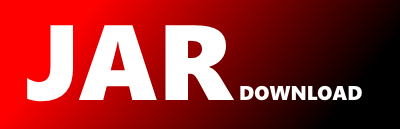
org.jmmo.tuple.Tuple1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples Show documentation
Show all versions of tuples Show documentation
Convenient java tuples library
package org.jmmo.tuple;
import java.util.Comparator;
import java.util.Iterator;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Function;
public class Tuple1 implements Tuple, Comparable> {
private static final long serialVersionUID = -8854900772811744378L;
private final T0 value0;
protected Tuple1(T0 value0) {
this.value0 = value0;
}
public static Tuple1 of(V0 v0) {
return new Tuple1<>(v0);
}
@SuppressWarnings("unchecked")
public static Tuple1 fromArray(A[] values) {
return new Tuple1<>((V) values[0]);
}
public static Tuple1 fromIterator(Iterator iterator) {
return new Tuple1<>(iterator.next());
}
public void unfold(Consumer valueConsumer) {
valueConsumer.accept(getValue0());
}
public R unfoldResult(Function valueFunction) {
return valueFunction.apply(getValue0());
}
public T0 getValue0() {
return value0;
}
@Override public int getSize() {
return 1;
}
@SuppressWarnings("unchecked")
@Override public E get(int i) {
switch (i) {
case 0: return (E) getValue0();
}
throw new IndexOutOfBoundsException("Tuple1 contains one element but " + i + " element requested");
}
@Override public Object[] toArray() {
return new Object[] { getValue0() };
}
protected static final Comparator> nullComparator = Comparator.nullsFirst(Comparator.naturalOrder());
@SuppressWarnings("unchecked")
@Override public int compareTo(Tuple1 o) {
return nullComparator.compare((Comparable) this.getValue0(), (Comparable) o.getValue0());
}
@Override public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Tuple1)) return false;
Tuple1> tuple1 = (Tuple1>) o;
return Objects.equals(getValue0(), tuple1.getValue0());
}
@Override public int hashCode() {
return Objects.hash(getValue0());
}
@Override public String toString() {
return "Tuple1{" +
"value0=" + getValue0() +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy