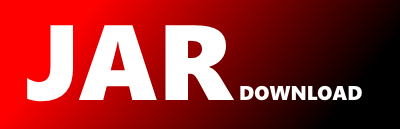
com.jme3.scene.plugins.blender.textures.UserUVCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-blender Show documentation
Show all versions of jme3-blender Show documentation
jMonkeyEngine is a 3D game engine for adventurous Java developers
The newest version!
package com.jme3.scene.plugins.blender.textures;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.jme3.math.Vector2f;
/**
* A collection of UV coordinates. The coords are stored in groups defined by the material index and their UV set name.
*
* @author Kaelthas (Marcin Roguski)
*/
public class UserUVCollection {
/** A map between material number and UV coordinates of mesh that has this material applied. */
private Map>> uvCoordinates = new HashMap>>();
/** A map between vertex index and its UV coordinates. */
private Map> uvsMap = new HashMap>();
/**
* Adds a single UV coordinates for a specified vertex index.
* @param materialIndex
* the material index
* @param uvSetName
* the UV set name
* @param uv
* the added UV coordinates
* @param jmeVertexIndex
* the index of the vertex in result jme mesh
*/
public void addUV(int materialIndex, String uvSetName, Vector2f uv, int jmeVertexIndex) {
// first get all UV sets for the specified material ...
LinkedHashMap> uvsForMaterial = uvCoordinates.get(materialIndex);
if (uvsForMaterial == null) {
uvsForMaterial = new LinkedHashMap>();
uvCoordinates.put(materialIndex, uvsForMaterial);
}
// ... then fetch the UVS for the specified UV set name ...
List uvsForName = uvsForMaterial.get(uvSetName);
if (uvsForName == null) {
uvsForName = new ArrayList();
uvsForMaterial.put(uvSetName, uvsForName);
}
// ... add the UV coordinates to the proper list ...
uvsForName.add(uv);
// ... and add the mapping of the UV coordinates to a vertex index for the specified UV set
Map uvToVertexIndexMapping = uvsMap.get(uvSetName);
if (uvToVertexIndexMapping == null) {
uvToVertexIndexMapping = new HashMap();
uvsMap.put(uvSetName, uvToVertexIndexMapping);
}
uvToVertexIndexMapping.put(jmeVertexIndex, uv);
}
/**
* @param uvSetName
* the name of the UV set
* @param vertexIndex
* the vertex index corresponds to the index in jme mesh and not the original one in blender
* @return a pre-existing coordinate vector
*/
public Vector2f getUVForVertex(String uvSetName, int vertexIndex) {
return uvsMap.get(uvSetName).get(vertexIndex);
}
/**
* @param materialNumber
* the material number that is appied to the mesh
* @return UV coordinates of vertices that belong to the required mesh part
*/
public LinkedHashMap> getUVCoordinates(int materialNumber) {
return uvCoordinates.get(materialNumber);
}
/**
* @return indicates if the mesh has UV coordinates
*/
public boolean hasUVCoordinates() {
return uvCoordinates.size() > 0;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy