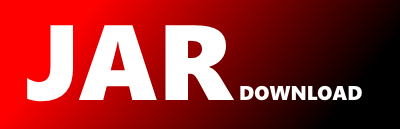
org.jinq.orm.stream.JinqStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
Jinq public API for extending Java 8 streams with database functionality
package org.jinq.orm.stream;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.stream.Stream;
import org.jinq.tuples.Pair;
import org.jinq.tuples.Tuple3;
import ch.epfl.labos.iu.orm.DateSorter;
import ch.epfl.labos.iu.orm.DoubleSorter;
import ch.epfl.labos.iu.orm.IntSorter;
import ch.epfl.labos.iu.orm.StringSorter;
public interface JinqStream extends Stream
{
public static interface Where extends Serializable {
public boolean where(U obj) throws E;
}
public JinqStream where(Where test);
public static interface Select extends Serializable {
public V select(U val);
}
public JinqStream select(Select select);
// TODO: Joins are somewhat dangerous because certain types of joins that are
// expressible here are NOT expressible in SQL. (Moving a join into
// a from clause is only possible if the join does not access variables from
// other things in the FROM clause *if* it ends up as a subquery. If we can
// express it as not a subquery, then it's ok.
// TODO: Perhaps only providing a join(DBSet other) is safer because
// I think it will translate into valid SQL code, but it prevents people from
// using navigational queries e.g. customers.join(customer -> customer.getPurchases);
public static interface Join extends Serializable {
public JinqStream join(U val);
}
// TODO: Rewrite join so that it doesn't take a DBSet
public JinqStream> join(Join join);
public JinqStream unique();
public static interface AggregateGroup extends Serializable {
public V aggregateSelect(W key, JinqStream val);
}
public JinqStream> group(Select select, AggregateGroup aggregate);
public static interface AggregateDouble extends Serializable {
public double aggregate(U val);
}
public static interface AggregateInteger extends Serializable {
public int aggregate(U val);
}
public static interface AggregateSelect extends Serializable {
public V aggregateSelect(JinqStream val);
}
public double sumDouble(AggregateDouble aggregate);
public int sumInt(AggregateInteger aggregate);
public double maxDouble(AggregateDouble aggregate);
public int maxInt(AggregateInteger aggregate);
public U selectAggregates(AggregateSelect aggregate);
public Pair aggregate(AggregateSelect aggregate1,
AggregateSelect aggregate2);
public Tuple3 aggregate(AggregateSelect aggregate1,
AggregateSelect aggregate2, AggregateSelect aggregate3);
public JinqStream sortedByIntAscending(final IntSorter sorter);
public JinqStream sortedByIntDescending(final IntSorter sorter);
public JinqStream sortedByDoubleAscending(final DoubleSorter sorter);
public JinqStream sortedByDoubleDescending(final DoubleSorter sorter);
public JinqStream sortedByStringAscending(final StringSorter sorter);
public JinqStream sortedByStringDescending(final StringSorter sorter);
public JinqStream sortedByDateAscending(final DateSorter sorter);
public JinqStream sortedByDateDescending(final DateSorter sorter);
public JinqStream firstN(int n);
public T getOnlyValue();
public JinqStream with(T toAdd);
// TODO: Should toList() throw an exception?
public List toList();
public String getDebugQueryString();
/**
* Used for recording an exception that occurred during processing
* somewhere in the stream chain.
*
* @param source lambda object that caused the exception (used so that
* if the same lambda causes multiple exceptions, only some of them
* need to be recorded in order to avoid memory issues)
* @param exception actual exception object
*/
public void propagateException(Object source, Throwable exception);
public Collection getExceptions();
/**
* Sets a hint on the stream for how the query should be executed
* @param name
* @param value
* @return this
*/
public JinqStream setHint(String name, Object value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy