreferences -->
* </xs:element>
* <xs:element name="SecurityInfo" minOccurs="0">
* <!-- Reference to inner class SecurityInfo -->
* </xs:element>
* <xs:element name="SubAccountBalance" minOccurs="0" maxOccurs="99">
* <!-- Reference to inner class SubAccountBalance -->
* </xs:element>
* </xs:sequence>
* <xs:attribute type="xs:int" use="optional" name="PointBalance"/>
* <xs:attribute use="optional" name="EnrollmentType">
* <xs:simpleType>
* <!-- Reference to inner class Enrollment -->
* </xs:simpleType>
* </xs:attribute>
* <xs:attribute type="xs:string" use="optional" name="EnrollmentMethod"/>
* </xs:complexType>
*
*/
public class AccountInfo
{
private ContactPerson memberInfo;
private MemberPreferences memberPreferences;
private SecurityInfo securityInfo;
private List subAccountBalanceList = new ArrayList();
private Integer pointBalance;
private Enrollment enrollment;
private String enrollmentMethod;
/**
* Get the 'MemberInfo' element value. Member information including name, contact information and employee information.
*
* @return value
*/
public ContactPerson getMemberInfo() {
return memberInfo;
}
/**
* Set the 'MemberInfo' element value. Member information including name, contact information and employee information.
*
* @param memberInfo
*/
public void setMemberInfo(ContactPerson memberInfo) {
this.memberInfo = memberInfo;
}
/**
* Get the 'MemberPreferences' element value.
*
* @return value
*/
public MemberPreferences getMemberPreferences() {
return memberPreferences;
}
/**
* Set the 'MemberPreferences' element value.
*
* @param memberPreferences
*/
public void setMemberPreferences(MemberPreferences memberPreferences) {
this.memberPreferences = memberPreferences;
}
/**
* Get the 'SecurityInfo' element value.
*
* @return value
*/
public SecurityInfo getSecurityInfo() {
return securityInfo;
}
/**
* Set the 'SecurityInfo' element value.
*
* @param securityInfo
*/
public void setSecurityInfo(SecurityInfo securityInfo) {
this.securityInfo = securityInfo;
}
/**
* Get the list of 'SubAccountBalance' element items.
*
* @return list
*/
public List getSubAccountBalances() {
return subAccountBalanceList;
}
/**
* Set the list of 'SubAccountBalance' element items.
*
* @param list
*/
public void setSubAccountBalances(List list) {
subAccountBalanceList = list;
}
/**
* Get the number of 'SubAccountBalance' element items.
* @return count
*/
public int sizeSubAccountBalances() {
return subAccountBalanceList.size();
}
/**
* Add a 'SubAccountBalance' element item.
* @param item
*/
public void addSubAccountBalance(SubAccountBalance item) {
subAccountBalanceList.add(item);
}
/**
* Get 'SubAccountBalance' element item by position.
* @return item
* @param index
*/
public SubAccountBalance getSubAccountBalance(int index) {
return subAccountBalanceList.get(index);
}
/**
* Remove all 'SubAccountBalance' element items.
*/
public void clearSubAccountBalances() {
subAccountBalanceList.clear();
}
/**
* Get the 'PointBalance' attribute value. The point balance for a loyalty account.
*
* @return value
*/
public Integer getPointBalance() {
return pointBalance;
}
/**
* Set the 'PointBalance' attribute value. The point balance for a loyalty account.
*
* @param pointBalance
*/
public void setPointBalance(Integer pointBalance) {
this.pointBalance = pointBalance;
}
/**
* Get the 'EnrollmentType' attribute value. Method in which enrollment occurs.
*
* @return value
*/
public Enrollment getEnrollment() {
return enrollment;
}
/**
* Set the 'EnrollmentType' attribute value. Method in which enrollment occurs.
*
* @param enrollment
*/
public void setEnrollment(Enrollment enrollment) {
this.enrollment = enrollment;
}
/**
* Get the 'EnrollmentMethod' attribute value. Means by which the enrollment was initiated. Refer to OpenTravel Code List Enrollment Method (ENR).
*
* @return value
*/
public String getEnrollmentMethod() {
return enrollmentMethod;
}
/**
* Set the 'EnrollmentMethod' attribute value. Means by which the enrollment was initiated. Refer to OpenTravel Code List Enrollment Method (ENR).
*
* @param enrollmentMethod
*/
public void setEnrollmentMethod(String enrollmentMethod) {
this.enrollmentMethod = enrollmentMethod;
}
/**
* Loyalty program preferences specified by the enrolling member.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="MemberPreferences" minOccurs="0">
* <xs:complexType>
* <xs:sequence>
* <xs:element name="AdditionalReward" minOccurs="0" maxOccurs="5">
* <!-- Reference to inner class AdditionalReward -->
* </xs:element>
* <xs:element name="Offer" minOccurs="0" maxOccurs="5">
* <!-- Reference to inner class Offer -->
* </xs:element>
* </xs:sequence>
* <xs:attribute type="xs:string" use="optional" name="Awareness"/>
* <xs:attributeGroup ref="ns:PromotionCodeGroup"/>
* <xs:attribute use="optional" name="AwardsPreference">
* <xs:simpleType>
* <!-- Reference to inner class AwardsPreference -->
* </xs:simpleType>
* </xs:attribute>
* </xs:complexType>
* </xs:element>
*
*/
public static class MemberPreferences
{
private List additionalRewardList = new ArrayList();
private List offerList = new ArrayList();
private String awareness;
private PromotionCodeGroup promotionCodeGroup;
private AwardsPreference awardsPreference;
/**
* Get the list of 'AdditionalReward' element items.
*
* @return list
*/
public List getAdditionalRewards() {
return additionalRewardList;
}
/**
* Set the list of 'AdditionalReward' element items.
*
* @param list
*/
public void setAdditionalRewards(List list) {
additionalRewardList = list;
}
/**
* Get the number of 'AdditionalReward' element items.
* @return count
*/
public int sizeAdditionalRewards() {
return additionalRewardList.size();
}
/**
* Add a 'AdditionalReward' element item.
* @param item
*/
public void addAdditionalReward(AdditionalReward item) {
additionalRewardList.add(item);
}
/**
* Get 'AdditionalReward' element item by position.
* @return item
* @param index
*/
public AdditionalReward getAdditionalReward(int index) {
return additionalRewardList.get(index);
}
/**
* Remove all 'AdditionalReward' element items.
*/
public void clearAdditionalRewards() {
additionalRewardList.clear();
}
/**
* Get the list of 'Offer' element items.
*
* @return list
*/
public List getOffers() {
return offerList;
}
/**
* Set the list of 'Offer' element items.
*
* @param list
*/
public void setOffers(List list) {
offerList = list;
}
/**
* Get the number of 'Offer' element items.
* @return count
*/
public int sizeOffers() {
return offerList.size();
}
/**
* Add a 'Offer' element item.
* @param item
*/
public void addOffer(Offer item) {
offerList.add(item);
}
/**
* Get 'Offer' element item by position.
* @return item
* @param index
*/
public Offer getOffer(int index) {
return offerList.get(index);
}
/**
* Remove all 'Offer' element items.
*/
public void clearOffers() {
offerList.clear();
}
/**
* Get the 'Awareness' attribute value. Indicates how person became aware of loyalty program.
*
* @return value
*/
public String getAwareness() {
return awareness;
}
/**
* Set the 'Awareness' attribute value. Indicates how person became aware of loyalty program.
*
* @param awareness
*/
public void setAwareness(String awareness) {
this.awareness = awareness;
}
/**
* Get the 'PromotionCodeGroup' attributeGroup value. Loyalty enrollment promotion code.
*
* @return value
*/
public PromotionCodeGroup getPromotionCodeGroup() {
return promotionCodeGroup;
}
/**
* Set the 'PromotionCodeGroup' attributeGroup value. Loyalty enrollment promotion code.
*
* @param promotionCodeGroup
*/
public void setPromotionCodeGroup(PromotionCodeGroup promotionCodeGroup) {
this.promotionCodeGroup = promotionCodeGroup;
}
/**
* Get the 'AwardsPreference' attribute value. Method by which awards are allocated.
*
* @return value
*/
public AwardsPreference getAwardsPreference() {
return awardsPreference;
}
/**
* Set the 'AwardsPreference' attribute value. Method by which awards are allocated.
*
* @param awardsPreference
*/
public void setAwardsPreference(AwardsPreference awardsPreference) {
this.awardsPreference = awardsPreference;
}
/**
* Additional programs that are honored by the primary loyalty account.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="AdditionalReward" minOccurs="0" maxOccurs="5">
* <xs:complexType>
* <xs:sequence>
* <xs:element type="ns:CompanyNameType" name="CompanyName" minOccurs="0"/>
* <xs:element type="ns:PersonNameType" name="Name" minOccurs="0"/>
* </xs:sequence>
* <xs:attribute type="xs:string" use="optional" name="MemberID"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class AdditionalReward
{
private CompanyName companyName;
private PersonName name;
private String memberID;
/**
* Get the 'CompanyName' element value.
*
* @return value
*/
public CompanyName getCompanyName() {
return companyName;
}
/**
* Set the 'CompanyName' element value.
*
* @param companyName
*/
public void setCompanyName(CompanyName companyName) {
this.companyName = companyName;
}
/**
* Get the 'Name' element value.
*
* @return value
*/
public PersonName getName() {
return name;
}
/**
* Set the 'Name' element value.
*
* @param name
*/
public void setName(PersonName name) {
this.name = name;
}
/**
* Get the 'MemberID' attribute value.
*
* @return value
*/
public String getMemberID() {
return memberID;
}
/**
* Set the 'MemberID' attribute value.
*
* @param memberID
*/
public void setMemberID(String memberID) {
this.memberID = memberID;
}
}
/**
* Source from which members can receive information.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="Offer" minOccurs="0" maxOccurs="5">
* <xs:complexType>
* <xs:sequence>
* <xs:element name="Communication" minOccurs="0" maxOccurs="5">
* <!-- Reference to inner class Communication -->
* </xs:element>
* </xs:sequence>
* <xs:attribute use="optional" name="Type">
* <xs:simpleType>
* <!-- Reference to inner class Type -->
* </xs:simpleType>
* </xs:attribute>
* </xs:complexType>
* </xs:element>
*
*/
public static class Offer
{
private List communicationList = new ArrayList();
private Type type;
/**
* Get the list of 'Communication' element items.
*
* @return list
*/
public List getCommunications() {
return communicationList;
}
/**
* Set the list of 'Communication' element items.
*
* @param list
*/
public void setCommunications(List list) {
communicationList = list;
}
/**
* Get the number of 'Communication' element items.
* @return count
*/
public int sizeCommunications() {
return communicationList.size();
}
/**
* Add a 'Communication' element item.
* @param item
*/
public void addCommunication(Communication item) {
communicationList.add(item);
}
/**
* Get 'Communication' element item by position.
* @return item
* @param index
*/
public Communication getCommunication(int index) {
return communicationList.get(index);
}
/**
* Remove all 'Communication' element items.
*/
public void clearCommunications() {
communicationList.clear();
}
/**
* Get the 'Type' attribute value. An enumerated list of offer sources.
*
* @return value
*/
public Type getType() {
return type;
}
/**
* Set the 'Type' attribute value. An enumerated list of offer sources.
*
* @param type
*/
public void setType(Type type) {
this.type = type;
}
/**
* Preferred method of offer communication.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="Communication" minOccurs="0" maxOccurs="5">
* <xs:complexType>
* <xs:attribute type="xs:string" use="optional" name="DistribType"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class Communication
{
private String distrib;
/**
* Get the 'DistribType' attribute value. An enumerated list of method of communication. Refer to OpenTravel Code List Distribution Type (DTB).
*
* @return value
*/
public String getDistrib() {
return distrib;
}
/**
* Set the 'DistribType' attribute value. An enumerated list of method of communication. Refer to OpenTravel Code List Distribution Type (DTB).
*
* @param distrib
*/
public void setDistrib(String distrib) {
this.distrib = distrib;
}
}
/**
* Schema fragment(s) for this class:
*
* <xs:simpleType xmlns:xs="http://www.w3.org/2001/XMLSchema">
* <xs:restriction base="xs:string">
* <xs:enumeration value="Partner"/>
* <xs:enumeration value="Loyalty"/>
* </xs:restriction>
* </xs:simpleType>
*
*/
public static enum Type {
PARTNER("Partner"), LOYALTY("Loyalty");
private final String value;
private Type(String value) {
this.value = value;
}
public String toString() {
return value;
}
public static Type convert(String value) {
for (Type inst : values()) {
if (inst.toString().equals(value)) {
return inst;
}
}
return null;
}
}
}
/**
* Schema fragment(s) for this class:
*
* <xs:simpleType xmlns:xs="http://www.w3.org/2001/XMLSchema">
* <xs:restriction base="xs:string">
* <xs:enumeration value="Points"/>
* <xs:enumeration value="Miles"/>
* </xs:restriction>
* </xs:simpleType>
*
*/
public static enum AwardsPreference {
POINTS("Points"), MILES("Miles");
private final String value;
private AwardsPreference(String value) {
this.value = value;
}
public String toString() {
return value;
}
public static AwardsPreference convert(String value) {
for (AwardsPreference inst : values()) {
if (inst.toString().equals(value)) {
return inst;
}
}
return null;
}
}
}
/**
* Information allowing member to securely access account.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="SecurityInfo" minOccurs="0">
* <xs:complexType>
* <xs:sequence>
* <xs:element name="PasswordHint" minOccurs="0" maxOccurs="2">
* <!-- Reference to inner class PasswordHint -->
* </xs:element>
* </xs:sequence>
* <xs:attribute type="xs:string" name="Username"/>
* <xs:attribute type="xs:string" name="Password"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class SecurityInfo
{
private List passwordHintList = new ArrayList();
private String username;
private String password;
/**
* Get the list of 'PasswordHint' element items.
*
* @return list
*/
public List getPasswordHints() {
return passwordHintList;
}
/**
* Set the list of 'PasswordHint' element items.
*
* @param list
*/
public void setPasswordHints(List list) {
passwordHintList = list;
}
/**
* Get the number of 'PasswordHint' element items.
* @return count
*/
public int sizePasswordHints() {
return passwordHintList.size();
}
/**
* Add a 'PasswordHint' element item.
* @param item
*/
public void addPasswordHint(PasswordHint item) {
passwordHintList.add(item);
}
/**
* Get 'PasswordHint' element item by position.
* @return item
* @param index
*/
public PasswordHint getPasswordHint(int index) {
return passwordHintList.get(index);
}
/**
* Remove all 'PasswordHint' element items.
*/
public void clearPasswordHints() {
passwordHintList.clear();
}
/**
* Get the 'Username' attribute value.
*
* @return value
*/
public String getUsername() {
return username;
}
/**
* Set the 'Username' attribute value.
*
* @param username
*/
public void setUsername(String username) {
this.username = username;
}
/**
* Get the 'Password' attribute value.
*
* @return value
*/
public String getPassword() {
return password;
}
/**
* Set the 'Password' attribute value.
*
* @param password
*/
public void setPassword(String password) {
this.password = password;
}
/**
* Alternate method to password for account access.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="PasswordHint" minOccurs="0" maxOccurs="2">
* <xs:complexType>
* <xs:simpleContent>
* <xs:extension base="xs:string">
* <xs:attribute use="optional" name="Hint">
* <xs:simpleType>
* <!-- Reference to inner class Hint -->
* </xs:simpleType>
* </xs:attribute>
* </xs:extension>
* </xs:simpleContent>
* </xs:complexType>
* </xs:element>
*
*/
public static class PasswordHint
{
private String string;
private Hint hint;
/**
* Get the extension value.
*
* @return value
*/
public String getString() {
return string;
}
/**
* Set the extension value.
*
* @param string
*/
public void setString(String string) {
this.string = string;
}
/**
* Get the 'Hint' attribute value.
*
* @return value
*/
public Hint getHint() {
return hint;
}
/**
* Set the 'Hint' attribute value.
*
* @param hint
*/
public void setHint(Hint hint) {
this.hint = hint;
}
/**
* Schema fragment(s) for this class:
*
* <xs:simpleType xmlns:xs="http://www.w3.org/2001/XMLSchema">
* <xs:restriction base="xs:string">
* <xs:enumeration value="Question"/>
* <xs:enumeration value="Answer"/>
* </xs:restriction>
* </xs:simpleType>
*
*/
public static enum Hint {
QUESTION("Question"), ANSWER("Answer");
private final String value;
private Hint(String value) {
this.value = value;
}
public String toString() {
return value;
}
public static Hint convert(String value) {
for (Hint inst : values()) {
if (inst.toString().equals(value)) {
return inst;
}
}
return null;
}
}
}
}
/**
* Used to specify a sub-account and its point balance associated with this loyalty account.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="SubAccountBalance" minOccurs="0" maxOccurs="99">
* <xs:complexType>
* <xs:attribute type="xs:string" use="optional" name="Type"/>
* <xs:attribute type="xs:int" use="optional" name="Balance"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class SubAccountBalance
{
private String type;
private Integer balance;
/**
* Get the 'Type' attribute value. Describes the type of sub account (e.g. miles, points, vouchers, stays).
*
* @return value
*/
public String getType() {
return type;
}
/**
* Set the 'Type' attribute value. Describes the type of sub account (e.g. miles, points, vouchers, stays).
*
* @param type
*/
public void setType(String type) {
this.type = type;
}
/**
* Get the 'Balance' attribute value. The current balance for this sub account.
*
* @return value
*/
public Integer getBalance() {
return balance;
}
/**
* Set the 'Balance' attribute value. The current balance for this sub account.
*
* @param balance
*/
public void setBalance(Integer balance) {
this.balance = balance;
}
}
/**
* Schema fragment(s) for this class:
*
* <xs:simpleType xmlns:xs="http://www.w3.org/2001/XMLSchema">
* <xs:restriction base="xs:string">
* <xs:enumeration value="Full"/>
* <xs:enumeration value="Partial"/>
* </xs:restriction>
* </xs:simpleType>
*
*/
public static enum Enrollment {
FULL("Full"), PARTIAL("Partial");
private final String value;
private Enrollment(String value) {
this.value = value;
}
public String toString() {
return value;
}
public static Enrollment convert(String value) {
for (Enrollment inst : values()) {
if (inst.toString().equals(value)) {
return inst;
}
}
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy