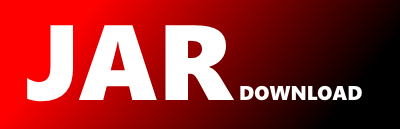
ch.epfl.labos.iu.orm.QueryComposer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
Jinq public API for extending Java 8 streams with database functionality
package ch.epfl.labos.iu.orm;
import java.util.Iterator;
import java.util.function.Consumer;
import java.util.function.Predicate;
import org.jinq.orm.stream.JinqStream;
import org.jinq.tuples.Pair;
import ch.epfl.labos.iu.orm.DBSet.AggregateDouble;
import ch.epfl.labos.iu.orm.DBSet.AggregateGroup;
import ch.epfl.labos.iu.orm.DBSet.AggregateInteger;
import ch.epfl.labos.iu.orm.DBSet.AggregateSelect;
import ch.epfl.labos.iu.orm.DBSet.Join;
import ch.epfl.labos.iu.orm.DBSet.Select;
import ch.epfl.labos.iu.orm.DBSet.Where;
public interface QueryComposer
{
public String getDebugQueryString();
// Actually executes the query and returns the results in an iterator
public Iterator executeAndReturnResultIterator(Consumer exceptionReporter);
// Returns a new query with the given operation integrated in
// (or returns null if the given operation cannot be integrated)
public QueryComposer with(T toAdd);
public QueryComposer sortedByInt(final IntSorter sorter, boolean isAscending);
public QueryComposer sortedByDouble(final DoubleSorter sorter, boolean isAscending);
public QueryComposer sortedByString(final StringSorter sorter, boolean isAscending);
public QueryComposer sortedByDate(final DateSorter sorter, boolean isAscending);
public QueryComposer firstN(int n);
// New stuff for Queryll2
public QueryComposer where(JinqStream.Where test);
public QueryComposer select(JinqStream.Select select);
public QueryComposer> join(JinqStream.Join join);
public QueryComposer unique();
public QueryComposer> group(JinqStream.Select select, JinqStream.AggregateGroup aggregate);
// returns null if the aggregates cannot be calculated
public Double sumDouble(JinqStream.AggregateDouble aggregate);
public Integer sumInt(JinqStream.AggregateInteger aggregate);
public Double maxDouble(JinqStream.AggregateDouble aggregate);
public Integer maxInt(JinqStream.AggregateInteger aggregate);
public U selectAggregates(JinqStream.AggregateSelect aggregate);
public Object[] multiaggregate(JinqStream.AggregateSelect[] aggregates);
public void setHint(String name, Object val);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy