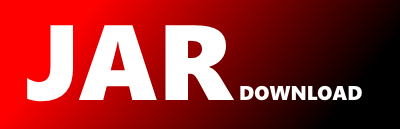
com.jme3.scene.plugins.blender.meshes.MeshBuffers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-blender Show documentation
Show all versions of jme3-blender Show documentation
jMonkeyEngine is a 3D game engine for adventurous Java developers
The newest version!
package com.jme3.scene.plugins.blender.meshes;
import java.nio.Buffer;
import java.nio.ByteBuffer;
import java.nio.FloatBuffer;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.NavigableMap;
import java.util.TreeMap;
import com.jme3.math.FastMath;
import com.jme3.math.Vector2f;
import com.jme3.math.Vector3f;
import com.jme3.scene.VertexBuffer;
import com.jme3.scene.VertexBuffer.Format;
import com.jme3.scene.VertexBuffer.Type;
import com.jme3.scene.VertexBuffer.Usage;
import com.jme3.util.BufferUtils;
/**
* A class that aggregates the mesh data to prepare proper buffers. The buffers refer only to ONE material.
*
* @author Marcin Roguski (Kaelthas)
*/
/* package */class MeshBuffers {
private static final int MAXIMUM_WEIGHTS_PER_VERTEX = 4;
/** The material index. */
private final int materialIndex;
/** The vertices. */
private List verts = new ArrayList();
/** The normals. */
private List normals = new ArrayList();
/** The UV coordinate sets. */
private Map> uvCoords = new HashMap>();
/** The vertex colors. */
private List vertColors = new ArrayList();
/** The indexes. */
private List indexes = new ArrayList();
/** The maximum weights count assigned to a single vertex. Used during weights normalization. */
private int maximumWeightsPerVertex;
/** A list of mapping between weights and indexes. Each entry for the proper vertex. */
private List> boneWeightAndIndexes = new ArrayList>();
/**
* Constructor stores only the material index value.
* @param materialIndex
* the material index
*/
public MeshBuffers(int materialIndex) {
this.materialIndex = materialIndex;
}
/**
* @return the material index
*/
public int getMaterialIndex() {
return materialIndex;
}
/**
* @return indexes buffer
*/
public Buffer getIndexBuffer() {
if (indexes.size() <= Short.MAX_VALUE) {
short[] indices = new short[indexes.size()];
for (int i = 0; i < indexes.size(); ++i) {
indices[i] = indexes.get(i).shortValue();
}
return BufferUtils.createShortBuffer(indices);
} else {
int[] indices = new int[indexes.size()];
for (int i = 0; i < indexes.size(); ++i) {
indices[i] = indexes.get(i).intValue();
}
return BufferUtils.createIntBuffer(indices);
}
}
/**
* @return positions buffer
*/
public VertexBuffer getPositionsBuffer() {
VertexBuffer positionBuffer = new VertexBuffer(Type.Position);
Vector3f[] data = verts.toArray(new Vector3f[verts.size()]);
positionBuffer.setupData(Usage.Static, 3, Format.Float, BufferUtils.createFloatBuffer(data));
return positionBuffer;
}
/**
* @return normals buffer
*/
public VertexBuffer getNormalsBuffer() {
VertexBuffer positionBuffer = new VertexBuffer(Type.Normal);
Vector3f[] data = normals.toArray(new Vector3f[normals.size()]);
positionBuffer.setupData(Usage.Static, 3, Format.Float, BufferUtils.createFloatBuffer(data));
return positionBuffer;
}
/**
* @return bone buffers
*/
public BoneBuffersData getBoneBuffers() {
BoneBuffersData result = null;
if (maximumWeightsPerVertex > 0) {
this.normalizeBoneBuffers(MAXIMUM_WEIGHTS_PER_VERTEX);
maximumWeightsPerVertex = MAXIMUM_WEIGHTS_PER_VERTEX;
FloatBuffer weightsFloatData = BufferUtils.createFloatBuffer(boneWeightAndIndexes.size() * MAXIMUM_WEIGHTS_PER_VERTEX);
ByteBuffer indicesData = BufferUtils.createByteBuffer(boneWeightAndIndexes.size() * MAXIMUM_WEIGHTS_PER_VERTEX);
int index = 0;
for (Map boneBuffersData : boneWeightAndIndexes) {
if (boneBuffersData.size() > 0) {
int count = 0;
for (Entry entry : boneBuffersData.entrySet()) {
weightsFloatData.put(index * MAXIMUM_WEIGHTS_PER_VERTEX + count, entry.getKey());
indicesData.put(index * MAXIMUM_WEIGHTS_PER_VERTEX + count, entry.getValue().byteValue());
++count;
}
} else {
// if no bone is assigned to this vertex then attach it to the 0-indexed root bone
weightsFloatData.put(index * MAXIMUM_WEIGHTS_PER_VERTEX, 1.0f);
indicesData.put(index * MAXIMUM_WEIGHTS_PER_VERTEX, (byte) 0);
}
++index;
}
VertexBuffer verticesWeights = new VertexBuffer(Type.BoneWeight);
verticesWeights.setupData(Usage.CpuOnly, maximumWeightsPerVertex, Format.Float, weightsFloatData);
VertexBuffer verticesWeightsIndices = new VertexBuffer(Type.BoneIndex);
verticesWeightsIndices.setupData(Usage.CpuOnly, maximumWeightsPerVertex, Format.UnsignedByte, indicesData);
result = new BoneBuffersData(maximumWeightsPerVertex, verticesWeights, verticesWeightsIndices);
}
return result;
}
/**
* @return UV coordinates sets
*/
public Map> getUvCoords() {
return uvCoords;
}
/**
* @return true if vertex colors are used and false otherwise
*/
public boolean areVertexColorsUsed() {
return vertColors.size() > 0;
}
/**
* @return vertex colors buffer
*/
public ByteBuffer getVertexColorsBuffer() {
ByteBuffer result = null;
if (vertColors.size() > 0) {
result = BufferUtils.createByteBuffer(4 * vertColors.size());
for (byte[] v : vertColors) {
if (v != null) {
result.put(v[0]).put(v[1]).put(v[2]).put(v[3]);
} else {
result.put((byte) 0).put((byte) 0).put((byte) 0).put((byte) 0);
}
}
result.flip();
}
return result;
}
/**
* @return true if indexes can be shorts' and false if they need to be ints'
*/
public boolean isShortIndexBuffer() {
return indexes.size() <= Short.MAX_VALUE;
}
/**
* Appends a vertex and normal to the buffers.
* @param vert
* vertex
* @param normal
* normal vector
*/
public void append(Vector3f vert, Vector3f normal) {
int index = this.indexOf(vert, normal, null);
if (index >= 0) {
indexes.add(index);
} else {
indexes.add(verts.size());
verts.add(vert);
normals.add(normal);
}
}
/**
* Appends the face data to the buffers.
* @param smooth
* tells if the face is smooth or flat
* @param verts
* the vertices
* @param normals
* the normals
* @param uvCoords
* the UV coordinates
* @param vertColors
* the vertex colors
* @param vertexGroups
* the vertex groups
*/
public void append(boolean smooth, Vector3f[] verts, Vector3f[] normals, Map> uvCoords, byte[][] vertColors, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy