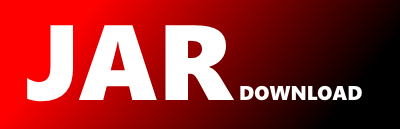
ch.epfl.labos.iu.orm.DBSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
Jinq public API for extending Java 8 streams with database functionality
package ch.epfl.labos.iu.orm;
import java.io.Serializable;
import java.util.Collection;
import org.jinq.orm.stream.JinqStream;
import org.jinq.orm.stream.NonQueryJinqStream;
import org.jinq.tuples.Pair;
public interface DBSet extends Collection
{
public T getValue();
public T get();
public DBSet with(T toAdd);
public DBSet comparisonClone();
public DBSet sortedByIntAscending(final IntSorter sorter);
public DBSet sortedByIntDescending(final IntSorter sorter);
public DBSet sortedByDoubleAscending(final DoubleSorter sorter);
public DBSet sortedByDoubleDescending(final DoubleSorter sorter);
public DBSet sortedByStringAscending(final StringSorter sorter);
public DBSet sortedByStringDescending(final StringSorter sorter);
public DBSet sortedByDateAscending(final DateSorter sorter);
public DBSet sortedByDateDescending(final DateSorter sorter);
public DBSet firstN(int n);
// New stuff for Queryll2
public static interface Where extends Serializable {
public boolean where(U obj);
}
public DBSet where(Where test);
public static interface Select extends Serializable {
public V select(U val);
}
public DBSet select(Select select);
// TODO: Joins are somewhat dangerous because certain types of joins that are
// expressible here are NOT expressible in SQL. (Moving a join into
// a from clause is only possible if the join does not access variables from
// other things in the FROM clause *if* it ends up as a subquery. If we can
// express it as not a subquery, then it's ok.
// TODO: Perhaps only providing a join(DBSet other) is safer because
// I think it will translate into valid SQL code, but it prevents people from
// using navigational queries e.g. customers.join(customer -> customer.getPurchases);
public static interface Join extends Serializable {
public DBSet join(U val);
}
public DBSet> join(Join join);
public static interface AggregateDouble extends Serializable {
public double aggregate(U val);
}
public static interface AggregateInteger extends Serializable {
public int aggregate(U val);
}
public static interface AggregateSelect extends Serializable {
public V aggregateSelect(DBSet val);
}
public double sumDouble(AggregateDouble aggregate);
public int sumInt(AggregateInteger aggregate);
public double maxDouble(AggregateDouble aggregate);
public int maxInt(AggregateInteger aggregate);
public U selectAggregates(AggregateSelect aggregate);
public DBSet unique();
public static interface AggregateGroup extends Serializable {
public V aggregateSelect(W key, DBSet val);
}
public DBSet> group(Select select, AggregateGroup aggregate);
default public JinqStream jinqStream()
{
return new NonQueryJinqStream<>(stream());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy