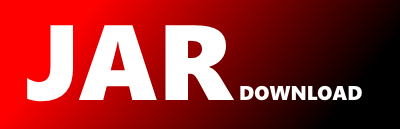
com.jme3.scene.plugins.blender.math.Matrix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-blender Show documentation
Show all versions of jme3-blender Show documentation
jMonkeyEngine is a 3D game engine for adventurous Java developers
The newest version!
package com.jme3.scene.plugins.blender.math; import java.text.DecimalFormat; import org.ejml.ops.CommonOps; import org.ejml.simple.SimpleMatrix; import org.ejml.simple.SimpleSVD; import com.jme3.math.FastMath; /** * Encapsulates a 4x4 matrix * * */ public class Matrix extends SimpleMatrix { private static final long serialVersionUID = 2396600537315902559L; public Matrix(int rows, int cols) { super(rows, cols); } /** * Copy constructor */ public Matrix(SimpleMatrix m) { super(m); } public Matrix(double[][] data) { super(data); } public static Matrix identity(int size) { Matrix result = new Matrix(size, size); CommonOps.setIdentity(result.mat); return result; } public Matrix pseudoinverse() { return this.pseudoinverse(1); } @SuppressWarnings("unchecked") public Matrix pseudoinverse(double lambda) { SimpleSVD
builds a rotation from a *simpleSVD = this.svd(); SimpleMatrix U = simpleSVD.getU(); SimpleMatrix S = simpleSVD.getW(); SimpleMatrix V = simpleSVD.getV(); int N = Math.min(this.numRows(),this.numCols()); double maxSingular = 0; for( int i = 0; i < N; ++i ) { if( S.get(i, i) > maxSingular ) { maxSingular = S.get(i, i); } } double tolerance = FastMath.DBL_EPSILON * Math.max(this.numRows(),this.numCols()) * maxSingular; for(int i=0;i setRotationQuaternion Quaternion
. * * @param quat * the quaternion to build the rotation from. * @throws NullPointerException * if quat is null. */ public void setRotationQuaternion(DQuaternion quat) { quat.toRotationMatrix(this); } public DTransform toTransform() { DTransform result = new DTransform(); result.setTranslation(this.toTranslationVector()); result.setRotation(this.toRotationQuat()); result.setScale(this.toScaleVector()); return result; } public Vector3d toTranslationVector() { return new Vector3d(this.get(0, 3), this.get(1, 3), this.get(2, 3)); } public DQuaternion toRotationQuat() { DQuaternion quat = new DQuaternion(); quat.fromRotationMatrix(this.get(0, 0), this.get(0, 1), this.get(0, 2), this.get(1, 0), this.get(1, 1), this.get(1, 2), this.get(2, 0), this.get(2, 1), this.get(2, 2)); return quat; } /** * Retrieves the scale vector from the matrix and stores it into a given * vector. */ public Vector3d toScaleVector() { Vector3d result = new Vector3d(); this.toScaleVector(result); return result; } /** * Retrieves the scale vector from the matrix and stores it into a given * vector. * * @param vector the vector where the scale will be stored */ public void toScaleVector(Vector3d vector) { double scaleX = Math.sqrt(this.get(0, 0) * this.get(0, 0) + this.get(1, 0) * this.get(1, 0) + this.get(2, 0) * this.get(2, 0)); double scaleY = Math.sqrt(this.get(0, 1) * this.get(0, 1) + this.get(1, 1) * this.get(1, 1) + this.get(2, 1) * this.get(2, 1)); double scaleZ = Math.sqrt(this.get(0, 2) * this.get(0, 2) + this.get(1, 2) * this.get(1, 2) + this.get(2, 2) * this.get(2, 2)); vector.set(scaleX, scaleY, scaleZ); } }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy