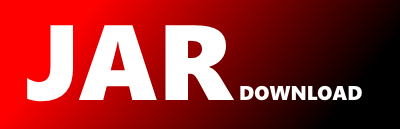
org.jleopard.session.SqlSession Maven / Gradle / Ivy
Show all versions of jleopard Show documentation
package org.jleopard.session;
import java.sql.ResultSet;
import java.util.List;
import org.jleopard.exception.SqlSessionException;
import org.jleopard.pageHelper.PageInfo;
/**
* Copyright (c) 2018, Chen_9g 陈刚 ([email protected]).
*
* DateTime 2018/4/8
*
* Find a way for success and not make excuses for failure.
*
* @see com.leopardframework.core.session.sessionFactory.SessionDirectImpl
* 管理与数据库操作的方法接口
*/
public interface SqlSession {
/**
* -----------------------Save---------------------------------
**/
int Save(T entity) throws SqlSessionException;
int SaveMore(List list) throws SqlSessionException;
int MySql(String sql, Object... args) throws SqlSessionException;
/**
* -----------------------Delete---------------------------------
**/
int Delete(T entity) throws SqlSessionException;
int Delete(Class cls, Object... primaryKeys) throws SqlSessionException;
/**
* -----------------------Update---------------------------------
**/
// int Update(Object entity) throws SQLException;
int Update(T entity, Object primaryKey) throws SqlSessionException;
/**
* -----------------------Get---------------------------------
**/
ResultSet Get(String sql, Object... args) throws SqlSessionException;
List Get(Class cls1, Class> cls2, String where, Object... args) throws SqlSessionException;
List Get(Class cls, String where, Object... args) throws SqlSessionException;
T Get(T entity) throws SqlSessionException;
List Get(Class cls, Object... primaryKeys) throws SqlSessionException;
List Get(Class cls) throws SqlSessionException;
PageInfo Get(Class cls, int page, int pageSize) throws SqlSessionException;
PageInfo Get(Class cls1,Class>cls2 ,int page, int pageSize) throws SqlSessionException;
// List Get(String sql,Object... args);
/**
* -----------------------Commit and rollback---------------------------------
**/
void Commit()throws SqlSessionException;
void Rollback() throws SqlSessionException;
/**
* -----------------------Close---------------------------------
**/
void Stop() throws SqlSessionException;
void Close() throws SqlSessionException;
}