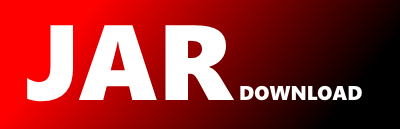
org.jlab.jaws.entity.AlarmClass Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.jlab.jaws.entity;
import org.apache.avro.generic.GenericArray;
import org.apache.avro.specific.SpecificData;
import org.apache.avro.util.Utf8;
import org.apache.avro.message.BinaryMessageEncoder;
import org.apache.avro.message.BinaryMessageDecoder;
import org.apache.avro.message.SchemaStore;
/** An alarm class */
@org.apache.avro.specific.AvroGenerated
public class AlarmClass extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = -6728679526053417282L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"AlarmClass\",\"namespace\":\"org.jlab.jaws.entity\",\"doc\":\"An alarm class\",\"fields\":[{\"name\":\"category\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"The alarm category\"},{\"name\":\"priority\",\"type\":{\"type\":\"enum\",\"name\":\"AlarmPriority\",\"doc\":\"Enumeration of possible priorities; useful for operators to know which alarms to handle first\",\"symbols\":[\"P1_CRITICAL\",\"P2_MAJOR\",\"P3_MINOR\",\"P4_INCIDENTAL\"]},\"doc\":\"The alarm priority\"},{\"name\":\"rationale\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Markdown formatted text describing the reason this alarm is necessary\"},{\"name\":\"correctiveaction\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Markdown formatted text describing the corrective action to take when the alarm becomes active\"},{\"name\":\"pointofcontactusername\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)\"},{\"name\":\"latchable\",\"type\":\"boolean\",\"doc\":\"Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement\",\"default\":false},{\"name\":\"filterable\",\"type\":\"boolean\",\"doc\":\"Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.\",\"default\":true},{\"name\":\"ondelayseconds\",\"type\":[\"null\",\"long\"],\"doc\":\"The number of seconds of on-delay\"},{\"name\":\"offdelayseconds\",\"type\":[\"null\",\"long\"],\"doc\":\"The number of seconds of off-delay\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private static final SpecificData MODEL$ = new SpecificData();
private static final BinaryMessageEncoder ENCODER =
new BinaryMessageEncoder<>(MODEL$, SCHEMA$);
private static final BinaryMessageDecoder DECODER =
new BinaryMessageDecoder<>(MODEL$, SCHEMA$);
/**
* Return the BinaryMessageEncoder instance used by this class.
* @return the message encoder used by this class
*/
public static BinaryMessageEncoder getEncoder() {
return ENCODER;
}
/**
* Return the BinaryMessageDecoder instance used by this class.
* @return the message decoder used by this class
*/
public static BinaryMessageDecoder getDecoder() {
return DECODER;
}
/**
* Create a new BinaryMessageDecoder instance for this class that uses the specified {@link SchemaStore}.
* @param resolver a {@link SchemaStore} used to find schemas by fingerprint
* @return a BinaryMessageDecoder instance for this class backed by the given SchemaStore
*/
public static BinaryMessageDecoder createDecoder(SchemaStore resolver) {
return new BinaryMessageDecoder<>(MODEL$, SCHEMA$, resolver);
}
/**
* Serializes this AlarmClass to a ByteBuffer.
* @return a buffer holding the serialized data for this instance
* @throws java.io.IOException if this instance could not be serialized
*/
public java.nio.ByteBuffer toByteBuffer() throws java.io.IOException {
return ENCODER.encode(this);
}
/**
* Deserializes a AlarmClass from a ByteBuffer.
* @param b a byte buffer holding serialized data for an instance of this class
* @return a AlarmClass instance decoded from the given buffer
* @throws java.io.IOException if the given bytes could not be deserialized into an instance of this class
*/
public static AlarmClass fromByteBuffer(
java.nio.ByteBuffer b) throws java.io.IOException {
return DECODER.decode(b);
}
/** The alarm category */
private java.lang.String category;
/** The alarm priority */
private org.jlab.jaws.entity.AlarmPriority priority;
/** Markdown formatted text describing the reason this alarm is necessary */
private java.lang.String rationale;
/** Markdown formatted text describing the corrective action to take when the alarm becomes active */
private java.lang.String correctiveaction;
/** Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example) */
private java.lang.String pointofcontactusername;
/** Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement */
private boolean latchable;
/** Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program. */
private boolean filterable;
/** The number of seconds of on-delay */
private java.lang.Long ondelayseconds;
/** The number of seconds of off-delay */
private java.lang.Long offdelayseconds;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public AlarmClass() {}
/**
* All-args constructor.
* @param category The alarm category
* @param priority The alarm priority
* @param rationale Markdown formatted text describing the reason this alarm is necessary
* @param correctiveaction Markdown formatted text describing the corrective action to take when the alarm becomes active
* @param pointofcontactusername Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
* @param latchable Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
* @param filterable Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
* @param ondelayseconds The number of seconds of on-delay
* @param offdelayseconds The number of seconds of off-delay
*/
public AlarmClass(java.lang.String category, org.jlab.jaws.entity.AlarmPriority priority, java.lang.String rationale, java.lang.String correctiveaction, java.lang.String pointofcontactusername, java.lang.Boolean latchable, java.lang.Boolean filterable, java.lang.Long ondelayseconds, java.lang.Long offdelayseconds) {
this.category = category;
this.priority = priority;
this.rationale = rationale;
this.correctiveaction = correctiveaction;
this.pointofcontactusername = pointofcontactusername;
this.latchable = latchable;
this.filterable = filterable;
this.ondelayseconds = ondelayseconds;
this.offdelayseconds = offdelayseconds;
}
@Override
public org.apache.avro.specific.SpecificData getSpecificData() { return MODEL$; }
@Override
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
@Override
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return category;
case 1: return priority;
case 2: return rationale;
case 3: return correctiveaction;
case 4: return pointofcontactusername;
case 5: return latchable;
case 6: return filterable;
case 7: return ondelayseconds;
case 8: return offdelayseconds;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
// Used by DatumReader. Applications should not call.
@Override
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: category = value$ != null ? value$.toString() : null; break;
case 1: priority = (org.jlab.jaws.entity.AlarmPriority)value$; break;
case 2: rationale = value$ != null ? value$.toString() : null; break;
case 3: correctiveaction = value$ != null ? value$.toString() : null; break;
case 4: pointofcontactusername = value$ != null ? value$.toString() : null; break;
case 5: latchable = (java.lang.Boolean)value$; break;
case 6: filterable = (java.lang.Boolean)value$; break;
case 7: ondelayseconds = (java.lang.Long)value$; break;
case 8: offdelayseconds = (java.lang.Long)value$; break;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
/**
* Gets the value of the 'category' field.
* @return The alarm category
*/
public java.lang.String getCategory() {
return category;
}
/**
* Sets the value of the 'category' field.
* The alarm category
* @param value the value to set.
*/
public void setCategory(java.lang.String value) {
this.category = value;
}
/**
* Gets the value of the 'priority' field.
* @return The alarm priority
*/
public org.jlab.jaws.entity.AlarmPriority getPriority() {
return priority;
}
/**
* Sets the value of the 'priority' field.
* The alarm priority
* @param value the value to set.
*/
public void setPriority(org.jlab.jaws.entity.AlarmPriority value) {
this.priority = value;
}
/**
* Gets the value of the 'rationale' field.
* @return Markdown formatted text describing the reason this alarm is necessary
*/
public java.lang.String getRationale() {
return rationale;
}
/**
* Sets the value of the 'rationale' field.
* Markdown formatted text describing the reason this alarm is necessary
* @param value the value to set.
*/
public void setRationale(java.lang.String value) {
this.rationale = value;
}
/**
* Gets the value of the 'correctiveaction' field.
* @return Markdown formatted text describing the corrective action to take when the alarm becomes active
*/
public java.lang.String getCorrectiveaction() {
return correctiveaction;
}
/**
* Sets the value of the 'correctiveaction' field.
* Markdown formatted text describing the corrective action to take when the alarm becomes active
* @param value the value to set.
*/
public void setCorrectiveaction(java.lang.String value) {
this.correctiveaction = value;
}
/**
* Gets the value of the 'pointofcontactusername' field.
* @return Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
*/
public java.lang.String getPointofcontactusername() {
return pointofcontactusername;
}
/**
* Sets the value of the 'pointofcontactusername' field.
* Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
* @param value the value to set.
*/
public void setPointofcontactusername(java.lang.String value) {
this.pointofcontactusername = value;
}
/**
* Gets the value of the 'latchable' field.
* @return Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
*/
public boolean getLatchable() {
return latchable;
}
/**
* Sets the value of the 'latchable' field.
* Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
* @param value the value to set.
*/
public void setLatchable(boolean value) {
this.latchable = value;
}
/**
* Gets the value of the 'filterable' field.
* @return Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
*/
public boolean getFilterable() {
return filterable;
}
/**
* Sets the value of the 'filterable' field.
* Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
* @param value the value to set.
*/
public void setFilterable(boolean value) {
this.filterable = value;
}
/**
* Gets the value of the 'ondelayseconds' field.
* @return The number of seconds of on-delay
*/
public java.lang.Long getOndelayseconds() {
return ondelayseconds;
}
/**
* Sets the value of the 'ondelayseconds' field.
* The number of seconds of on-delay
* @param value the value to set.
*/
public void setOndelayseconds(java.lang.Long value) {
this.ondelayseconds = value;
}
/**
* Gets the value of the 'offdelayseconds' field.
* @return The number of seconds of off-delay
*/
public java.lang.Long getOffdelayseconds() {
return offdelayseconds;
}
/**
* Sets the value of the 'offdelayseconds' field.
* The number of seconds of off-delay
* @param value the value to set.
*/
public void setOffdelayseconds(java.lang.Long value) {
this.offdelayseconds = value;
}
/**
* Creates a new AlarmClass RecordBuilder.
* @return A new AlarmClass RecordBuilder
*/
public static org.jlab.jaws.entity.AlarmClass.Builder newBuilder() {
return new org.jlab.jaws.entity.AlarmClass.Builder();
}
/**
* Creates a new AlarmClass RecordBuilder by copying an existing Builder.
* @param other The existing builder to copy.
* @return A new AlarmClass RecordBuilder
*/
public static org.jlab.jaws.entity.AlarmClass.Builder newBuilder(org.jlab.jaws.entity.AlarmClass.Builder other) {
if (other == null) {
return new org.jlab.jaws.entity.AlarmClass.Builder();
} else {
return new org.jlab.jaws.entity.AlarmClass.Builder(other);
}
}
/**
* Creates a new AlarmClass RecordBuilder by copying an existing AlarmClass instance.
* @param other The existing instance to copy.
* @return A new AlarmClass RecordBuilder
*/
public static org.jlab.jaws.entity.AlarmClass.Builder newBuilder(org.jlab.jaws.entity.AlarmClass other) {
if (other == null) {
return new org.jlab.jaws.entity.AlarmClass.Builder();
} else {
return new org.jlab.jaws.entity.AlarmClass.Builder(other);
}
}
/**
* RecordBuilder for AlarmClass instances.
*/
@org.apache.avro.specific.AvroGenerated
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
/** The alarm category */
private java.lang.String category;
/** The alarm priority */
private org.jlab.jaws.entity.AlarmPriority priority;
/** Markdown formatted text describing the reason this alarm is necessary */
private java.lang.String rationale;
/** Markdown formatted text describing the corrective action to take when the alarm becomes active */
private java.lang.String correctiveaction;
/** Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example) */
private java.lang.String pointofcontactusername;
/** Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement */
private boolean latchable;
/** Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program. */
private boolean filterable;
/** The number of seconds of on-delay */
private java.lang.Long ondelayseconds;
/** The number of seconds of off-delay */
private java.lang.Long offdelayseconds;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$, MODEL$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(org.jlab.jaws.entity.AlarmClass.Builder other) {
super(other);
if (isValidValue(fields()[0], other.category)) {
this.category = data().deepCopy(fields()[0].schema(), other.category);
fieldSetFlags()[0] = other.fieldSetFlags()[0];
}
if (isValidValue(fields()[1], other.priority)) {
this.priority = data().deepCopy(fields()[1].schema(), other.priority);
fieldSetFlags()[1] = other.fieldSetFlags()[1];
}
if (isValidValue(fields()[2], other.rationale)) {
this.rationale = data().deepCopy(fields()[2].schema(), other.rationale);
fieldSetFlags()[2] = other.fieldSetFlags()[2];
}
if (isValidValue(fields()[3], other.correctiveaction)) {
this.correctiveaction = data().deepCopy(fields()[3].schema(), other.correctiveaction);
fieldSetFlags()[3] = other.fieldSetFlags()[3];
}
if (isValidValue(fields()[4], other.pointofcontactusername)) {
this.pointofcontactusername = data().deepCopy(fields()[4].schema(), other.pointofcontactusername);
fieldSetFlags()[4] = other.fieldSetFlags()[4];
}
if (isValidValue(fields()[5], other.latchable)) {
this.latchable = data().deepCopy(fields()[5].schema(), other.latchable);
fieldSetFlags()[5] = other.fieldSetFlags()[5];
}
if (isValidValue(fields()[6], other.filterable)) {
this.filterable = data().deepCopy(fields()[6].schema(), other.filterable);
fieldSetFlags()[6] = other.fieldSetFlags()[6];
}
if (isValidValue(fields()[7], other.ondelayseconds)) {
this.ondelayseconds = data().deepCopy(fields()[7].schema(), other.ondelayseconds);
fieldSetFlags()[7] = other.fieldSetFlags()[7];
}
if (isValidValue(fields()[8], other.offdelayseconds)) {
this.offdelayseconds = data().deepCopy(fields()[8].schema(), other.offdelayseconds);
fieldSetFlags()[8] = other.fieldSetFlags()[8];
}
}
/**
* Creates a Builder by copying an existing AlarmClass instance
* @param other The existing instance to copy.
*/
private Builder(org.jlab.jaws.entity.AlarmClass other) {
super(SCHEMA$, MODEL$);
if (isValidValue(fields()[0], other.category)) {
this.category = data().deepCopy(fields()[0].schema(), other.category);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.priority)) {
this.priority = data().deepCopy(fields()[1].schema(), other.priority);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.rationale)) {
this.rationale = data().deepCopy(fields()[2].schema(), other.rationale);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.correctiveaction)) {
this.correctiveaction = data().deepCopy(fields()[3].schema(), other.correctiveaction);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.pointofcontactusername)) {
this.pointofcontactusername = data().deepCopy(fields()[4].schema(), other.pointofcontactusername);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.latchable)) {
this.latchable = data().deepCopy(fields()[5].schema(), other.latchable);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.filterable)) {
this.filterable = data().deepCopy(fields()[6].schema(), other.filterable);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.ondelayseconds)) {
this.ondelayseconds = data().deepCopy(fields()[7].schema(), other.ondelayseconds);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.offdelayseconds)) {
this.offdelayseconds = data().deepCopy(fields()[8].schema(), other.offdelayseconds);
fieldSetFlags()[8] = true;
}
}
/**
* Gets the value of the 'category' field.
* The alarm category
* @return The value.
*/
public java.lang.String getCategory() {
return category;
}
/**
* Sets the value of the 'category' field.
* The alarm category
* @param value The value of 'category'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setCategory(java.lang.String value) {
validate(fields()[0], value);
this.category = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'category' field has been set.
* The alarm category
* @return True if the 'category' field has been set, false otherwise.
*/
public boolean hasCategory() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'category' field.
* The alarm category
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearCategory() {
category = null;
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'priority' field.
* The alarm priority
* @return The value.
*/
public org.jlab.jaws.entity.AlarmPriority getPriority() {
return priority;
}
/**
* Sets the value of the 'priority' field.
* The alarm priority
* @param value The value of 'priority'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setPriority(org.jlab.jaws.entity.AlarmPriority value) {
validate(fields()[1], value);
this.priority = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'priority' field has been set.
* The alarm priority
* @return True if the 'priority' field has been set, false otherwise.
*/
public boolean hasPriority() {
return fieldSetFlags()[1];
}
/**
* Clears the value of the 'priority' field.
* The alarm priority
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearPriority() {
priority = null;
fieldSetFlags()[1] = false;
return this;
}
/**
* Gets the value of the 'rationale' field.
* Markdown formatted text describing the reason this alarm is necessary
* @return The value.
*/
public java.lang.String getRationale() {
return rationale;
}
/**
* Sets the value of the 'rationale' field.
* Markdown formatted text describing the reason this alarm is necessary
* @param value The value of 'rationale'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setRationale(java.lang.String value) {
validate(fields()[2], value);
this.rationale = value;
fieldSetFlags()[2] = true;
return this;
}
/**
* Checks whether the 'rationale' field has been set.
* Markdown formatted text describing the reason this alarm is necessary
* @return True if the 'rationale' field has been set, false otherwise.
*/
public boolean hasRationale() {
return fieldSetFlags()[2];
}
/**
* Clears the value of the 'rationale' field.
* Markdown formatted text describing the reason this alarm is necessary
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearRationale() {
rationale = null;
fieldSetFlags()[2] = false;
return this;
}
/**
* Gets the value of the 'correctiveaction' field.
* Markdown formatted text describing the corrective action to take when the alarm becomes active
* @return The value.
*/
public java.lang.String getCorrectiveaction() {
return correctiveaction;
}
/**
* Sets the value of the 'correctiveaction' field.
* Markdown formatted text describing the corrective action to take when the alarm becomes active
* @param value The value of 'correctiveaction'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setCorrectiveaction(java.lang.String value) {
validate(fields()[3], value);
this.correctiveaction = value;
fieldSetFlags()[3] = true;
return this;
}
/**
* Checks whether the 'correctiveaction' field has been set.
* Markdown formatted text describing the corrective action to take when the alarm becomes active
* @return True if the 'correctiveaction' field has been set, false otherwise.
*/
public boolean hasCorrectiveaction() {
return fieldSetFlags()[3];
}
/**
* Clears the value of the 'correctiveaction' field.
* Markdown formatted text describing the corrective action to take when the alarm becomes active
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearCorrectiveaction() {
correctiveaction = null;
fieldSetFlags()[3] = false;
return this;
}
/**
* Gets the value of the 'pointofcontactusername' field.
* Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
* @return The value.
*/
public java.lang.String getPointofcontactusername() {
return pointofcontactusername;
}
/**
* Sets the value of the 'pointofcontactusername' field.
* Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
* @param value The value of 'pointofcontactusername'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setPointofcontactusername(java.lang.String value) {
validate(fields()[4], value);
this.pointofcontactusername = value;
fieldSetFlags()[4] = true;
return this;
}
/**
* Checks whether the 'pointofcontactusername' field has been set.
* Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
* @return True if the 'pointofcontactusername' field has been set, false otherwise.
*/
public boolean hasPointofcontactusername() {
return fieldSetFlags()[4];
}
/**
* Clears the value of the 'pointofcontactusername' field.
* Username (identifier) of point of contact. Full contact info should be obtained from user database (LDAP for example)
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearPointofcontactusername() {
pointofcontactusername = null;
fieldSetFlags()[4] = false;
return this;
}
/**
* Gets the value of the 'latchable' field.
* Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
* @return The value.
*/
public boolean getLatchable() {
return latchable;
}
/**
* Sets the value of the 'latchable' field.
* Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
* @param value The value of 'latchable'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setLatchable(boolean value) {
validate(fields()[5], value);
this.latchable = value;
fieldSetFlags()[5] = true;
return this;
}
/**
* Checks whether the 'latchable' field has been set.
* Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
* @return True if the 'latchable' field has been set, false otherwise.
*/
public boolean hasLatchable() {
return fieldSetFlags()[5];
}
/**
* Clears the value of the 'latchable' field.
* Indicates whether this alarm latches when activated and can only be cleared after an explicit acknowledgement
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearLatchable() {
fieldSetFlags()[5] = false;
return this;
}
/**
* Gets the value of the 'filterable' field.
* Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
* @return The value.
*/
public boolean getFilterable() {
return filterable;
}
/**
* Sets the value of the 'filterable' field.
* Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
* @param value The value of 'filterable'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setFilterable(boolean value) {
validate(fields()[6], value);
this.filterable = value;
fieldSetFlags()[6] = true;
return this;
}
/**
* Checks whether the 'filterable' field has been set.
* Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
* @return True if the 'filterable' field has been set, false otherwise.
*/
public boolean hasFilterable() {
return fieldSetFlags()[6];
}
/**
* Clears the value of the 'filterable' field.
* Indicates whether this alarm can be filtered out of view (typically done when a portion of the machine is turned off). Some alarms must always be monitored, regardless of program.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearFilterable() {
fieldSetFlags()[6] = false;
return this;
}
/**
* Gets the value of the 'ondelayseconds' field.
* The number of seconds of on-delay
* @return The value.
*/
public java.lang.Long getOndelayseconds() {
return ondelayseconds;
}
/**
* Sets the value of the 'ondelayseconds' field.
* The number of seconds of on-delay
* @param value The value of 'ondelayseconds'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setOndelayseconds(java.lang.Long value) {
validate(fields()[7], value);
this.ondelayseconds = value;
fieldSetFlags()[7] = true;
return this;
}
/**
* Checks whether the 'ondelayseconds' field has been set.
* The number of seconds of on-delay
* @return True if the 'ondelayseconds' field has been set, false otherwise.
*/
public boolean hasOndelayseconds() {
return fieldSetFlags()[7];
}
/**
* Clears the value of the 'ondelayseconds' field.
* The number of seconds of on-delay
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearOndelayseconds() {
ondelayseconds = null;
fieldSetFlags()[7] = false;
return this;
}
/**
* Gets the value of the 'offdelayseconds' field.
* The number of seconds of off-delay
* @return The value.
*/
public java.lang.Long getOffdelayseconds() {
return offdelayseconds;
}
/**
* Sets the value of the 'offdelayseconds' field.
* The number of seconds of off-delay
* @param value The value of 'offdelayseconds'.
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder setOffdelayseconds(java.lang.Long value) {
validate(fields()[8], value);
this.offdelayseconds = value;
fieldSetFlags()[8] = true;
return this;
}
/**
* Checks whether the 'offdelayseconds' field has been set.
* The number of seconds of off-delay
* @return True if the 'offdelayseconds' field has been set, false otherwise.
*/
public boolean hasOffdelayseconds() {
return fieldSetFlags()[8];
}
/**
* Clears the value of the 'offdelayseconds' field.
* The number of seconds of off-delay
* @return This builder.
*/
public org.jlab.jaws.entity.AlarmClass.Builder clearOffdelayseconds() {
offdelayseconds = null;
fieldSetFlags()[8] = false;
return this;
}
@Override
@SuppressWarnings("unchecked")
public AlarmClass build() {
try {
AlarmClass record = new AlarmClass();
record.category = fieldSetFlags()[0] ? this.category : (java.lang.String) defaultValue(fields()[0]);
record.priority = fieldSetFlags()[1] ? this.priority : (org.jlab.jaws.entity.AlarmPriority) defaultValue(fields()[1]);
record.rationale = fieldSetFlags()[2] ? this.rationale : (java.lang.String) defaultValue(fields()[2]);
record.correctiveaction = fieldSetFlags()[3] ? this.correctiveaction : (java.lang.String) defaultValue(fields()[3]);
record.pointofcontactusername = fieldSetFlags()[4] ? this.pointofcontactusername : (java.lang.String) defaultValue(fields()[4]);
record.latchable = fieldSetFlags()[5] ? this.latchable : (java.lang.Boolean) defaultValue(fields()[5]);
record.filterable = fieldSetFlags()[6] ? this.filterable : (java.lang.Boolean) defaultValue(fields()[6]);
record.ondelayseconds = fieldSetFlags()[7] ? this.ondelayseconds : (java.lang.Long) defaultValue(fields()[7]);
record.offdelayseconds = fieldSetFlags()[8] ? this.offdelayseconds : (java.lang.Long) defaultValue(fields()[8]);
return record;
} catch (org.apache.avro.AvroMissingFieldException e) {
throw e;
} catch (java.lang.Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumWriter
WRITER$ = (org.apache.avro.io.DatumWriter)MODEL$.createDatumWriter(SCHEMA$);
@Override public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumReader
READER$ = (org.apache.avro.io.DatumReader)MODEL$.createDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
@Override protected boolean hasCustomCoders() { return true; }
@Override public void customEncode(org.apache.avro.io.Encoder out)
throws java.io.IOException
{
out.writeString(this.category);
out.writeEnum(this.priority.ordinal());
out.writeString(this.rationale);
out.writeString(this.correctiveaction);
out.writeString(this.pointofcontactusername);
out.writeBoolean(this.latchable);
out.writeBoolean(this.filterable);
if (this.ondelayseconds == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeLong(this.ondelayseconds);
}
if (this.offdelayseconds == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeLong(this.offdelayseconds);
}
}
@Override public void customDecode(org.apache.avro.io.ResolvingDecoder in)
throws java.io.IOException
{
org.apache.avro.Schema.Field[] fieldOrder = in.readFieldOrderIfDiff();
if (fieldOrder == null) {
this.category = in.readString();
this.priority = org.jlab.jaws.entity.AlarmPriority.values()[in.readEnum()];
this.rationale = in.readString();
this.correctiveaction = in.readString();
this.pointofcontactusername = in.readString();
this.latchable = in.readBoolean();
this.filterable = in.readBoolean();
if (in.readIndex() != 1) {
in.readNull();
this.ondelayseconds = null;
} else {
this.ondelayseconds = in.readLong();
}
if (in.readIndex() != 1) {
in.readNull();
this.offdelayseconds = null;
} else {
this.offdelayseconds = in.readLong();
}
} else {
for (int i = 0; i < 9; i++) {
switch (fieldOrder[i].pos()) {
case 0:
this.category = in.readString();
break;
case 1:
this.priority = org.jlab.jaws.entity.AlarmPriority.values()[in.readEnum()];
break;
case 2:
this.rationale = in.readString();
break;
case 3:
this.correctiveaction = in.readString();
break;
case 4:
this.pointofcontactusername = in.readString();
break;
case 5:
this.latchable = in.readBoolean();
break;
case 6:
this.filterable = in.readBoolean();
break;
case 7:
if (in.readIndex() != 1) {
in.readNull();
this.ondelayseconds = null;
} else {
this.ondelayseconds = in.readLong();
}
break;
case 8:
if (in.readIndex() != 1) {
in.readNull();
this.offdelayseconds = null;
} else {
this.offdelayseconds = in.readLong();
}
break;
default:
throw new java.io.IOException("Corrupt ResolvingDecoder.");
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy