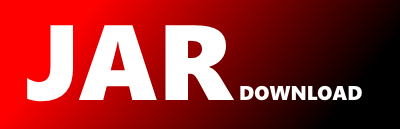
sql.create_db_manager.xslt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orm Show documentation
Show all versions of orm Show documentation
Jinq legacy ORM for generating entity classes from XML
The newest version!
package ;
import java.sql.DriverManager;
import java.sql.Connection;
import java.sql.SQLException;
import java.lang.reflect.Method;
import java.io.PrintWriter;
import ch.epfl.labos.iu.orm.query2.SQLQueryTransforms;
import ch.epfl.labos.iu.orm.queryll2.runtime.QueryllEntityConfigurationInfo;
import ch.epfl.labos.iu.orm.queryll2.runtime.ConfigureQueryll;
import ch.epfl.labos.iu.orm.queryll2.runtime.ORMEntity;
import ch.epfl.labos.iu.orm.queryll2.runtime.ORMField;
public class DBManager implements ConfigureQueryll
{
public PrintWriter testOut = null; // When non-null, we're in test mode
public Connection con = null;
public boolean isQueryOnly = false;
public boolean usePartialQueryCaching = true;
public boolean useFullQueryCaching = true;
EntityManager cachedEntityManager;
public EntityManager begin()
{
if (con != null)
{
if (!isQueryOnly)
{
try {
con.setAutoCommit(false);
} catch (SQLException e)
{
e.printStackTrace();
}
}
}
if (isQueryOnly && cachedEntityManager != null)
{
EntityManager toReturn = cachedEntityManager;
cachedEntityManager = null;
return toReturn;
}
return new EntityManager(this);
}
public void end(EntityManager em, boolean commit)
{
try {
if (!isQueryOnly)
{
if (commit)
{
em.flushDirty();
if (con != null) con.commit();
}
else
{
if (con != null) con.rollback();
}
if (con != null) con.setAutoCommit(true);
}
else
cachedEntityManager = em;
} catch (SQLException e)
{
e.printStackTrace();
}
}
public void doTransaction(Transaction transaction)
{
EntityManager em = begin();
end(em, transaction.execute(em));
}
public static interface Transaction
{
// returns true to commit the changes
public boolean execute(EntityManager em);
}
public void close()
{
try {
if (con != null) con.close();
} catch (SQLException e)
{
e.printStackTrace();
}
}
public void configureQueryll(QueryllEntityConfigurationInfo config)
{
}
SQLQueryTransforms queryll; // Place for storing opaque queryll information
public void storeQueryllAnalysisResults(SQLQueryTransforms queryllAnalysis)
{
this.queryll = queryllAnalysis;
}
// Creates a debug DBManager that displays queries directly to output
// and does not start or query a real database
//
public DBManager(PrintWriter output)
{
this(output, null, null, false);
}
public DBManager(boolean logQueries)
{
this(null, " ", " ", logQueries);
}
public DBManager()
{
this(true);
}
public DBManager(PrintWriter output, String jdbcDriver, String jdbcPath, boolean logQueries)
{
testOut = output;
if (jdbcPath != null)
{
try {
if (jdbcDriver != null)
Class.forName(jdbcDriver);
con = DriverManager.getConnection(
jdbcPath/*, "APP", "APP"*/);
if (logQueries)
{
con = (Connection)Class.forName("ch.epfl.labos.iu.orm.trace.LoggedConnection")
.getConstructor(Class.forName("java.sql.Connection"))
.newInstance(con);
}
} catch(Exception e)
{
e.printStackTrace();
}
try
{
Method m = java.lang.Class.forName(" .OptimizationBackdoor").getMethod("initializeOptimizations", new Class[0]);
m.invoke(null, new Object[0]);
} catch (Exception e) {}
}
}
public int timeout = 0;
}
config.registerORMEntity(new ORMEntity(
" ",
" ",
" ",
new ORMField[] {
}));
new ORMField(" ",
" ",
" ",
false ,
false
),
config.registerORMSimpleLink(
" ",
" ",
" ",
" ",
" ",
" ",
" ",
" ");
config.registerORMNMLink(
" ",
" ",
" ",
" ",
" ",
" ",
" ",
" ",
" ",
" ");
© 2015 - 2025 Weber Informatics LLC | Privacy Policy