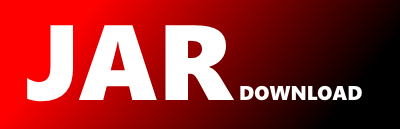
org.jibx.ota.loyalty.CertificateCreateRQ Maven / Gradle / Ivy
The newest version!
package org.jibx.ota.loyalty;
import java.util.ArrayList;
import java.util.List;
import org.jibx.ota.base.LoyaltyCertificateNumberGroup;
import org.jibx.ota.base.OTAPayloadStdAttributes;
import org.jibx.ota.base.POS;
import org.jibx.ota.base.PersonName;
/**
* The CertificateCreateRQ allows businesses to communicate with their loyalty service provider to generate redemption certificates for their customers.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="OTA_LoyaltyCertificateCreateRQ">
* <xs:complexType>
* <xs:sequence>
* <xs:element type="ns:POS_Type" name="POS" minOccurs="0"/>
* <xs:element name="Customer" minOccurs="0" maxOccurs="999">
* <!-- Reference to inner class Customer -->
* </xs:element>
* <xs:element name="TravelInfos">
* <xs:complexType>
* <xs:sequence>
* <xs:element type="ns:LoyaltyTravelInfoType" name="TravelInfo" maxOccurs="99"/>
* <xs:element name="LoyaltyCertificateDetail" minOccurs="0" maxOccurs="999">
* <!-- Reference to inner class LoyaltyCertificateDetail -->
* </xs:element>
* </xs:sequence>
* </xs:complexType>
* </xs:element>
* </xs:sequence>
* <xs:attributeGroup ref="ns:OTA_PayloadStdAttributes"/>
* </xs:complexType>
* </xs:element>
*
*/
public class CertificateCreateRQ
{
private POS POS;
private List customerList = new ArrayList();
private List travelInfosTravelInfoList = new ArrayList();
private List loyaltyCertificateDetailList = new ArrayList();
private OTAPayloadStdAttributes OTAPayloadStdAttributes;
/**
* Get the 'POS' element value. This identifies the party making the request. Trading partners are able to define their own UniqueID.
*
* @return value
*/
public POS getPOS() {
return POS;
}
/**
* Set the 'POS' element value. This identifies the party making the request. Trading partners are able to define their own UniqueID.
*
* @param POS
*/
public void setPOS(POS POS) {
this.POS = POS;
}
/**
* Get the list of 'Customer' element items.
*
* @return list
*/
public List getCustomers() {
return customerList;
}
/**
* Set the list of 'Customer' element items.
*
* @param list
*/
public void setCustomers(List list) {
customerList = list;
}
/**
* Get the number of 'Customer' element items.
* @return count
*/
public int sizeCustomers() {
return customerList.size();
}
/**
* Add a 'Customer' element item.
* @param item
*/
public void addCustomer(Customer item) {
customerList.add(item);
}
/**
* Get 'Customer' element item by position.
* @return item
* @param index
*/
public Customer getCustomer(int index) {
return customerList.get(index);
}
/**
* Remove all 'Customer' element items.
*/
public void clearCustomers() {
customerList.clear();
}
/**
* Get the list of 'TravelInfo' element items. Information about the customers trip, including hotel stay, air flight and car rental information.
*
* @return list
*/
public List getTravelInfosTravelInfos() {
return travelInfosTravelInfoList;
}
/**
* Set the list of 'TravelInfo' element items. Information about the customers trip, including hotel stay, air flight and car rental information.
*
* @param list
*/
public void setTravelInfosTravelInfos(List list) {
travelInfosTravelInfoList = list;
}
/**
* Get the number of 'TravelInfo' element items.
* @return count
*/
public int sizeTravelInfosTravelInfos() {
return travelInfosTravelInfoList.size();
}
/**
* Add a 'TravelInfo' element item.
* @param item
*/
public void addTravelInfosTravelInfo(LoyaltyTravelInfo item) {
travelInfosTravelInfoList.add(item);
}
/**
* Get 'TravelInfo' element item by position.
* @return item
* @param index
*/
public LoyaltyTravelInfo getTravelInfosTravelInfo(int index) {
return travelInfosTravelInfoList.get(index);
}
/**
* Remove all 'TravelInfo' element items.
*/
public void clearTravelInfosTravelInfos() {
travelInfosTravelInfoList.clear();
}
/**
* Get the list of 'LoyaltyCertificateDetail' element items. Information about the customers trip and loyalty program.
*
* @return list
*/
public List getLoyaltyCertificateDetails() {
return loyaltyCertificateDetailList;
}
/**
* Set the list of 'LoyaltyCertificateDetail' element items. Information about the customers trip and loyalty program.
*
* @param list
*/
public void setLoyaltyCertificateDetails(List list) {
loyaltyCertificateDetailList = list;
}
/**
* Get the number of 'LoyaltyCertificateDetail' element items.
* @return count
*/
public int sizeLoyaltyCertificateDetails() {
return loyaltyCertificateDetailList.size();
}
/**
* Add a 'LoyaltyCertificateDetail' element item.
* @param item
*/
public void addLoyaltyCertificateDetail(LoyaltyCertificateDetail item) {
loyaltyCertificateDetailList.add(item);
}
/**
* Get 'LoyaltyCertificateDetail' element item by position.
* @return item
* @param index
*/
public LoyaltyCertificateDetail getLoyaltyCertificateDetail(int index) {
return loyaltyCertificateDetailList.get(index);
}
/**
* Remove all 'LoyaltyCertificateDetail' element items.
*/
public void clearLoyaltyCertificateDetails() {
loyaltyCertificateDetailList.clear();
}
/**
* Get the 'OTA_PayloadStdAttributes' attributeGroup value.
*
* @return value
*/
public OTAPayloadStdAttributes getOTAPayloadStdAttributes() {
return OTAPayloadStdAttributes;
}
/**
* Set the 'OTA_PayloadStdAttributes' attributeGroup value.
*
* @param OTAPayloadStdAttributes
*/
public void setOTAPayloadStdAttributes(
OTAPayloadStdAttributes OTAPayloadStdAttributes) {
this.OTAPayloadStdAttributes = OTAPayloadStdAttributes;
}
/**
* Customer for whom a certificate is created.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="Customer" minOccurs="0" maxOccurs="999">
* <xs:complexType>
* <xs:complexContent>
* <xs:extension base="ns:PersonNameType">
* <xs:attribute type="xs:string" use="optional" name="RPH"/>
* <xs:attribute type="xs:string" use="optional" name="ResBookDesigCode"/>
* <xs:attribute type="xs:string" use="optional" name="FlightRPH"/>
* </xs:extension>
* </xs:complexContent>
* </xs:complexType>
* </xs:element>
*
*/
public static class Customer extends PersonName
{
private String RPH;
private String resBookDesigCode;
private String flightRPH;
/**
* Get the 'RPH' attribute value. The Reference Place Holder (RPH) is an index code used to identify an instance in a collection of like items (e.g. used to assign individual passengers or clients to particular itinerary items).
*
* @return value
*/
public String getRPH() {
return RPH;
}
/**
* Set the 'RPH' attribute value. The Reference Place Holder (RPH) is an index code used to identify an instance in a collection of like items (e.g. used to assign individual passengers or clients to particular itinerary items).
*
* @param RPH
*/
public void setRPH(String RPH) {
this.RPH = RPH;
}
/**
* Get the 'ResBookDesigCode' attribute value. Booking class of this customer.
*
* @return value
*/
public String getResBookDesigCode() {
return resBookDesigCode;
}
/**
* Set the 'ResBookDesigCode' attribute value. Booking class of this customer.
*
* @param resBookDesigCode
*/
public void setResBookDesigCode(String resBookDesigCode) {
this.resBookDesigCode = resBookDesigCode;
}
/**
* Get the 'FlightRPH' attribute value. Reference to the flight for which the certificate is requested.
*
* @return value
*/
public String getFlightRPH() {
return flightRPH;
}
/**
* Set the 'FlightRPH' attribute value. Reference to the flight for which the certificate is requested.
*
* @param flightRPH
*/
public void setFlightRPH(String flightRPH) {
this.flightRPH = flightRPH;
}
}
/**
* Information about the customers loyalty program and certificates.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="LoyaltyCertificateDetail" minOccurs="0" maxOccurs="999">
* <xs:complexType>
* <xs:attributeGroup ref="ns:LoyaltyCertificateNumberGroup"/>
* <xs:attribute type="xs:string" use="optional" name="PinNumber"/>
* <xs:attribute type="xs:string" use="optional" name="CustomerRPH"/>
* <xs:attribute type="xs:string" use="optional" name="FlightRPH"/>
* <xs:attribute type="xs:string" use="optional" name="AwardType"/>
* <xs:attribute type="xs:int" use="optional" name="CertificateRefNumber"/>
* </xs:complexType>
* </xs:element>
*
*/
public static class LoyaltyCertificateDetail
{
private LoyaltyCertificateNumberGroup loyaltyCertificateNumberGroup;
private String pinNumber;
private String customerRPH;
private String flightRPH;
private String award;
private Integer certificateRefNumber;
/**
* Get the 'LoyaltyCertificateNumberGroup' attributeGroup value.
*
* @return value
*/
public LoyaltyCertificateNumberGroup getLoyaltyCertificateNumberGroup() {
return loyaltyCertificateNumberGroup;
}
/**
* Set the 'LoyaltyCertificateNumberGroup' attributeGroup value.
*
* @param loyaltyCertificateNumberGroup
*/
public void setLoyaltyCertificateNumberGroup(
LoyaltyCertificateNumberGroup loyaltyCertificateNumberGroup) {
this.loyaltyCertificateNumberGroup = loyaltyCertificateNumberGroup;
}
/**
* Get the 'PinNumber' attribute value. The personal identification number (password) for the Member Number.
*
* @return value
*/
public String getPinNumber() {
return pinNumber;
}
/**
* Set the 'PinNumber' attribute value. The personal identification number (password) for the Member Number.
*
* @param pinNumber
*/
public void setPinNumber(String pinNumber) {
this.pinNumber = pinNumber;
}
/**
* Get the 'CustomerRPH' attribute value. Reference of the customer the certificate is requested for.
*
* @return value
*/
public String getCustomerRPH() {
return customerRPH;
}
/**
* Set the 'CustomerRPH' attribute value. Reference of the customer the certificate is requested for.
*
* @param customerRPH
*/
public void setCustomerRPH(String customerRPH) {
this.customerRPH = customerRPH;
}
/**
* Get the 'FlightRPH' attribute value. Reference of the flight the certificate is requested for.
*
* @return value
*/
public String getFlightRPH() {
return flightRPH;
}
/**
* Set the 'FlightRPH' attribute value. Reference of the flight the certificate is requested for.
*
* @param flightRPH
*/
public void setFlightRPH(String flightRPH) {
this.flightRPH = flightRPH;
}
/**
* Get the 'AwardType' attribute value. Describes the currency type to be deducted (e.g. miles, points, vouchers, stays).
*
* @return value
*/
public String getAward() {
return award;
}
/**
* Set the 'AwardType' attribute value. Describes the currency type to be deducted (e.g. miles, points, vouchers, stays).
*
* @param award
*/
public void setAward(String award) {
this.award = award;
}
/**
* Get the 'CertificateRefNumber' attribute value. A unique reference for the created certificate.
*
* @return value
*/
public Integer getCertificateRefNumber() {
return certificateRefNumber;
}
/**
* Set the 'CertificateRefNumber' attribute value. A unique reference for the created certificate.
*
* @param certificateRefNumber
*/
public void setCertificateRefNumber(Integer certificateRefNumber) {
this.certificateRefNumber = certificateRefNumber;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy