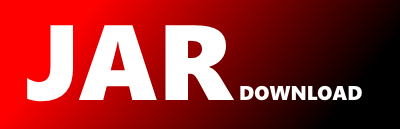
com.jme3.input.vr.osvr.OSVRInput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-vr Show documentation
Show all versions of jme3-vr Show documentation
jMonkeyEngine is a 3-D game engine for adventurous Java developers
package com.jme3.input.vr.osvr;
import java.util.logging.Logger;
import com.jme3.app.VREnvironment;
import com.jme3.input.vr.VRInputAPI;
import com.jme3.input.vr.VRInputType;
import com.jme3.input.vr.VRTrackedController;
import com.jme3.math.Quaternion;
import com.jme3.math.Vector2f;
import com.jme3.math.Vector3f;
import com.jme3.renderer.Camera;
import com.jme3.scene.Spatial;
import com.jme3.system.osvr.osvrclientkit.OsvrClientKitLibrary;
import com.jme3.system.osvr.osvrclientkit.OsvrClientKitLibrary.OSVR_ClientInterface;
import com.jme3.system.osvr.osvrclientreporttypes.OSVR_AnalogReport;
import com.jme3.system.osvr.osvrclientreporttypes.OSVR_ButtonReport;
import com.jme3.system.osvr.osvrclientreporttypes.OSVR_Pose3;
import com.jme3.system.osvr.osvrinterface.OsvrInterfaceLibrary;
import com.jme3.system.osvr.osvrtimevalue.OSVR_TimeValue;
import com.sun.jna.Callback;
import com.sun.jna.Pointer;
import com.sun.jna.ptr.PointerByReference;
/**
* A class that wraps an OSVR input.
* @author reden - phr00t - https://github.com/phr00t
* @author Julien Seinturier - COMEX SA - http://www.seinturier.fr
*/
public class OSVRInput implements VRInputAPI {
private static final Logger logger = Logger.getLogger(OSVRInput.class.getName());
// position example: https://github.com/OSVR/OSVR-Core/blob/master/examples/clients/TrackerState.c
// button example: https://github.com/OSVR/OSVR-Core/blob/master/examples/clients/ButtonCallback.c
// analog example: https://github.com/OSVR/OSVR-Core/blob/master/examples/clients/AnalogCallback.c
private static final int ANALOG_COUNT = 3, BUTTON_COUNT = 7, CHANNEL_COUNT = 3;
OSVR_ClientInterface[][] buttons;
OSVR_ClientInterface[][][] analogs;
OSVR_ClientInterface[] hands;
OSVR_Pose3[] handState;
Callback buttonHandler, analogHandler;
OSVR_TimeValue tv = new OSVR_TimeValue();
boolean[] isHandTracked = new boolean[2];
private float[][][] analogState;
private float[][] buttonState;
private final Quaternion tempq = new Quaternion();
private final Vector3f tempv = new Vector3f();
private final Vector2f temp2 = new Vector2f();
private final boolean[][] buttonDown = new boolean[16][16];
private static final Vector2f temp2Axis = new Vector2f();
private static final Vector2f lastCallAxis[] = new Vector2f[16];
private static float axisMultiplier = 1f;
private VREnvironment environment = null;
/**
* Get the system String that identifies a controller.
* @param left is the controller is the left one (false
if the right controller is needed).
* @param index the index of the controller.
* @return the system String that identifies the controller.
*/
public static byte[] getButtonString(boolean left, byte index) {
if( left ) {
return new byte[] { '/', 'c', 'o', 'n', 't', 'r', 'o', 'l', 'l', 'e', 'r', '/', 'l', 'e', 'f', 't', '/', index, (byte)0 };
}
return new byte[] { '/', 'c', 'o', 'n', 't', 'r', 'o', 'l', 'l', 'e', 'r', '/', 'r', 'i', 'g', 'h', 't', '/', index, (byte)0 };
}
/**
* The left-hand system String.
*/
public static byte[] leftHand = { '/', 'm', 'e', '/', 'h', 'a', 'n', 'd', 's', '/', 'l', 'e', 'f', 't', (byte)0 };
/**
* The right-hand system String.
*/
public static byte[] rightHand = { '/', 'm', 'e', '/', 'h', 'a', 'n', 'd', 's', '/', 'r', 'i', 'g', 'h', 't', (byte)0 };
/**
* Create a new OSVR input attached to the given {@link VREnvironment VR environment}.
* @param environment the {@link VREnvironment VR environment} to which the input is attached.
*/
public OSVRInput(VREnvironment environment){
this.environment = environment;
}
@Override
public boolean isButtonDown(int controllerIndex, VRInputType checkButton) {
return buttonState[controllerIndex][checkButton.getValue()] != 0f;
}
@Override
public boolean wasButtonPressedSinceLastCall(int controllerIndex, VRInputType checkButton) {
boolean buttonDownNow = isButtonDown(controllerIndex, checkButton);
int checkButtonValue = checkButton.getValue();
boolean retval = buttonDownNow == true && buttonDown[controllerIndex][checkButtonValue] == false;
buttonDown[controllerIndex][checkButtonValue] = buttonDownNow;
return retval;
}
@Override
public void resetInputSinceLastCall() {
for(int i=0;i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy