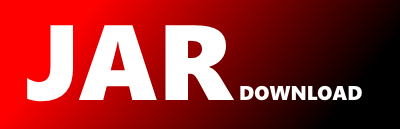
org.jitsi.impl.neomedia.protocol.PullBufferStreamAdapter Maven / Gradle / Ivy
/*
* Copyright @ 2015 Atlassian Pty Ltd
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jitsi.impl.neomedia.protocol;
import java.io.*;
import javax.media.*;
import javax.media.format.*;
import javax.media.protocol.*;
/**
* Represents a PullBufferStream which reads its data from a
* specific PullSourceStream.
*
* @author Lyubomir Marinov
*/
public class PullBufferStreamAdapter
extends BufferStreamAdapter
implements PullBufferStream
{
/**
* Initializes a new PullBufferStreamAdapter instance which
* reads its data from a specific PullSourceStream with a
* specific Format
*
* @param stream the PullSourceStream the new instance is to
* read its data from
* @param format the Format of the specified input
* stream and of the new instance
*/
public PullBufferStreamAdapter(PullSourceStream stream, Format format)
{
super(stream, format);
}
/**
* Gets the frame size measured in bytes defined by a specific
* Format.
*
* @param format the Format to determine the frame size in
* bytes of
* @return the frame size measured in bytes defined by the specified
* Format
*/
private static int getFrameSizeInBytes(Format format)
{
AudioFormat audioFormat = (AudioFormat) format;
int frameSizeInBits = audioFormat.getFrameSizeInBits();
if (frameSizeInBits <= 0)
return
(audioFormat.getSampleSizeInBits() / 8)
* audioFormat.getChannels();
return (frameSizeInBits <= 8) ? 1 : (frameSizeInBits / 8);
}
/**
* Implements PullBufferStream#read(Buffer). Delegates to the wrapped
* PullSourceStream by either allocating a new byte[] buffer or using the
* existing one in the specified Buffer.
*
* @param buffer Buffer to read
* @throws IOException if I/O errors occurred during read operation
*/
public void read(Buffer buffer)
throws IOException
{
Object data = buffer.getData();
byte[] bytes = null;
if (data != null)
{
if (data instanceof byte[])
{
bytes = (byte[]) data;
}
else if (data instanceof short[])
{
short[] shorts = (short[]) data;
bytes = new byte[2 * shorts.length];
}
else if (data instanceof int[])
{
int[] ints = (int[]) data;
bytes = new byte[4 * ints.length];
}
}
if (bytes == null)
{
int frameSizeInBytes = getFrameSizeInBytes(getFormat());
bytes
= new byte[
1024
* ((frameSizeInBytes <= 0) ? 4 : frameSizeInBytes)];
}
read(buffer, bytes, 0, bytes.length);
}
/**
* Implements BufferStreamAdapter#doRead(Buffer, byte[], int, int).
* Delegates to the wrapped PullSourceStream.
*
* @param buffer
* @param data byte array to read
* @param offset to start reading
* @param length length to read
* @return number of bytes read
* @throws IOException if I/O related errors occurred during read operation
*/
@Override
protected int doRead(Buffer buffer, byte[] data, int offset, int length)
throws IOException
{
return stream.read(data, offset, length);
}
/**
* Implements PullBufferStream#willReadBlock(). Delegates to the wrapped
* PullSourceStream.
*
* @return true if this stream will block on read operation, false otherwise
*/
public boolean willReadBlock()
{
return stream.willReadBlock();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy