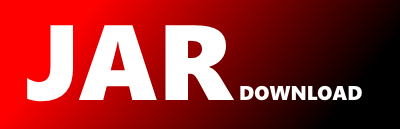
org.jibx.ota.loyalty.AccountRS Maven / Gradle / Ivy
The newest version!
package org.jibx.ota.loyalty;
import org.jibx.ota.base.Action;
import org.jibx.ota.base.CustomerLoyaltyGroup;
import org.jibx.ota.base.Errors;
import org.jibx.ota.base.Location;
import org.jibx.ota.base.OTAPayloadStdAttributes;
import org.jibx.ota.base.Success;
import org.jibx.ota.base.UniqueID;
import org.jibx.ota.base.Warnings;
/**
* This message may be used to respond to a loyalty account create request or a loyalty account read request.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="OTA_LoyaltyAccountRS">
* <xs:complexType>
* <xs:choice>
* <xs:sequence>
* <xs:element type="ns:SuccessType" name="Success"/>
* <xs:element type="ns:WarningsType" name="Warnings" minOccurs="0"/>
* <xs:element type="ns:UniqueID_Type" name="UniqueID"/>
* <xs:element name="AccountInfo" minOccurs="0">
* <!-- Reference to inner class AccountInfo -->
* </xs:element>
* <xs:element type="ns:LocationType" name="Location" minOccurs="0"/>
* </xs:sequence>
* <xs:element type="ns:ErrorsType" name="Errors"/>
* </xs:choice>
* <xs:attributeGroup ref="ns:OTA_PayloadStdAttributes"/>
* </xs:complexType>
* </xs:element>
*
*/
public class AccountRS
{
private int choiceSelect = -1;
private static final int SUCCESS_CHOICE = 0;
private static final int ERRORS_CHOICE = 1;
private Success success;
private Warnings warnings;
private UniqueID uniqueID;
private AccountInfo accountInfo;
private Location location;
private Errors errors;
private OTAPayloadStdAttributes OTAPayloadStdAttributes;
private void setChoiceSelect(int choice) {
if (choiceSelect == -1) {
choiceSelect = choice;
} else if (choiceSelect != choice) {
throw new IllegalStateException(
"Need to call clearChoiceSelect() before changing existing choice");
}
}
/**
* Clear the choice selection.
*/
public void clearChoiceSelect() {
choiceSelect = -1;
}
/**
* Check if Success is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifSuccess() {
return choiceSelect == SUCCESS_CHOICE;
}
/**
* Get the 'Success' element value.
*
* @return value
*/
public Success getSuccess() {
return success;
}
/**
* Set the 'Success' element value.
*
* @param success
*/
public void setSuccess(Success success) {
setChoiceSelect(SUCCESS_CHOICE);
this.success = success;
}
/**
* Get the 'Warnings' element value.
*
* @return value
*/
public Warnings getWarnings() {
return warnings;
}
/**
* Set the 'Warnings' element value.
*
* @param warnings
*/
public void setWarnings(Warnings warnings) {
setChoiceSelect(SUCCESS_CHOICE);
this.warnings = warnings;
}
/**
* Get the 'UniqueID' element value.
*
* @return value
*/
public UniqueID getUniqueID() {
return uniqueID;
}
/**
* Set the 'UniqueID' element value.
*
* @param uniqueID
*/
public void setUniqueID(UniqueID uniqueID) {
setChoiceSelect(SUCCESS_CHOICE);
this.uniqueID = uniqueID;
}
/**
* Get the 'AccountInfo' element value.
*
* @return value
*/
public AccountInfo getAccountInfo() {
return accountInfo;
}
/**
* Set the 'AccountInfo' element value.
*
* @param accountInfo
*/
public void setAccountInfo(AccountInfo accountInfo) {
setChoiceSelect(SUCCESS_CHOICE);
this.accountInfo = accountInfo;
}
/**
* Get the 'Location' element value. The location code where the enrollment originated.
*
* @return value
*/
public Location getLocation() {
return location;
}
/**
* Set the 'Location' element value. The location code where the enrollment originated.
*
* @param location
*/
public void setLocation(Location location) {
setChoiceSelect(SUCCESS_CHOICE);
this.location = location;
}
/**
* Check if Errors is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifErrors() {
return choiceSelect == ERRORS_CHOICE;
}
/**
* Get the 'Errors' element value.
*
* @return value
*/
public Errors getErrors() {
return errors;
}
/**
* Set the 'Errors' element value.
*
* @param errors
*/
public void setErrors(Errors errors) {
setChoiceSelect(ERRORS_CHOICE);
this.errors = errors;
}
/**
* Get the 'OTA_PayloadStdAttributes' attributeGroup value.
*
* @return value
*/
public OTAPayloadStdAttributes getOTAPayloadStdAttributes() {
return OTAPayloadStdAttributes;
}
/**
* Set the 'OTA_PayloadStdAttributes' attributeGroup value.
*
* @param OTAPayloadStdAttributes
*/
public void setOTAPayloadStdAttributes(
OTAPayloadStdAttributes OTAPayloadStdAttributes) {
this.OTAPayloadStdAttributes = OTAPayloadStdAttributes;
}
/**
* Loyalty account information, including member information, preferences, security and sub-account balances.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="AccountInfo" minOccurs="0">
* <xs:complexType>
* <xs:complexContent>
* <xs:extension base="ns:AccountInfoType">
* <xs:sequence>
* <xs:element name="CustLoyalty" minOccurs="0">
* <xs:complexType>
* <xs:attributeGroup ref="ns:CustomerLoyaltyGroup"/>
* </xs:complexType>
* </xs:element>
* </xs:sequence>
* <xs:attribute type="ns:ActionType" name="Action"/>
* </xs:extension>
* </xs:complexContent>
* </xs:complexType>
* </xs:element>
*
*/
public static class AccountInfo extends org.jibx.ota.loyalty.AccountInfo
{
private CustomerLoyaltyGroup custLoyalty;
private Action action;
/**
* Get the 'CustomerLoyaltyGroup' attributeGroup value. Further account information.
*
* @return value
*/
public CustomerLoyaltyGroup getCustLoyalty() {
return custLoyalty;
}
/**
* Set the 'CustomerLoyaltyGroup' attributeGroup value. Further account information.
*
* @param custLoyalty
*/
public void setCustLoyalty(CustomerLoyaltyGroup custLoyalty) {
this.custLoyalty = custLoyalty;
}
/**
* Get the 'Action' attribute value. The action that has been applied to the account information.
*
* @return value
*/
public Action getAction() {
return action;
}
/**
* Set the 'Action' attribute value. The action that has been applied to the account information.
*
* @param action
*/
public void setAction(Action action) {
this.action = action;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy