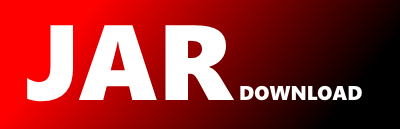
sql.create_entity.xslt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orm Show documentation
Show all versions of orm Show documentation
Jinq legacy ORM for generating entity classes from XML
The newest version!
package ;
import ch.epfl.labos.iu.orm.DBSet;
import ch.epfl.labos.iu.orm.VectorSet;
import ch.epfl.labos.iu.orm.LazySet;
import org.jinq.tuples.Pair;
import org.jinq.tuples.Tuple3;
import org.jinq.tuples.Tuple4;
import org.jinq.tuples.Tuple5;
import org.jinq.orm.annotations.NoSideEffects;
import java.sql.Date;
import java.sql.ResultSet;
import java.sql.Statement;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class implements Cloneable
{
EntityManager em;
public ()
{
}
public void persist(EntityManager em)
{
this.em = em;
idKey = ;
em.newInstance(this);
}
public void markAsDirty()
{
if (em != null)
{
em.dirtyInstance(this);
// TODO: extend this to sets
}
}
public void dispose()
{
if (em != null)
em.dispose(this);
em = null;
}
public (EntityManager em, ResultSet rs, int column) throws SQLException
{
this.em = em;
idKey = ;
}
public (EntityManager em, ResultSet rs, String prefix) throws SQLException
{
this.em = em;
idKey = ;
}
idKey;
public idKey()
{
return idKey;
}
comparisonCopy;
public copyForComparison()
{
if (comparisonCopy != null) return comparisonCopy;
copy = new ();
copy. = ;
comparisonCopy = copy;
return copy;
}
public Object clone() throws CloneNotSupportedException
{
// TODO: This is bogus (doesn't handle sets correctly)
return super.clone();
}
}
private ;
public get ()
{
return ;
}
public void set ( _val)
{
if (em != null)
em.dirtyInstance(this);
= _val;
}
= rs. (prefix + "_ ");
= rs. (column);
column++;
class Set extends LazySet< >
{
protected VectorSet< > createRealizedSet()
{
VectorSet< > newset = new VectorSet< >();
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ ", "
+ " FROM as A, AS C"
+ " WHERE 1=1"
+ " AND A. = ?"
+ " AND A. = C. "
);
int idx = 0;
idx++;
stmt.setObject(idx, );
ResultSet rs = stmt.executeQuery();
while ( rs.next() ) {
newset.add(em.create (rs, "C"));
}
rs.close();
stmt.close();
} catch(SQLException e)
{
e.printStackTrace();
}
return newset;
}
public Set(boolean isEmpty)
{
super(isEmpty);
}
public boolean add( _val)
{
super.add(_val);
if (em != null)
em.dirtyInstance( .this);
_val.set ( .this);
return true;
}
public boolean remove( _val)
{
super.remove(_val);
if (em != null)
em.dirtyInstance( .this);
_val.set (null);
return true;
}
}
DBSet< > ;
public DBSet< > get ()
{
return ;
}
public void set (DBSet< > _val)
{
= _val;
}
boolean is Realized;
String _linkcol_ _ ;
;
public get ()
{
if (!is Realized)
{
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ " FROM as C"
+ " WHERE 1=1"
+ " AND C. = " + _linkcol_ _
);
ResultSet rs = stmt.executeQuery();
if (rs.next())
= em.create (rs, "C");
rs.close();
stmt.close();
is Realized = true;
} catch(SQLException e)
{
e.printStackTrace();
}
}
return ;
}
public void set ( _val)
{
if (em != null)
em.dirtyInstance(this);
= _val;
is Realized = true;
}
boolean is Realized;
String _linkcol_ _ ;
;
public get ()
{
if (!is Realized)
{
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ " FROM as C"
+ " WHERE 1=1"
+ " AND C. = " + _linkcol_ _
);
ResultSet rs = stmt.executeQuery();
if (rs.next())
= em.create (rs, "C");
rs.close();
stmt.close();
is Realized = true;
} catch(SQLException e)
{
e.printStackTrace();
}
}
return ;
}
public void set ( _val)
{
if (em != null)
em.dirtyInstance(this);
if (get () != null)
.get ().remove(this);
= _val;
_val.get ().add(this);
}
class Set extends LazySet< >
{
protected VectorSet< > createRealizedSet()
{
VectorSet< > newset = new VectorSet< >();
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ ", "
+ " FROM as A, AS C"
+ " WHERE 1=1"
+ " AND A. = ? "
+ " AND A. = C. "
);
int idx = 0;
idx++;
stmt.setObject(idx, );
ResultSet rs = stmt.executeQuery();
while ( rs.next() ) {
newset.add(em.create (rs, "C"));
}
rs.close();
stmt.close();
} catch(SQLException e)
{
e.printStackTrace();
}
return newset;
}
public Set(boolean isEmpty)
{
super(isEmpty);
}
public boolean add( _val)
{
if (em != null)
em.dirtyInstance( .this);
return super.add(_val);
}
public boolean remove( _val)
{
if (em != null)
em.dirtyInstance( .this);
return super.remove(_val);
}
}
DBSet< > ;
public DBSet< > get ()
{
return ;
}
public void set (DBSet< > _val)
{
= _val;
}
boolean is Realized;
String _linkcol_ _ ;
;
public get ()
{
if (!is Realized)
{
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ " FROM as C"
+ " WHERE 1=1"
+ " AND C. = " + _linkcol_ _
);
ResultSet rs = stmt.executeQuery();
if (rs.next())
= em.create (rs, "C");
rs.close();
stmt.close();
is Realized = true;
} catch(SQLException e)
{
e.printStackTrace();
}
}
return ;
}
public void set ( _val)
{
if (em != null)
em.dirtyInstance(this);
if (get () != null)
.set (null);
= _val;
_val.set (this);
}
boolean is Realized;
String _linkcol_ _ ;
;
public get ()
{
if (!is Realized)
{
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ " FROM as C"
+ " WHERE 1=1"
+ " AND C. = " + _linkcol_ _
);
ResultSet rs = stmt.executeQuery();
if (rs.next())
= em.create (rs, "C");
rs.close();
stmt.close();
is Realized = true;
} catch(SQLException e)
{
e.printStackTrace();
}
}
return ;
}
public void set ( _val)
{
if (em != null)
em.dirtyInstance(this);
is Realized = true;
= _val;
}
class Set extends LazySet< >
{
protected VectorSet< > createRealizedSet()
{
VectorSet< > newset = new VectorSet< >();
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ ", "
+ " FROM as A, AS C, as B"
+ " WHERE 1=1"
+ " AND A. = ? "
+ " AND A. = C. "
+ " AND A. = B. "
+ " AND B. = C. "
);
int idx = 0;
idx++;
stmt.setObject(idx, );
ResultSet rs = stmt.executeQuery();
while ( rs.next() ) {
newset.add(em.create (rs, "C"));
}
rs.close();
stmt.close();
} catch(SQLException e)
{
e.printStackTrace();
}
return newset;
}
public Set(boolean isEmpty)
{
super(isEmpty);
}
public boolean add( _val)
{
if (em != null)
em.dirtyInstance( .this);
super.add(_val);
_val.get ().add( .this);
return true;
}
public boolean remove( _val)
{
if (em != null)
em.dirtyInstance( .this);
super.remove(_val);
_val.get ().remove( .this);
return true;
}
}
DBSet< > ;
public DBSet< > get ()
{
return ;
}
public void set (DBSet< > _val)
{
= _val;
}
class Set extends LazySet< >
{
protected VectorSet< > createRealizedSet()
{
VectorSet< > newset = new VectorSet< >();
try {
PreparedStatement stmt = em.db.con.prepareStatement("SELECT "
+ " "
+ ", "
+ " FROM as A, AS C, as B "
+ " WHERE 1=1"
+ " AND A. = ? "
+ " AND A. = C. "
+ " AND B. = C. "
+ " AND A. = B. "
);
int idx = 0;
idx++;
stmt.setObject(idx, );
ResultSet rs = stmt.executeQuery();
while ( rs.next() ) {
newset.add(em.create (rs, "C"));
}
rs.close();
stmt.close();
} catch(SQLException e)
{
e.printStackTrace();
}
return newset;
}
public Set(boolean isEmpty)
{
super(isEmpty);
}
public boolean add( _val)
{
if (em != null)
em.dirtyInstance( .this);
return super.add(_val);
}
public boolean remove( _val)
{
if (em != null)
em.dirtyInstance( .this);
return super.remove(_val);
}
}
DBSet< > ;
public DBSet< > get ()
{
return ;
}
public void set (DBSet< > _val)
{
= _val;
}
get ().clear();
if (get () != null)
get ().get ().remove(this);
set (null);
for ( obj: get ())
obj.set (null);
set (null);
if (get () != null)
get ().set (null);
get ().clear();
for ( obj: get ())
obj.get ().remove(this);
copy. = .comparisonClone();
if ( instanceof LazySet && copy. instanceof LazySet)
{
((LazySet< >) ).setRealizeListener((LazySet< >)copy. );
}
copy. = .comparisonClone();
if ( instanceof LazySet)
{
((LazySet< >) ).setRealizeListener((LazySet< >)copy. );
}
copy. = .comparisonClone();
if ( instanceof LazySet)
{
((LazySet< >) ).setRealizeListener((LazySet< >)copy. );
}
copy. = .comparisonClone();
if ( instanceof LazySet)
{
((LazySet< >) ).setRealizeListener((LazySet< >)copy. );
}
= new Set(true);
is Realized = true;
is Realized = true;
= new Set(true);
is Realized = true;
is Realized = true;
= new Set(true);
= new Set(true);
= new Set(false);
is Realized = false;
_linkcol_ _ = rs.getString("LINK_" + prefix + "_ ");
is Realized = false;
_linkcol_ _ = rs.getString("LINK_" + prefix + "_ ");
= new Set(false);
is Realized = false;
_linkcol_ _ = rs.getString("LINK_" + prefix + "_ ");
is Realized = false;
_linkcol_ _ = rs.getString("LINK_" + prefix + "_ ");
= new Set(false);
= new Set(false);
= new Set(false);
is Realized = false;
_linkcol_ _ = rs.getString(column);
column++;
is Realized = false;
_linkcol_ _ = rs.getString(column);
column++;
= new Set(false);
is Realized = false;
_linkcol_ _ = rs.getString(column);
column++;
is Realized = false;
_linkcol_ _ = rs.getString(column);
column++;
= new Set(false);
= new Set(false);
NoKey
new Pair<
,
>(
,
)
new Tuple3<
,
>(
,
)
new Tuple4<
,
>(
,
)
new Tuple5<
,
>(
,
)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy