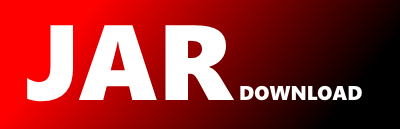
org.jinq.jpa.jpqlquery.JPQLQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jinq-jpa Show documentation
Show all versions of jinq-jpa Show documentation
Jinq functional-style queries for JPA
package org.jinq.jpa.jpqlquery;
import java.util.List;
/**
* Data structure used to represent JPQL queries and the conversions
* needed to parse results into a form usable by Jinq.
*/
public abstract class JPQLQuery implements JPQLFragment
{
public static JPQLQuery findAllEntities(String entityName)
{
SelectFromWhere query = new SelectFromWhere<>();
From from = From.forEntity(entityName);
query.cols = ColumnExpressions.singleColumn(
new SimpleRowReader<>(), new FromAliasExpression(from));
query.froms.add(from);
return query;
}
public JPQLQuery()
{
}
/**
* @return true iff the query is a simple select...from...where style query
*/
public abstract boolean isSelectFromWhere();
public abstract boolean isSelectOnly();
public abstract boolean isSelectFromWhereGroupHaving();
public abstract boolean canSelectWhere();
public abstract boolean canSelectHaving();
public abstract boolean canAggregate();
public abstract boolean canUnsortAggregate();
public abstract boolean canSort();
public abstract boolean canDistinct();
public abstract boolean isValidSubquery();
public abstract String getQueryString();
public abstract List getQueryParameters();
public abstract JPQLQuery shallowCopy();
public abstract RowReader getRowReader();
// TODO: If I code things carefully, maybe this method isn't needed.
// public JPQLQuery copy()
// {
// JPQLQuery newQuery = new JPQLQuery<>();
// newQuery.query = query;
// return newQuery;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy