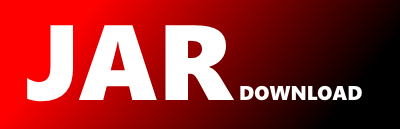
com.jme3.system.AppSettings Maven / Gradle / Ivy
Show all versions of jme3-core Show documentation
/*
* Copyright (c) 2009-2022 jMonkeyEngine
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* * Neither the name of 'jMonkeyEngine' nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.jme3.system;
import com.jme3.opencl.DefaultPlatformChooser;
import com.jme3.opencl.PlatformChooser;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.prefs.BackingStoreException;
import java.util.prefs.Preferences;
/**
* AppSettings
provides a store of configuration
* to be used by the application.
*
* By default only the {@link JmeContext context} uses the configuration,
* however the user may set and retrieve the settings as well.
* The settings can be stored either in the Java preferences
* (using {@link #save(java.lang.String) }) or
* a .properties file (using {@link #save(java.io.OutputStream) }).
*
* @author Kirill Vainer
*/
public final class AppSettings extends HashMap {
private static final long serialVersionUID = 1L;
private static final AppSettings defaults = new AppSettings(false);
/**
* Use LWJGL as the display system and force using the OpenGL2.0 renderer.
*
* If the underlying system does not support OpenGL2.0, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL2 = "LWJGL-OpenGL2";
/**
* Use LWJGL as the display system and force using the core OpenGL3.2 renderer.
*
* If the underlying system does not support OpenGL3.2, then the context
* initialization will throw an exception. Note that currently jMonkeyEngine
* does not have any shaders that support OpenGL3.2 therefore this
* option is not useful.
*
* Note: OpenGL 3.2 is used to give 3.x support to Mac users.
*
* @deprecated Previously meant 3.2, use LWJGL_OPENGL32 or LWJGL_OPENGL30
* @see AppSettings#setRenderer(java.lang.String)
*/
@Deprecated
public static final String LWJGL_OPENGL3 = "LWJGL-OpenGL3";
/**
* Use LWJGL as the display system and force using the core OpenGL3.0 renderer.
*
* If the underlying system does not support OpenGL3.0, then the context
* initialization will throw an exception. Note that currently jMonkeyEngine
* does not have any shaders that support OpenGL3.0 therefore this
* option is not useful.
*
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL30 = "LWJGL-OpenGL30";
/**
* Use LWJGL as the display system and force using the core OpenGL3.1 renderer.
*
* If the underlying system does not support OpenGL3.1, then the context
* initialization will throw an exception. Note that currently jMonkeyEngine
* does not have any shaders that support OpenGL3.0 therefore this
* option is not useful.
*
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL31 = "LWJGL-OpenGL31";
/**
* Use LWJGL as the display system and force using the core OpenGL3.2 renderer.
*
* If the underlying system does not support OpenGL3.2, then the context
* initialization will throw an exception. Note that currently jMonkeyEngine
* does not have any shaders that support OpenGL3.2 therefore this
* option is not useful.
*
* Note: OpenGL 3.2 is used to give 3.x support to Mac users.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL32 = LWJGL_OPENGL3;
/**
* Use LWJGL as the display system and force using the OpenGL3.3 renderer.
*
* If the underlying system does not support OpenGL3.3, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL33 = "LWJGL-OpenGL33";
/**
* Use LWJGL as the display system and force using the OpenGL4.0 renderer.
*
* If the underlying system does not support OpenGL4.0, then the context
* initialization will throw an exception.
*
* @deprecated Use LWJGL_OPENGL40
* @see AppSettings#setRenderer(java.lang.String)
*/
@Deprecated
public static final String LWJGL_OPENGL4 = "LWJGL-OpenGL4";
/**
* Use LWJGL as the display system and force using the OpenGL4.0 renderer.
*
* If the underlying system does not support OpenGL4.0, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL40 = LWJGL_OPENGL4;
/**
* Use LWJGL as the display system and force using the OpenGL4.1 renderer.
*
* If the underlying system does not support OpenGL4.1, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL41 = "LWJGL-OpenGL41";
/**
* Use LWJGL as the display system and force using the OpenGL4.2 renderer.
*
* If the underlying system does not support OpenGL4.2, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL42 = "LWJGL-OpenGL42";
/**
* Use LWJGL as the display system and force using the OpenGL4.3 renderer.
*
* If the underlying system does not support OpenGL4.3, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL43 = "LWJGL-OpenGL43";
/**
* Use LWJGL as the display system and force using the OpenGL4.4 renderer.
*
* If the underlying system does not support OpenGL4.4, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL44 = "LWJGL-OpenGL44";
/**
* Use LWJGL as the display system and force using the OpenGL4.5 renderer.
*
* If the underlying system does not support OpenGL4.5, then the context
* initialization will throw an exception.
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String LWJGL_OPENGL45 = "LWJGL-OpenGL45";
/**
* Use the LWJGL OpenAL based renderer for audio capabilities.
*
* @see AppSettings#setAudioRenderer(java.lang.String)
*/
public static final String LWJGL_OPENAL = "LWJGL";
/**
* Use the Android MediaPlayer / SoundPool based renderer for Android audio capabilities.
*
* NOTE: Supports Android 2.2+ platforms.
*
* @see AppSettings#setAudioRenderer(java.lang.String)
* @deprecated This audio renderer has too many limitations.
* use {@link #ANDROID_OPENAL_SOFT} instead.
*/
@Deprecated
public static final String ANDROID_MEDIAPLAYER = "MediaPlayer";
/**
* Use the OpenAL Soft based renderer for Android audio capabilities.
*
* This is the current default for Android platforms.
* NOTE: Only to be used on Android 2.3+ platforms due to using OpenSL.
*
* @see AppSettings#setAudioRenderer(java.lang.String)
*/
public static final String ANDROID_OPENAL_SOFT = "OpenAL_SOFT";
/**
* Use JogAmp's JOGL as the display system, with the OpenGL forward compatible profile
*
* N.B: This backend is EXPERIMENTAL
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String JOGL_OPENGL_FORWARD_COMPATIBLE = "JOGL_OPENGL_FORWARD_COMPATIBLE";
/**
* Use JogAmp's JOGL as the display system, with the backward compatible profile
*
* N.B: This backend is EXPERIMENTAL
*
* @see AppSettings#setRenderer(java.lang.String)
*/
public static final String JOGL_OPENGL_BACKWARD_COMPATIBLE = "JOGL_OPENGL_BACKWARD_COMPATIBLE";
/**
* Use JogAmp's JOAL as the audio renderer.
*
* N.B: This backend is EXPERIMENTAL
*
* @see AppSettings#setAudioRenderer(java.lang.String)
*/
public static final String JOAL = "JOAL";
static {
defaults.put("CenterWindow", true);
defaults.put("Width", 640);
defaults.put("Height", 480);
defaults.put("WindowWidth", Integer.MIN_VALUE);
defaults.put("WindowHeight", Integer.MIN_VALUE);
defaults.put("BitsPerPixel", 24);
defaults.put("Frequency", 60);
defaults.put("DepthBits", 24);
defaults.put("StencilBits", 0);
defaults.put("Samples", 0);
defaults.put("Fullscreen", false);
defaults.put("Title", JmeVersion.FULL_NAME);
defaults.put("Renderer", LWJGL_OPENGL32);
defaults.put("AudioRenderer", LWJGL_OPENAL);
defaults.put("DisableJoysticks", true);
defaults.put("UseInput", true);
defaults.put("VSync", true);
defaults.put("FrameRate", -1);
defaults.put("SettingsDialogImage", "/com/jme3/app/Monkey.png");
defaults.put("MinHeight", 0);
defaults.put("MinWidth", 0);
defaults.put("GammaCorrection", true);
defaults.put("Resizable", false);
defaults.put("SwapBuffers", true);
defaults.put("OpenCL", false);
defaults.put("OpenCLPlatformChooser", DefaultPlatformChooser.class.getName());
defaults.put("UseRetinaFrameBuffer", false);
defaults.put("WindowYPosition", 0);
defaults.put("WindowXPosition", 0);
// defaults.put("Icons", null);
}
/**
* Create a new instance of AppSettings
.
*
* If loadDefaults
is true, then the default settings
* will be set on the AppSettings.
* Use false if you want to change some settings but you would like the
* application to load settings from previous launches.
*
* @param loadDefaults If default settings are to be loaded.
*/
public AppSettings(boolean loadDefaults) {
if (loadDefaults) {
putAll(defaults);
}
}
/**
* Copies all settings from other
to this
* AppSettings.
*
* Any settings that are specified in other will overwrite settings
* set on this AppSettings.
*
* @param other The AppSettings to copy the settings from
*/
public void copyFrom(AppSettings other) {
this.putAll(other);
}
/**
* Same as {@link #copyFrom(com.jme3.system.AppSettings) }, except
* doesn't overwrite settings that are already set.
*
* @param other The AppSettings to merge the settings from
*/
public void mergeFrom(AppSettings other) {
for (String key : other.keySet()) {
if (!this.containsKey(key)) {
put(key, other.get(key));
}
}
}
/**
* Loads the settings from the given properties input stream.
*
* @param in The InputStream to load from
* @throws IOException If an IOException occurs
*
* @see #save(java.io.OutputStream)
*/
public void load(InputStream in) throws IOException {
Properties props = new Properties();
props.load(in);
for (Map.Entry