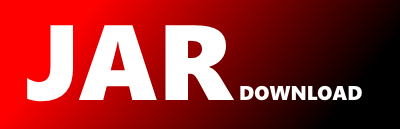
com.jme3.system.osvr.osvrrendermanageropengl.OSVR_OpenGLToolkitFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-vr Show documentation
Show all versions of jme3-vr Show documentation
jMonkeyEngine is a 3-D game engine for adventurous Java developers
package com.jme3.system.osvr.osvrrendermanageropengl;
import com.ochafik.lang.jnaerator.runtime.NativeSize;
import com.sun.jna.Callback;
import com.sun.jna.Pointer;
import com.sun.jna.Structure;
import com.sun.jna.ptr.IntByReference;
import java.util.Arrays;
import java.util.List;
/**
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java , Rococoa, or JNA.
*/
public class OSVR_OpenGLToolkitFunctions extends Structure {
public NativeSize size;
/** C type : void* */
public Pointer data;
/** C type : create_callback* */
public OSVR_OpenGLToolkitFunctions.create_callback create;
/** C type : destroy_callback* */
public OSVR_OpenGLToolkitFunctions.destroy_callback destroy;
/** C type : handleEvents_callback* */
public OSVR_OpenGLToolkitFunctions.handleEvents_callback handleEvents;
/** C type : getDisplayFrameBuffer_callback* */
public OSVR_OpenGLToolkitFunctions.getDisplayFrameBuffer_callback getDisplayFrameBuffer;
/** C type : getDisplaySizeOverride_callback* */
public OSVR_OpenGLToolkitFunctions.getDisplaySizeOverride_callback getDisplaySizeOverride;
public interface create_callback extends Callback {
void apply(Pointer data);
};
public interface destroy_callback extends Callback {
void apply(Pointer data);
};
public interface OSVR_CBool_callback extends Callback {
int apply(Pointer data, OSVR_OpenGLContextParams p);
};
public interface OSVR_CBool_callback2 extends Callback {
int apply(Pointer data);
};
public interface OSVR_CBool_callback3 extends Callback {
int apply(Pointer data, NativeSize display);
};
public interface OSVR_CBool_callback4 extends Callback {
int apply(Pointer data, NativeSize display);
};
public interface OSVR_CBool_callback5 extends Callback {
int apply(Pointer data, byte verticalSync);
};
public interface handleEvents_callback extends Callback {
byte apply(Pointer data);
};
public interface getDisplayFrameBuffer_callback extends Callback {
byte apply(Pointer data, NativeSize display, IntByReference frameBufferOut);
};
public interface getDisplaySizeOverride_callback extends Callback {
byte apply(Pointer data, NativeSize display, IntByReference width, IntByReference height);
};
public OSVR_OpenGLToolkitFunctions() {
super();
}
@Override
protected List getFieldOrder() {
return Arrays.asList("size", "data", "create", "destroy", "handleEvents", "getDisplayFrameBuffer", "getDisplaySizeOverride");
}
/**
* @param data C type : void*
* @param create C type : create_callback*
* @param destroy C type : destroy_callback*
* @param handleEvents C type : handleEvents_callback*
* @param getDisplayFrameBuffer C type : getDisplayFrameBuffer_callback*
* @param getDisplaySizeOverride C type : getDisplaySizeOverride_callback*
*/
public OSVR_OpenGLToolkitFunctions(NativeSize size, Pointer data, OSVR_OpenGLToolkitFunctions.create_callback create, OSVR_OpenGLToolkitFunctions.destroy_callback destroy, OSVR_OpenGLToolkitFunctions.handleEvents_callback handleEvents, OSVR_OpenGLToolkitFunctions.getDisplayFrameBuffer_callback getDisplayFrameBuffer, OSVR_OpenGLToolkitFunctions.getDisplaySizeOverride_callback getDisplaySizeOverride) {
super();
this.size = size;
this.data = data;
this.create = create;
this.destroy = destroy;
this.handleEvents = handleEvents;
this.getDisplayFrameBuffer = getDisplayFrameBuffer;
this.getDisplaySizeOverride = getDisplaySizeOverride;
}
public OSVR_OpenGLToolkitFunctions(Pointer peer) {
super(peer);
}
public static class ByReference extends OSVR_OpenGLToolkitFunctions implements Structure.ByReference {
};
public static class ByValue extends OSVR_OpenGLToolkitFunctions implements Structure.ByValue {
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy