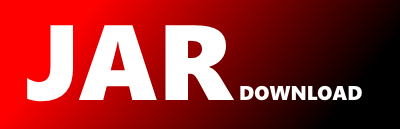
org.jibx.ota.loyalty.CertificateRedemptionRQ Maven / Gradle / Ivy
The newest version!
package org.jibx.ota.loyalty;
import java.util.ArrayList;
import java.util.List;
import org.jibx.ota.base.LoyaltyCertificateNumberGroup;
import org.jibx.ota.base.OTAPayloadStdAttributes;
import org.jibx.ota.base.PersonName;
import org.jibx.ota.base.PromotionCodeGroup;
/**
* The CertificateRedemptionRQ allows businesses to communicate with their loyalty service provider to generate redemption certificates for their customers.
*
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="http://www.opentravel.org/OTA/2003/05" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="OTA_LoyaltyCertificateRedemptionRQ">
* <xs:complexType>
* <xs:sequence>
* <xs:element name="RedemptionInfo">
* <xs:complexType>
* <xs:sequence>
* <xs:element name="RedemptionDetail">
* <xs:complexType>
* <xs:attributeGroup ref="ns:LoyaltyCertificateNumberGroup"/>
* <xs:attributeGroup ref="ns:PromotionCodeGroup"/>
* </xs:complexType>
* </xs:element>
* <xs:element type="ns:PersonNameType" name="Customer" minOccurs="0"/>
* <xs:element name="TravelInfos">
* <xs:complexType>
* <xs:sequence>
* <xs:element type="ns:LoyaltyTravelInfoType" name="TravelInfo" maxOccurs="99"/>
* </xs:sequence>
* </xs:complexType>
* </xs:element>
* </xs:sequence>
* </xs:complexType>
* </xs:element>
* </xs:sequence>
* <xs:attributeGroup ref="ns:OTA_PayloadStdAttributes"/>
* </xs:complexType>
* </xs:element>
*
*/
public class CertificateRedemptionRQ
{
private LoyaltyCertificateNumberGroup redemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup;
private PromotionCodeGroup redemptionInfoRedemptionDetailPromotionCodeGroup;
private PersonName redemptionInfoCustomer;
private List redemptionInfoTravelInfoList = new ArrayList();
private OTAPayloadStdAttributes OTAPayloadStdAttributes;
/**
* Get the 'LoyaltyCertificateNumberGroup' attributeGroup value.
*
* @return value
*/
public LoyaltyCertificateNumberGroup getRedemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup() {
return redemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup;
}
/**
* Set the 'LoyaltyCertificateNumberGroup' attributeGroup value.
*
* @param redemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup
*/
public void setRedemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup(
LoyaltyCertificateNumberGroup redemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup) {
this.redemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup = redemptionInfoRedemptionDetailLoyaltyCertificateNumberGroup;
}
/**
* Get the 'PromotionCodeGroup' attributeGroup value.
*
* @return value
*/
public PromotionCodeGroup getRedemptionInfoRedemptionDetailPromotionCodeGroup() {
return redemptionInfoRedemptionDetailPromotionCodeGroup;
}
/**
* Set the 'PromotionCodeGroup' attributeGroup value.
*
* @param redemptionInfoRedemptionDetailPromotionCodeGroup
*/
public void setRedemptionInfoRedemptionDetailPromotionCodeGroup(
PromotionCodeGroup redemptionInfoRedemptionDetailPromotionCodeGroup) {
this.redemptionInfoRedemptionDetailPromotionCodeGroup = redemptionInfoRedemptionDetailPromotionCodeGroup;
}
/**
* Get the 'Customer' element value. Information about the individual associated with the loyalty program.
*
* @return value
*/
public PersonName getRedemptionInfoCustomer() {
return redemptionInfoCustomer;
}
/**
* Set the 'Customer' element value. Information about the individual associated with the loyalty program.
*
* @param redemptionInfoCustomer
*/
public void setRedemptionInfoCustomer(PersonName redemptionInfoCustomer) {
this.redemptionInfoCustomer = redemptionInfoCustomer;
}
/**
* Get the list of 'TravelInfo' element items. Information about the customers trip and loyalty program.
*
* @return list
*/
public List getRedemptionInfoTravelInfos() {
return redemptionInfoTravelInfoList;
}
/**
* Set the list of 'TravelInfo' element items. Information about the customers trip and loyalty program.
*
* @param list
*/
public void setRedemptionInfoTravelInfos(List list) {
redemptionInfoTravelInfoList = list;
}
/**
* Get the number of 'TravelInfo' element items.
* @return count
*/
public int sizeRedemptionInfoTravelInfos() {
return redemptionInfoTravelInfoList.size();
}
/**
* Add a 'TravelInfo' element item.
* @param item
*/
public void addRedemptionInfoTravelInfo(LoyaltyTravelInfo item) {
redemptionInfoTravelInfoList.add(item);
}
/**
* Get 'TravelInfo' element item by position.
* @return item
* @param index
*/
public LoyaltyTravelInfo getRedemptionInfoTravelInfo(int index) {
return redemptionInfoTravelInfoList.get(index);
}
/**
* Remove all 'TravelInfo' element items.
*/
public void clearRedemptionInfoTravelInfos() {
redemptionInfoTravelInfoList.clear();
}
/**
* Get the 'OTA_PayloadStdAttributes' attributeGroup value.
*
* @return value
*/
public OTAPayloadStdAttributes getOTAPayloadStdAttributes() {
return OTAPayloadStdAttributes;
}
/**
* Set the 'OTA_PayloadStdAttributes' attributeGroup value.
*
* @param OTAPayloadStdAttributes
*/
public void setOTAPayloadStdAttributes(
OTAPayloadStdAttributes OTAPayloadStdAttributes) {
this.OTAPayloadStdAttributes = OTAPayloadStdAttributes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy