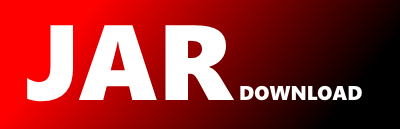
com.googlecode.jmxtrans.model.ResultFixtures Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmxtrans-core Show documentation
Show all versions of jmxtrans-core Show documentation
This module contains most of the core logic of JmxTrans.
/**
* The MIT License
* Copyright © 2010 JmxTrans team
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.googlecode.jmxtrans.model;
import com.google.common.collect.ImmutableList;
public final class ResultFixtures {
private ResultFixtures() {}
public static Result booleanTrueResult() {
return new Result(
0,
"Verbose",
"sun.management.MemoryImpl",
"ObjectDomainName",
"VerboseMemory",
"type=Memory",
ImmutableList.of(),
true);
}
public static ImmutableList singleTrueResult() {
return ImmutableList.of(booleanTrueResult());
}
public static Result booleanFalseResult() {
return new Result(
0,
"Verbose",
"sun.management.MemoryImpl",
"ObjectDomainName",
"VerboseMemory",
"type=Memory",
ImmutableList.of(),
false);
}
public static Result numericResult() {
return numericResult(10);
}
public static Result numericResult(Object numericValue) {
return new Result(
0,
"ObjectPendingFinalizationCount",
"sun.management.MemoryImpl",
"ObjectDomainName",
"MemoryAlias",
"type=Memory",
ImmutableList.of(),
numericValue);
}
public static Result numericResultWithColon() {
return numericResultWithColon(10);
}
public static Result numericResultWithColon(Object numericValue) {
return new Result(
0,
"Count",
"com.yammer.metrics.reporting.JmxReporter$Meter",
"fakehostname.example.com-org.openrepose.core",
null,
"type=\"ResponseCode\",scope=\"127.0.0.1:8008\",name=\"4XX\"",
ImmutableList.of(),
numericValue);
}
public static Result stringResult() {
return stringResult("value is a string");
}
public static Result stringResult(String value) {
return new Result(
0,
"NonHeapMemoryUsage",
"sun.management.MemoryImpl",
"ObjectDomainName",
"MemoryAlias",
"type=Memory",
ImmutableList.of("ObjectPendingFinalizationCount"),
value);
}
private static Result nonHeapMemoryResult(String valuePath, int value) {
return new Result(
0,
"NonHeapMemoryUsage",
"sun.management.MemoryImpl",
"ObjectDomainName",
"MemoryAlias",
"type=Memory",
ImmutableList.of(valuePath),
value);
}
public static ImmutableList hashResults() {
return ImmutableList.of(
nonHeapMemoryResult("committed", 12345),
nonHeapMemoryResult("init", 23456),
nonHeapMemoryResult("max", -1),
nonHeapMemoryResult("used", 45678));
}
public static Result numericBelowCPrecisionResult() {
return new Result(
0,
"ObjectPendingFinalizationCount",
"sun.management.MemoryImpl",
"ObjectDomainName",
"MemoryAlias",
"type=Memory",
ImmutableList.of(),
Double.MIN_VALUE);
}
public static Iterable singleNumericBelowCPrecisionResult() {
return ImmutableList.of(numericBelowCPrecisionResult());
}
public static Result numericResultWithTypenames(String typeName) {
return new Result(
0,
"ObjectPendingFinalizationCount",
"sun.management.MemoryImpl",
"ObjectDomainName",
"ObjectPendingFinalizationCount",
typeName,
ImmutableList.of(),
10);
}
public static ImmutableList singleFalseResult() {
return ImmutableList.of(booleanFalseResult());
}
public static ImmutableList singleNumericResult() {
return ImmutableList.of(numericResult());
}
public static ImmutableList dummyResults() {
return ImmutableList.of(
numericResult(),
booleanTrueResult(),
booleanFalseResult());
}
public static ImmutableList dummyResultWithColon() {
return ImmutableList.of(
numericResultWithColon(),
booleanTrueResult());
}
public static ImmutableList singleResult(Result result) {
return ImmutableList.of(result);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy