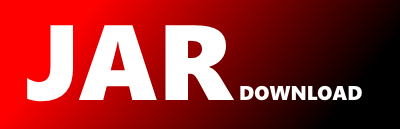
org.jmmo.tuple.Tuple6 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tuples Show documentation
Show all versions of tuples Show documentation
Convenient java tuples library
package org.jmmo.tuple;
import org.jmmo.function.Consumer6;
import org.jmmo.function.Function6;
import java.util.Comparator;
import java.util.Iterator;
import java.util.Objects;
public class Tuple6 implements Tuple, Comparable> {
private static final long serialVersionUID = 7059741881567341693L;
private final T0 value0;
private final T1 value1;
private final T2 value2;
private final T3 value3;
private final T4 value4;
private final T5 value5;
protected Tuple6(T0 value0, T1 value1, T2 value2, T3 value3, T4 value4, T5 value5) {
this.value0 = value0;
this.value1 = value1;
this.value2 = value2;
this.value3 = value3;
this.value4 = value4;
this.value5 = value5;
}
public static Tuple6 of(V0 v0, V1 v1, V2 v2, V3 v3, V4 v4, V5 v5) {
return new Tuple6<>(v0, v1, v2, v3, v4, v5);
}
@SuppressWarnings("unchecked")
public static
Tuple6 fromArray(A[] values) {
return new Tuple6<>((V0) values[0], (V1) values[1], (V2) values[2], (V3) values[3], (V4) values[4], (V5) values[5]);
}
@SuppressWarnings("unchecked")
public static
Tuple6 fromIterator(Iterator iterator) {
return new Tuple6<>((V0) iterator.next(), (V1) iterator.next(), (V2) iterator.next(), (V3) iterator.next(),
(V4) iterator.next(), (V5) iterator.next());
}
public void unfold(Consumer6 valueConsumer) {
valueConsumer.accept(getValue0(), getValue1(), getValue2(), getValue3(), getValue4(), getValue5());
}
public R unfoldResult(Function6 valueFunction) {
return valueFunction.apply(getValue0(), getValue1(), getValue2(), getValue3(), getValue4(), getValue5());
}
public T0 getValue0() {
return value0;
}
public T1 getValue1() {
return value1;
}
public T2 getValue2() {
return value2;
}
public T3 getValue3() {
return value3;
}
public T4 getValue4() {
return value4;
}
public T5 getValue5() {
return value5;
}
@Override public int getSize() {
return 6;
}
@SuppressWarnings("unchecked")
@Override public E get(int i) {
switch (i) {
case 0: return (E) getValue0();
case 1: return (E) getValue1();
case 2: return (E) getValue2();
case 3: return (E) getValue3();
case 4: return (E) getValue4();
case 5: return (E) getValue5();
}
throw new IndexOutOfBoundsException("Tuple6 contains six elements but " + i + " element requested");
}
@Override public Object[] toArray() {
return new Object[] { getValue0(), getValue1(), getValue2(), getValue3(), getValue4(), getValue5() };
}
@SuppressWarnings("unchecked")
private static final Comparator comparator =
Comparator.>comparing(tuple -> (Comparable) tuple.getValue0(), Tuple1.nullComparator)
.thenComparing(
Comparator.>comparing(tuple -> (Comparable) tuple.getValue1(), Tuple1.nullComparator))
.thenComparing(
Comparator.>comparing(tuple -> (Comparable) tuple.getValue2(), Tuple1.nullComparator))
.thenComparing(
Comparator.>comparing(tuple -> (Comparable) tuple.getValue3(), Tuple1.nullComparator))
.thenComparing(
Comparator.>comparing(tuple -> (Comparable) tuple.getValue4(), Tuple1.nullComparator))
.thenComparing(
Comparator.>comparing(tuple -> (Comparable) tuple.getValue5(), Tuple1.nullComparator));
@SuppressWarnings("unchecked")
@Override public int compareTo(Tuple6 o) {
return comparator.compare(this, o);
}
@Override public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Tuple6)) return false;
Tuple6, ?, ?, ?, ?, ?> tuple6 = (Tuple6, ?, ?, ?, ?, ?>) o;
return Objects.equals(getValue0(), tuple6.getValue0()) &&
Objects.equals(getValue1(), tuple6.getValue1()) &&
Objects.equals(getValue2(), tuple6.getValue2()) &&
Objects.equals(getValue3(), tuple6.getValue3()) &&
Objects.equals(getValue4(), tuple6.getValue4()) &&
Objects.equals(getValue5(), tuple6.getValue5());
}
@Override public int hashCode() {
return Objects.hash(getValue0(), getValue1(), getValue2(), getValue3(), getValue4(), getValue5());
}
@Override public String toString() {
return "Tuple5{" +
"value0=" + getValue0() +
", value1=" + getValue1() +
", value2=" + getValue2() +
", value3=" + getValue3() +
", value4=" + getValue4() +
", value5=" + getValue5() +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy