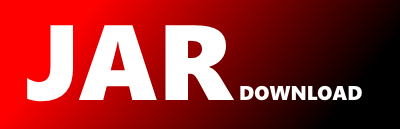
com.jme3.system.osvr.osvrtimevalue.OsvrTimeValueLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jme3-vr Show documentation
Show all versions of jme3-vr Show documentation
jMonkeyEngine is a 3-D game engine for adventurous Java developers
package com.jme3.system.osvr.osvrtimevalue;
import com.sun.jna.Library;
import com.sun.jna.Native;
import com.sun.jna.NativeLibrary;
import com.sun.jna.Pointer;
import com.sun.jna.PointerType;
/**
* JNA Wrapper for library osvrTimeValue
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java , Rococoa, or JNA.
*/
public class OsvrTimeValueLibrary implements Library {
public static final String JNA_LIBRARY_NAME = "osvrClientKit";
public static final NativeLibrary JNA_NATIVE_LIB = NativeLibrary.getInstance(OsvrTimeValueLibrary.JNA_LIBRARY_NAME);
static {
Native.register(OsvrTimeValueLibrary.class, OsvrTimeValueLibrary.JNA_NATIVE_LIB);
}
public static final int OSVR_TRUE = 1;
public static final int OSVR_FALSE = 0;
/**
* Gets the current time in the TimeValue. Parallel to gettimeofday.
* Original signature : void osvrTimeValueGetNow(OSVR_TimeValue*)
*/
public static native void osvrTimeValueGetNow(OSVR_TimeValue dest);
/**
* Converts from a TimeValue struct to your system's struct timeval.
* @param dest Pointer to an empty struct timeval for your platform.
* @param src A pointer to an OSVR_TimeValue you'd like to convert from.
* If either parameter is NULL, the function will return without doing
* anything.
* Original signature : void osvrTimeValueToStructTimeval(timeval*, const OSVR_TimeValue*)
*/
public static native void osvrTimeValueToStructTimeval(OsvrTimeValueLibrary.timeval dest, OSVR_TimeValue src);
/**
* Converts from a TimeValue struct to your system's struct timeval.
* @param dest An OSVR_TimeValue destination pointer.
* @param src Pointer to a struct timeval you'd like to convert from.
* The result is normalized.
* If either parameter is NULL, the function will return without doing
* anything.
* Original signature : void osvrStructTimevalToTimeValue(OSVR_TimeValue*, timeval*)
*/
public static native void osvrStructTimevalToTimeValue(OSVR_TimeValue dest, OsvrTimeValueLibrary.timeval src);
/**
* "Normalizes" a time value so that the absolute number of microseconds
* is less than 1,000,000, and that the sign of both components is the same.
* @param tv Address of a struct TimeValue to normalize in place.
* If the given pointer is NULL, this function returns without doing anything.
* Original signature : void osvrTimeValueNormalize(OSVR_TimeValue*)
*/
public static native void osvrTimeValueNormalize(OSVR_TimeValue tv);
/**
* Sums two time values, replacing the first with the result.
* @param tvA Destination and first source.
* @param tvB second source
* If a given pointer is NULL, this function returns without doing anything.
* Both parameters are expected to be in normalized form.
* Original signature : void osvrTimeValueSum(OSVR_TimeValue*, const OSVR_TimeValue*)
*/
public static native void osvrTimeValueSum(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
/**
* Computes the difference between two time values, replacing the first
* with the result.
* Effectively, `*tvA = *tvA - *tvB`
* @param tvA Destination and first source.
* @param tvB second source
* If a given pointer is NULL, this function returns without doing anything.
* Both parameters are expected to be in normalized form.
* Original signature : void osvrTimeValueDifference(OSVR_TimeValue*, const OSVR_TimeValue*)
*/
public static native void osvrTimeValueDifference(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
/**
* Compares two time values (assumed to be normalized), returning
* the same values as strcmp
* @return <0 if A is earlier than B, 0 if they are the same, and >0 if A
* is later than B.
* Original signature : int osvrTimeValueCmp(const OSVR_TimeValue*, const OSVR_TimeValue*)
*/
public static native int osvrTimeValueCmp(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
/**
* Compute the difference between the two time values, returning the
* duration as a double-precision floating-point number of seconds.
* Effectively, `ret = *tvA - *tvB`
* @param tvA first source.
* @param tvB second source
* @return Duration of timespan in seconds (floating-point)
* Original signature : double osvrTimeValueDurationSeconds(const OSVR_TimeValue*, const OSVR_TimeValue*)
*/
public static native double osvrTimeValueDurationSeconds(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
/**
* True if A is later than B.
* Original signature : OSVR_CBool osvrTimeValueGreater(const OSVR_TimeValue*, const OSVR_TimeValue*)
*/
public static native byte osvrTimeValueGreater(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
/**
* Returns true if the time value is normalized. Typically used in assertions.
* Original signature : bool osvrTimeValueIsNormalized(const OSVR_TimeValue&)
*/
public static native byte osvrTimeValueIsNormalized(OSVR_TimeValue tv);
/**
* Operator > overload for time values
* Original signature : bool operator>(const OSVR_TimeValue&, const OSVR_TimeValue&)
*/
public static native byte operatorGreater(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
/**
* Operator == overload for time values
* Original signature : bool operator==(const OSVR_TimeValue&, const OSVR_TimeValue&)
*/
public static native byte operatorIsEqual(OSVR_TimeValue tvA, OSVR_TimeValue tvB);
public static class timeval extends PointerType {
public timeval(Pointer address) {
super(address);
}
public timeval() {
super();
}
};
}