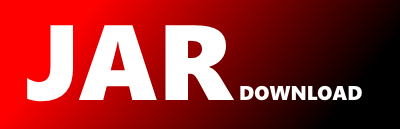
ch.epfl.labos.iu.orm.LazySet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
Jinq public API for extending Java 8 streams with database functionality
package ch.epfl.labos.iu.orm;
import java.util.Iterator;
public class LazySet extends BaseSet
{
LazySet listener;
protected VectorSet data;
public DBSet comparisonClone()
{
if (data != null)
return data.comparisonClone();
LazySet copy = new LazySet();
return copy;
}
public Object clone() throws CloneNotSupportedException
{
LazySet copy = (LazySet)super.clone();
if (data != null)
copy.data = (VectorSet)data.clone();
return copy;
}
public LazySet()
{
data = null;
}
public LazySet(boolean isEmpty)
{
if (isEmpty)
data = new VectorSet();
else
data = null;
}
public void setRealizeListener(LazySet listener)
{
this.listener = listener;
}
public void realize()
{
if (data == null)
{
data = createRealizedSet();
if (listener != null)
{
try {
listener.data = (VectorSet)data.clone();
} catch (CloneNotSupportedException e)
{
e.printStackTrace();
}
}
}
}
protected VectorSet createRealizedSet()
{
return null;
}
public int size()
{
realize();
return data.size();
}
public Iterator iterator()
{
Iterator lazyIterator = new LazyIterator(this);
return lazyIterator;
}
public boolean add(T o)
{
realize();
return data.add(o);
}
public boolean remove(Object o)
{
realize();
return data.remove(o);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy