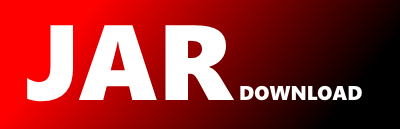
org.jitsi.service.neomedia.VideoMediaStream Maven / Gradle / Ivy
/*
* Copyright @ 2015 Atlassian Pty Ltd
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jitsi.service.neomedia;
import java.awt.*;
import java.util.*;
import java.util.List;
import org.jitsi.service.neomedia.control.*;
import org.jitsi.service.neomedia.rtp.*;
import org.jitsi.util.event.*;
/**
* Extends the MediaStream interface and adds methods specific to
* video streaming.
*
* @author Emil Ivov
* @author Lyubomir Marinov
*/
public interface VideoMediaStream
extends MediaStream
{
/**
* The name of the property used to control whether {@link VideoMediaStream}
* should request retransmissions for lost RTP packets using RTCP NACK.
*/
String REQUEST_RETRANSMISSIONS_PNAME
= VideoMediaStream.class.getName() + ".REQUEST_RETRANSMISSIONS";
/**
* Adds a specific VideoListener to this VideoMediaStream
* in order to receive notifications when visual/video Components
* are being added and removed.
*
* Adding a listener which has already been added does nothing i.e. it is
* not added more than once and thus does not receive one and the same
* VideoEvent multiple times
*
* @param listener the VideoListener to be notified when
* visual/video Components are being added or removed in this
* VideoMediaStream
*/
public void addVideoListener(VideoListener listener);
/**
* Gets the KeyFrameControl of this VideoMediaStream.
*
* @return the KeyFrameControl of this VideoMediaStream
*/
public KeyFrameControl getKeyFrameControl();
/**
* Gets the visual Component, if any, depicting the video streamed
* from the local peer to the remote peer.
*
* @return the visual Component depicting the local video if local
* video is actually being streamed from the local peer to the remote peer;
* otherwise, null
*/
public Component getLocalVisualComponent();
/**
* Gets the QualityControl of this VideoMediaStream.
*
* @return the QualityControl of this VideoMediaStream
*/
public QualityControl getQualityControl();
/**
* Gets the visual Component where video from the remote peer is
* being rendered or null if no video is currently being rendered.
*
* @return the visual Component where video from the remote peer is
* being rendered or null if no video is currently being rendered
* @deprecated Since multiple videos may be received from the remote peer
* and rendered, it is not clear which one of them is to be singled out as
* the return value. Thus {@link #getVisualComponent(long)} and
* {@link #getVisualComponents()} are to be used instead.
*/
@Deprecated
public Component getVisualComponent();
/**
* Gets the visual Component rendering the ReceiveStream
* with a specific SSRC.
*
* @param ssrc the SSRC of the ReceiveStream to get the associated
* rendering visual Component of
* @return the visual Component rendering the
* ReceiveStream with the specified ssrc if any;
* otherwise, null
*/
public Component getVisualComponent(long ssrc);
/**
* Gets a list of the visual Components where video from the remote
* peer is being rendered.
*
* @return a list of the visual Components where video from the
* remote peer is being rendered
*/
public List getVisualComponents();
/**
* Move origin of a partial desktop streaming MediaDevice.
*
* @param x new x coordinate origin
* @param y new y coordinate origin
*/
public void movePartialDesktopStreaming(int x, int y);
/**
* Removes a specific VideoListener from this
* VideoMediaStream in order to have to no longer receive
* notifications when visual/video Components are being added and
* removed.
*
* @param listener the VideoListener to no longer be notified when
* visual/video Components are being added or removed in this
* VideoMediaStream
*/
public void removeVideoListener(VideoListener listener);
/**
* Updates the QualityControl of this VideoMediaStream.
*
* @param advancedParams parameters of advanced attributes that may affect
* quality control
*/
public void updateQualityControl(Map advancedParams);
/**
* Creates an instance of {@link BandwidthEstimator} for this
* {@link MediaStream} if one doesn't already exist. Returns the instance.
*/
public BandwidthEstimator getOrCreateBandwidthEstimator();
}