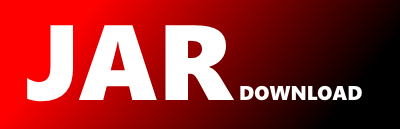
com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-vr Show documentation
Show all versions of jme3-vr Show documentation
jMonkeyEngine is a 3-D game engine for adventurous Java developers
package com.jme3.system.osvr.osvrdisplay;
import com.jme3.system.osvr.osvrclientkit.OsvrClientKitLibrary;
import com.sun.jna.Library;
import com.sun.jna.Native;
import com.sun.jna.NativeLibrary;
import com.sun.jna.Pointer;
import com.sun.jna.PointerType;
import com.sun.jna.ptr.DoubleByReference;
import com.sun.jna.ptr.FloatByReference;
import com.sun.jna.ptr.IntByReference;
import com.sun.jna.ptr.PointerByReference;
import java.nio.ByteBuffer;
import java.nio.DoubleBuffer;
import java.nio.FloatBuffer;
import java.nio.IntBuffer;
/**
* JNA Wrapper for library osvrDisplay
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java , Rococoa, or JNA.
*/
public class OsvrDisplayLibrary implements Library {
public static final String JNA_LIBRARY_NAME = "osvrClientKit";
public static final NativeLibrary JNA_NATIVE_LIB = NativeLibrary.getInstance(OsvrDisplayLibrary.JNA_LIBRARY_NAME);
static {
Native.register(OsvrDisplayLibrary.class, OsvrDisplayLibrary.JNA_NATIVE_LIB);
}
/**
* Allocates a display configuration object populated with data from the
* OSVR system.
* Before this call will succeed, your application will need to be correctly
* and fully connected to an OSVR server. You may consider putting this call in
* a loop alternating with osvrClientUpdate() until this call succeeds.
* Data provided by a display configuration object:
* - The logical display topology (number and relationship of viewers, eyes,
* and surfaces), which remains constant throughout the life of the
* configuration object. (A method of notification of change here is TBD).
* - Pose data for viewers (not required for rendering) and pose/view data for
* eyes (used for rendering) which is based on tracker data: if used, these
* should be queried every frame.
* - Projection matrix data for surfaces, which while in current practice may
* be relatively unchanging, we are not guaranteeing them to be constant:
* these should be queried every frame.
* - Video-input-relative viewport size/location for a surface: would like this
* to be variable, but probably not feasible. If you have input, please
* comment on the dev mailing list.
* - Per-surface distortion strategy priorities/availabilities: constant. Note
* the following, though...
* - Per-surface distortion strategy parameters: variable, request each frame.
* (Could make constant with a notification if needed?)
* Important note: While most of this data is immediately available if you are
* successful in getting a display config object, the pose-based data (viewer
* pose, eye pose, eye view matrix) needs tracker state, so at least one (and in
* practice, typically more) osvrClientUpdate() must be performed before a new
* tracker report is available to populate that state. See
* osvrClientCheckDisplayStartup() to query if all startup data is available.
* todo Decide if relative viewport should be constant in a display config,
* and update docs accordingly.
* todo Decide if distortion params should be constant in a display config,
* and update docs accordingly.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or some other
* error occurred, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetDisplay(OSVR_ClientContext, OSVR_DisplayConfig*)
* @deprecated use the safer method
* {@link #osvrClientGetDisplay(com.jme3.system.osvr.osvrclientkit.OsvrClientKitLibrary.OSVR_ClientContext, com.sun.jna.ptr.PointerByReference)}
* instead
*/
@Deprecated
public static native byte osvrClientGetDisplay(Pointer ctx, Pointer disp);
/**
* Allocates a display configuration object populated with data from the
* OSVR system.
* Before this call will succeed, your application will need to be correctly
* and fully connected to an OSVR server. You may consider putting this call in
* a loop alternating with osvrClientUpdate() until this call succeeds.
* Data provided by a display configuration object:
* - The logical display topology (number and relationship of viewers, eyes,
* and surfaces), which remains constant throughout the life of the
* configuration object. (A method of notification of change here is TBD).
* - Pose data for viewers (not required for rendering) and pose/view data for
* eyes (used for rendering) which is based on tracker data: if used, these
* should be queried every frame.
* - Projection matrix data for surfaces, which while in current practice may
* be relatively unchanging, we are not guaranteeing them to be constant:
* these should be queried every frame.
* - Video-input-relative viewport size/location for a surface: would like this
* to be variable, but probably not feasible. If you have input, please
* comment on the dev mailing list.
* - Per-surface distortion strategy priorities/availabilities: constant. Note
* the following, though...
* - Per-surface distortion strategy parameters: variable, request each frame.
* (Could make constant with a notification if needed?)
* Important note: While most of this data is immediately available if you are
* successful in getting a display config object, the pose-based data (viewer
* pose, eye pose, eye view matrix) needs tracker state, so at least one (and in
* practice, typically more) osvrClientUpdate() must be performed before a new
* tracker report is available to populate that state. See
* osvrClientCheckDisplayStartup() to query if all startup data is available.
* todo Decide if relative viewport should be constant in a display config,
* and update docs accordingly.
* todo Decide if distortion params should be constant in a display config,
* and update docs accordingly.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or some other
* error occurred, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetDisplay(OSVR_ClientContext, OSVR_DisplayConfig*)
*/
public static native byte osvrClientGetDisplay(OsvrClientKitLibrary.OSVR_ClientContext ctx, PointerByReference disp);
/**
* Allocates a display configuration object populated with data from the
* OSVR system.
* Before this call will succeed, your application will need to be correctly
* and fully connected to an OSVR server. You may consider putting this call in
* a loop alternating with osvrClientUpdate() until this call succeeds.
* Data provided by a display configuration object:
* - The logical display topology (number and relationship of viewers, eyes,
* and surfaces), which remains constant throughout the life of the
* configuration object. (A method of notification of change here is TBD).
* - Pose data for viewers (not required for rendering) and pose/view data for
* eyes (used for rendering) which is based on tracker data: if used, these
* should be queried every frame.
* - Projection matrix data for surfaces, which while in current practice may
* be relatively unchanging, we are not guaranteeing them to be constant:
* these should be queried every frame.
* - Video-input-relative viewport size/location for a surface: would like this
* to be variable, but probably not feasible. If you have input, please
* comment on the dev mailing list.
* - Per-surface distortion strategy priorities/availabilities: constant. Note
* the following, though...
* - Per-surface distortion strategy parameters: variable, request each frame.
* (Could make constant with a notification if needed?)
* Important note: While most of this data is immediately available if you are
* successful in getting a display config object, the pose-based data (viewer
* pose, eye pose, eye view matrix) needs tracker state, so at least one (and in
* practice, typically more) osvrClientUpdate() must be performed before a new
* tracker report is available to populate that state. See
* osvrClientCheckDisplayStartup() to query if all startup data is available.
* todo Decide if relative viewport should be constant in a display config,
* and update docs accordingly.
* todo Decide if distortion params should be constant in a display config,
* and update docs accordingly.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or some other
* error occurred, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetDisplay(OSVR_ClientContext, OSVR_DisplayConfig*)
*/
public static native byte osvrClientGetDisplay(Pointer ctx, PointerByReference disp);
/**
* Frees a display configuration object. The corresponding context must
* still be open.
* If you fail to call this, it will be automatically called as part of
* clean-up when the corresponding context is closed.
* @return OSVR_RETURN_FAILURE if a null config was passed, or if the given
* display object was already freed.
* Original signature : OSVR_ReturnCode osvrClientFreeDisplay(OSVR_DisplayConfig)
* @deprecated use the safer method
* {@link #osvrClientFreeDisplay(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig)}
* instead
*/
@Deprecated
public static native byte osvrClientFreeDisplay(Pointer disp);
/**
* Frees a display configuration object. The corresponding context must
* still be open.
* If you fail to call this, it will be automatically called as part of
* clean-up when the corresponding context is closed.
* @return OSVR_RETURN_FAILURE if a null config was passed, or if the given
* display object was already freed.
* Original signature : OSVR_ReturnCode osvrClientFreeDisplay(OSVR_DisplayConfig)
*/
public static native byte osvrClientFreeDisplay(OsvrDisplayLibrary.OSVR_DisplayConfig disp);
/**
* Checks to see if a display is fully configured and ready, including
* having received its first pose update.
* Once this first succeeds, it will continue to succeed for the lifetime of
* the display config object, so it is not necessary to keep calling once you
* get a successful result.
* @return OSVR_RETURN_FAILURE if a null config was passed, or if the given
* display config object was otherwise not ready for full use.
* Original signature : OSVR_ReturnCode osvrClientCheckDisplayStartup(OSVR_DisplayConfig)
* @deprecated use the safer method
* {@link #osvrClientCheckDisplayStartup(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig)}
* instead
*/
@Deprecated
public static native byte osvrClientCheckDisplayStartup(Pointer disp);
/**
* Checks to see if a display is fully configured and ready, including
* having received its first pose update.
* Once this first succeeds, it will continue to succeed for the lifetime of
* the display config object, so it is not necessary to keep calling once you
* get a successful result.
* @return OSVR_RETURN_FAILURE if a null config was passed, or if the given
* display config object was otherwise not ready for full use.
* Original signature : OSVR_ReturnCode osvrClientCheckDisplayStartup(OSVR_DisplayConfig)
*/
public static native byte osvrClientCheckDisplayStartup(OsvrDisplayLibrary.OSVR_DisplayConfig disp);
/**
* A display config can have one or more display inputs to pass pixels
* over (HDMI/DVI connections, etcetera): retrieve the number of display inputs in
* the current configuration.
* @param disp Display config object.
* @param numDisplayInputs Number of display inputs in the logical display
* topology, **constant** throughout the active, valid lifetime of a display
* config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in
* which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumDisplayInputs(OSVR_DisplayConfig, OSVR_DisplayInputCount*)
* @deprecated use the safer method
* {@link #osvrClientGetNumDisplayInputs(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, java.nio.ByteBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetNumDisplayInputs(Pointer disp, Pointer numDisplayInputs);
/**
* A display config can have one or more display inputs to pass pixels
* over (HDMI/DVI connections, etcetera): retrieve the number of display inputs in
* the current configuration.
* @param disp Display config object.
* @param numDisplayInputs Number of display inputs in the logical display
* topology, **constant** throughout the active, valid lifetime of a display
* config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in
* which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumDisplayInputs(OSVR_DisplayConfig, OSVR_DisplayInputCount*)
*/
public static native byte osvrClientGetNumDisplayInputs(OsvrDisplayLibrary.OSVR_DisplayConfig disp, ByteBuffer numDisplayInputs);
/**
* Retrieve the pixel dimensions of a given display input for a display
* config
* @param disp Display config object.
* @param displayInputIndex The zero-based index of the display input.
* @param width Width (in pixels) of the display input.
* @param height Height (in pixels) of the display input.
* The out parameters are **constant** throughout the active, valid lifetime of
* a display config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in
* which case the output arguments are unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetDisplayDimensions(OSVR_DisplayConfig, OSVR_DisplayInputCount, OSVR_DisplayDimension*, OSVR_DisplayDimension*)
* @deprecated use the safer method
* {@link #osvrClientGetDisplayDimensions(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, byte, java.nio.IntBuffer, java.nio.IntBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetDisplayDimensions(Pointer disp, byte displayInputIndex, IntByReference width, IntByReference height);
/**
* Retrieve the pixel dimensions of a given display input for a display
* config
* @param disp Display config object.
* @param displayInputIndex The zero-based index of the display input.
* @param width Width (in pixels) of the display input.
* @param height Height (in pixels) of the display input.
* The out parameters are **constant** throughout the active, valid lifetime of
* a display config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in
* which case the output arguments are unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetDisplayDimensions(OSVR_DisplayConfig, OSVR_DisplayInputCount, OSVR_DisplayDimension*, OSVR_DisplayDimension*)
*/
public static native byte osvrClientGetDisplayDimensions(OsvrDisplayLibrary.OSVR_DisplayConfig disp, byte displayInputIndex, IntBuffer width, IntBuffer height);
/**
* A display config can have one (or theoretically more) viewers:
* retrieve the viewer count.
* @param disp Display config object.
* @param viewers Number of viewers in the logical display topology,
* *constant** throughout the active, valid lifetime of a display config
* object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumViewers(OSVR_DisplayConfig, OSVR_ViewerCount*)
* @deprecated use the safer method
* {@link #osvrClientGetNumViewers(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, java.nio.IntBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetNumViewers(Pointer disp, IntByReference viewers);
/**
* A display config can have one (or theoretically more) viewers:
* retrieve the viewer count.
* @param disp Display config object.
* @param viewers Number of viewers in the logical display topology,
* *constant** throughout the active, valid lifetime of a display config
* object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumViewers(OSVR_DisplayConfig, OSVR_ViewerCount*)
*/
public static native byte osvrClientGetNumViewers(OsvrDisplayLibrary.OSVR_DisplayConfig disp, IntBuffer viewers);
/**
* Get the pose of a viewer in a display config.
* Note that there may not necessarily be any surfaces rendered from this pose
* (it's the unused "center" eye in a stereo configuration, for instance) so
* only use this if it makes integration into your engine or existing
* applications (not originally designed for stereo) easier.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the pose argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerPose(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_Pose3*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerPose(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, com.sun.jna.Pointer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerPose(Pointer disp, int viewer, Pointer pose);
/**
* Get the pose of a viewer in a display config.
* Note that there may not necessarily be any surfaces rendered from this pose
* (it's the unused "center" eye in a stereo configuration, for instance) so
* only use this if it makes integration into your engine or existing
* applications (not originally designed for stereo) easier.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the pose argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerPose(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_Pose3*)
*/
public static native byte osvrClientGetViewerPose(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, Pointer pose);
/**
* Each viewer in a display config can have one or more "eyes" which
* have a substantially similar pose: get the count.
* @param disp Display config object.
* @param viewer Viewer ID
* @param eyes Number of eyes for this viewer in the logical display
* topology, **constant** throughout the active, valid lifetime of a display
* config object
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumEyesForViewer(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount*)
* @deprecated use the safer method
* {@link #osvrClientGetNumEyesForViewer(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, java.nio.ByteBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetNumEyesForViewer(Pointer disp, int viewer, Pointer eyes);
/**
* Each viewer in a display config can have one or more "eyes" which
* have a substantially similar pose: get the count.
* @param disp Display config object.
* @param viewer Viewer ID
* @param eyes Number of eyes for this viewer in the logical display
* topology, **constant** throughout the active, valid lifetime of a display
* config object
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumEyesForViewer(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount*)
*/
public static native byte osvrClientGetNumEyesForViewer(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, ByteBuffer eyes);
/**
* Get the "viewpoint" for the given eye of a viewer in a display
* config.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param pose Room-space pose (not relative to pose of the viewer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the pose argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyePose(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_Pose3*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyePose(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, com.sun.jna.Pointer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyePose(Pointer disp, int viewer, byte eye, Pointer pose);
/**
* Get the "viewpoint" for the given eye of a viewer in a display
* config.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param pose Room-space pose (not relative to pose of the viewer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the pose argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyePose(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_Pose3*)
*/
public static native byte osvrClientGetViewerEyePose(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, Pointer pose);
/**
* Get the view matrix (inverse of pose) for the given eye of a
* viewer in a display config - matrix of **doubles**.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param mat Pass a double[::OSVR_MATRIX_SIZE] to get the transformation
* matrix from room space to eye space (not relative to pose of the viewer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeViewMatrixd(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_MatrixConventions, double*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeViewMatrixd(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, short, java.nio.DoubleBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeViewMatrixd(Pointer disp, int viewer, byte eye, short flags, DoubleByReference mat);
/**
* Get the view matrix (inverse of pose) for the given eye of a
* viewer in a display config - matrix of **doubles**.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param mat Pass a double[::OSVR_MATRIX_SIZE] to get the transformation
* matrix from room space to eye space (not relative to pose of the viewer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeViewMatrixd(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_MatrixConventions, double*)
*/
public static native byte osvrClientGetViewerEyeViewMatrixd(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, short flags, DoubleBuffer mat);
/**
* Get the view matrix (inverse of pose) for the given eye of a
* viewer in a display config - matrix of **floats**.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param mat Pass a float[::OSVR_MATRIX_SIZE] to get the transformation
* matrix from room space to eye space (not relative to pose of the viewer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeViewMatrixf(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_MatrixConventions, float*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeViewMatrixf(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, short, java.nio.FloatBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeViewMatrixf(Pointer disp, int viewer, byte eye, short flags, FloatByReference mat);
/**
* Get the view matrix (inverse of pose) for the given eye of a
* viewer in a display config - matrix of **floats**.
* Will only succeed if osvrClientCheckDisplayStartup() succeeds.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param mat Pass a float[::OSVR_MATRIX_SIZE] to get the transformation
* matrix from room space to eye space (not relative to pose of the viewer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed or no pose was
* yet available, in which case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeViewMatrixf(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_MatrixConventions, float*)
*/
public static native byte osvrClientGetViewerEyeViewMatrixf(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, short flags, FloatBuffer mat);
/**
* Each eye of each viewer in a display config has one or more surfaces
* (aka "screens") on which content should be rendered.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surfaces Number of surfaces (numbered [0, surfaces - 1]) for the
* given viewer and eye. **Constant** throughout the active, valid lifetime of
* a display config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumSurfacesForViewerEye(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount*)
* @deprecated use the safer method
* {@link #osvrClientGetNumSurfacesForViewerEye(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, java.nio.IntBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetNumSurfacesForViewerEye(Pointer disp, int viewer, byte eye, IntByReference surfaces);
/**
* Each eye of each viewer in a display config has one or more surfaces
* (aka "screens") on which content should be rendered.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surfaces Number of surfaces (numbered [0, surfaces - 1]) for the
* given viewer and eye. **Constant** throughout the active, valid lifetime of
* a display config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetNumSurfacesForViewerEye(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount*)
*/
public static native byte osvrClientGetNumSurfacesForViewerEye(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, IntBuffer surfaces);
/**
* Get the dimensions/location of the viewport **within the display
* input** for a surface seen by an eye of a viewer in a display config. (This
* does not include other video inputs that may be on a single virtual desktop,
* etc. or explicitly account for display configurations that use multiple
* video inputs. It does not necessarily indicate that a viewport in the sense
* of glViewport must be created with these parameters, though the parameter
* order matches for convenience.)
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param left Output: Distance in pixels from the left of the video input
* to the left of the viewport.
* @param bottom Output: Distance in pixels from the bottom of the video
* input to the bottom of the viewport.
* @param width Output: Width of viewport in pixels.
* @param height Output: Height of viewport in pixels.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output arguments are unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetRelativeViewportForViewerEyeSurface(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_ViewportDimension*, OSVR_ViewportDimension*, OSVR_ViewportDimension*, OSVR_ViewportDimension*)
* @deprecated use the safer method
* {@link #osvrClientGetRelativeViewportForViewerEyeSurface(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.IntBuffer, java.nio.IntBuffer, java.nio.IntBuffer, java.nio.IntBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetRelativeViewportForViewerEyeSurface(Pointer disp, int viewer, byte eye, int surface, IntByReference left, IntByReference bottom, IntByReference width, IntByReference height);
/**
* Get the dimensions/location of the viewport **within the display
* input** for a surface seen by an eye of a viewer in a display config. (This
* does not include other video inputs that may be on a single virtual desktop,
* etc. or explicitly account for display configurations that use multiple
* video inputs. It does not necessarily indicate that a viewport in the sense
* of glViewport must be created with these parameters, though the parameter
* order matches for convenience.)
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param left Output: Distance in pixels from the left of the video input
* to the left of the viewport.
* @param bottom Output: Distance in pixels from the bottom of the video
* input to the bottom of the viewport.
* @param width Output: Width of viewport in pixels.
* @param height Output: Height of viewport in pixels.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output arguments are unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetRelativeViewportForViewerEyeSurface(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_ViewportDimension*, OSVR_ViewportDimension*, OSVR_ViewportDimension*, OSVR_ViewportDimension*)
*/
public static native byte osvrClientGetRelativeViewportForViewerEyeSurface(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, IntBuffer left, IntBuffer bottom, IntBuffer width, IntBuffer height);
/**
* Get the index of the display input for a surface seen by an eye of a
* viewer in a display config.
* This is the OSVR-assigned display input: it may not (and in practice,
* usually will not) match any platform-specific display indices. This function
* exists to associate surfaces with video inputs as enumerated by
* osvrClientGetNumDisplayInputs().
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param displayInput Zero-based index of the display input pixels for
* this surface are transmitted over.
* This association is **constant** throughout the active, valid lifetime of a
* display config object.
* @see #osvrClientGetNumDisplayInputs(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, java.nio.ByteBuffer)
* @see #osvrClientGetRelativeViewportForViewerEyeSurface(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.IntBuffer, java.nio.IntBuffer, java.nio.IntBuffer, java.nio.IntBuffer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which
* case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceDisplayInputIndex(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_DisplayInputCount*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeSurfaceDisplayInputIndex(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.ByteBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeSurfaceDisplayInputIndex(Pointer disp, int viewer, byte eye, int surface, Pointer displayInput);
/**
* Get the index of the display input for a surface seen by an eye of a
* viewer in a display config.
* This is the OSVR-assigned display input: it may not (and in practice,
* usually will not) match any platform-specific display indices. This function
* exists to associate surfaces with video inputs as enumerated by
* osvrClientGetNumDisplayInputs().
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param displayInput Zero-based index of the display input pixels for
* this surface are transmitted over.
* This association is **constant** throughout the active, valid lifetime of a
* display config object.
* @see #osvrClientGetNumDisplayInputs(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, java.nio.ByteBuffer)
* @see #osvrClientGetRelativeViewportForViewerEyeSurface(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.IntBuffer, java.nio.IntBuffer, java.nio.IntBuffer, java.nio.IntBuffer)
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which
* case the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceDisplayInputIndex(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_DisplayInputCount*)
*/
public static native byte osvrClientGetViewerEyeSurfaceDisplayInputIndex(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, ByteBuffer displayInput);
/**
* Get the projection matrix for a surface seen by an eye of a viewer
* in a display config. (double version)
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param near Distance from viewpoint to near clipping plane - must be
* positive.
* @param far Distance from viewpoint to far clipping plane - must be positive
* and not equal to near, typically greater than near.
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param matrix Output projection matrix: supply an array of 16
* (::OSVR_MATRIX_SIZE) doubles.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceProjectionMatrixd(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, double, double, OSVR_MatrixConventions, double*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeSurfaceProjectionMatrixd(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, double, double, short, java.nio.DoubleBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeSurfaceProjectionMatrixd(Pointer disp, int viewer, byte eye, int surface, double near, double far, short flags, DoubleByReference matrix);
/**
* Get the projection matrix for a surface seen by an eye of a viewer
* in a display config. (double version)
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param near Distance from viewpoint to near clipping plane - must be
* positive.
* @param far Distance from viewpoint to far clipping plane - must be positive
* and not equal to near, typically greater than near.
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param matrix Output projection matrix: supply an array of 16
* (::OSVR_MATRIX_SIZE) doubles.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceProjectionMatrixd(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, double, double, OSVR_MatrixConventions, double*)
*/
public static native byte osvrClientGetViewerEyeSurfaceProjectionMatrixd(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, double near, double far, short flags, DoubleBuffer matrix);
/**
* Get the projection matrix for a surface seen by an eye of a viewer
* in a display config. (float version)
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param near Distance to near clipping plane - must be nonzero, typically
* positive.
* @param far Distance to far clipping plane - must be nonzero, typically
* positive and greater than near.
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param matrix Output projection matrix: supply an array of 16
* (::OSVR_MATRIX_SIZE) floats.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceProjectionMatrixf(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, float, float, OSVR_MatrixConventions, float*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeSurfaceProjectionMatrixf(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, float, float, short, java.nio.FloatBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeSurfaceProjectionMatrixf(Pointer disp, int viewer, byte eye, int surface, float near, float far, short flags, FloatByReference matrix);
/**
* Get the projection matrix for a surface seen by an eye of a viewer
* in a display config. (float version)
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param near Distance to near clipping plane - must be nonzero, typically
* positive.
* @param far Distance to far clipping plane - must be nonzero, typically
* positive and greater than near.
* @param flags Bitwise OR of matrix convention flags (see @ref MatrixFlags)
* @param matrix Output projection matrix: supply an array of 16
* (::OSVR_MATRIX_SIZE) floats.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceProjectionMatrixf(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, float, float, OSVR_MatrixConventions, float*)
*/
public static native byte osvrClientGetViewerEyeSurfaceProjectionMatrixf(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, float near, float far, short flags, FloatBuffer matrix);
/**
* Get the clipping planes (positions at unit distance) for a surface
* seen by an eye of a viewer
* in a display config.
* This is only for use in integrations that cannot accept a fully-formulated
* projection matrix as returned by
* osvrClientGetViewerEyeSurfaceProjectionMatrixf() or
* osvrClientGetViewerEyeSurfaceProjectionMatrixd(), and may not necessarily
* provide the same optimizations.
* As all the planes are given at unit (1) distance, before passing these
* planes to a consuming function in your application/engine, you will typically
* divide them by your near clipping plane distance.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param left Distance to left clipping plane
* @param right Distance to right clipping plane
* @param bottom Distance to bottom clipping plane
* @param top Distance to top clipping plane
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output arguments are unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceProjectionClippingPlanes(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, double*, double*, double*, double*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeSurfaceProjectionClippingPlanes(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.DoubleBuffer, java.nio.DoubleBuffer, java.nio.DoubleBuffer, java.nio.DoubleBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeSurfaceProjectionClippingPlanes(Pointer disp, int viewer, byte eye, int surface, DoubleByReference left, DoubleByReference right, DoubleByReference bottom, DoubleByReference top);
/**
* Get the clipping planes (positions at unit distance) for a surface
* seen by an eye of a viewer
* in a display config.
* This is only for use in integrations that cannot accept a fully-formulated
* projection matrix as returned by
* osvrClientGetViewerEyeSurfaceProjectionMatrixf() or
* osvrClientGetViewerEyeSurfaceProjectionMatrixd(), and may not necessarily
* provide the same optimizations.
* As all the planes are given at unit (1) distance, before passing these
* planes to a consuming function in your application/engine, you will typically
* divide them by your near clipping plane distance.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param left Distance to left clipping plane
* @param right Distance to right clipping plane
* @param bottom Distance to bottom clipping plane
* @param top Distance to top clipping plane
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output arguments are unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceProjectionClippingPlanes(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, double*, double*, double*, double*)
*/
public static native byte osvrClientGetViewerEyeSurfaceProjectionClippingPlanes(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, DoubleBuffer left, DoubleBuffer right, DoubleBuffer bottom, DoubleBuffer top);
/**
* Determines if a surface seen by an eye of a viewer in a display
* config requests some distortion to be performed.
* This simply reports true or false, and does not specify which kind of
* distortion implementations have been parameterized for this display. For
* each distortion implementation your application supports, you'll want to
* call the corresponding priority function to find out if it is available.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param distortionRequested Output parameter: whether distortion is
* requested. **Constant** throughout the active, valid lifetime of a display
* config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientDoesViewerEyeSurfaceWantDistortion(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_CBool*)
* @deprecated use the safer method
* {@link #osvrClientDoesViewerEyeSurfaceWantDistortion(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.ByteBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientDoesViewerEyeSurfaceWantDistortion(Pointer disp, int viewer, byte eye, int surface, Pointer distortionRequested);
/**
* Determines if a surface seen by an eye of a viewer in a display
* config requests some distortion to be performed.
* This simply reports true or false, and does not specify which kind of
* distortion implementations have been parameterized for this display. For
* each distortion implementation your application supports, you'll want to
* call the corresponding priority function to find out if it is available.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param distortionRequested Output parameter: whether distortion is
* requested. **Constant** throughout the active, valid lifetime of a display
* config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientDoesViewerEyeSurfaceWantDistortion(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_CBool*)
*/
public static native byte osvrClientDoesViewerEyeSurfaceWantDistortion(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, ByteBuffer distortionRequested);
/**
* Returns the priority/availability of radial distortion parameters for
* a surface seen by an eye of a viewer in a display config.
* If osvrClientDoesViewerEyeSurfaceWantDistortion() reports false, then the
* display does not request distortion of any sort, and thus neither this nor
* any other distortion strategy priority function will report an "available"
* priority.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param priority Output: the priority level. Negative values
* (canonically OSVR_DISTORTION_PRIORITY_UNAVAILABLE) indicate this technique
* not available, higher values indicate higher preference for the given
* technique based on the device's description. **Constant** throughout the
* active, valid lifetime of a display config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceRadialDistortionPriority(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_DistortionPriority*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeSurfaceRadialDistortionPriority(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, java.nio.IntBuffer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeSurfaceRadialDistortionPriority(Pointer disp, int viewer, byte eye, int surface, IntByReference priority);
/**
* Returns the priority/availability of radial distortion parameters for
* a surface seen by an eye of a viewer in a display config.
* If osvrClientDoesViewerEyeSurfaceWantDistortion() reports false, then the
* display does not request distortion of any sort, and thus neither this nor
* any other distortion strategy priority function will report an "available"
* priority.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param priority Output: the priority level. Negative values
* (canonically OSVR_DISTORTION_PRIORITY_UNAVAILABLE) indicate this technique
* not available, higher values indicate higher preference for the given
* technique based on the device's description. **Constant** throughout the
* active, valid lifetime of a display config object.
* @return OSVR_RETURN_FAILURE if invalid parameters were passed, in which case
* the output argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceRadialDistortionPriority(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_DistortionPriority*)
*/
public static native byte osvrClientGetViewerEyeSurfaceRadialDistortionPriority(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, IntBuffer priority);
/**
* Returns the radial distortion parameters, if known/requested, for a
* surface seen by an eye of a viewer in a display config.
* Will only succeed if osvrClientGetViewerEyeSurfaceRadialDistortionPriority()
* reports a non-negative priority.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param params Output: the parameters for radial distortion
* @return OSVR_RETURN_FAILURE if this surface does not have these parameters
* described, or if invalid parameters were passed, in which case the output
* argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceRadialDistortion(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_RadialDistortionParameters*)
* @deprecated use the safer method
* {@link #osvrClientGetViewerEyeSurfaceRadialDistortion(com.jme3.system.osvr.osvrdisplay.OsvrDisplayLibrary.OSVR_DisplayConfig, int, byte, int, com.sun.jna.Pointer)}
* instead
*/
@Deprecated
public static native byte osvrClientGetViewerEyeSurfaceRadialDistortion(Pointer disp, int viewer, byte eye, int surface, Pointer params);
/**
* Returns the radial distortion parameters, if known/requested, for a
* surface seen by an eye of a viewer in a display config.
* Will only succeed if osvrClientGetViewerEyeSurfaceRadialDistortionPriority()
* reports a non-negative priority.
* @param disp Display config object
* @param viewer Viewer ID
* @param eye Eye ID
* @param surface Surface ID
* @param params Output: the parameters for radial distortion
* @return OSVR_RETURN_FAILURE if this surface does not have these parameters
* described, or if invalid parameters were passed, in which case the output
* argument is unmodified.
* Original signature : OSVR_ReturnCode osvrClientGetViewerEyeSurfaceRadialDistortion(OSVR_DisplayConfig, OSVR_ViewerCount, OSVR_EyeCount, OSVR_SurfaceCount, OSVR_RadialDistortionParameters*)
*/
public static native byte osvrClientGetViewerEyeSurfaceRadialDistortion(OsvrDisplayLibrary.OSVR_DisplayConfig disp, int viewer, byte eye, int surface, Pointer params);
public static class OSVR_ClientContext extends PointerType {
public OSVR_ClientContext(Pointer address) {
super(address);
}
public OSVR_ClientContext() {
super();
}
};
public static class OSVR_DisplayConfig extends PointerType {
public OSVR_DisplayConfig(Pointer address) {
super(address);
}
public OSVR_DisplayConfig() {
super();
}
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy