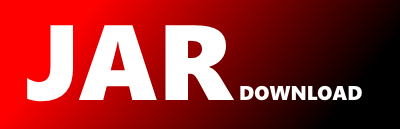
com.googlecode.jmxtrans.model.output.InfluxDbWriterFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmxtrans-output-influxdb Show documentation
Show all versions of jmxtrans-output-influxdb Show documentation
This module contains InfluxDB output writers.
The newest version!
/**
* The MIT License
* Copyright © 2010 JmxTrans team
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.googlecode.jmxtrans.model.output;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.googlecode.jmxtrans.model.OutputWriterFactory;
import com.googlecode.jmxtrans.model.ResultAttribute;
import com.googlecode.jmxtrans.model.ResultAttributes;
import com.googlecode.jmxtrans.model.output.support.ResultTransformerOutputWriter;
import lombok.AccessLevel;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import org.apache.commons.lang.StringUtils;
import org.influxdb.InfluxDB;
import org.influxdb.InfluxDBFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collections;
import java.util.List;
import static com.google.common.base.MoreObjects.firstNonNull;
import static java.lang.Boolean.FALSE;
import static java.lang.Boolean.TRUE;
@EqualsAndHashCode(exclude = {"influxDB"})
public class InfluxDbWriterFactory implements OutputWriterFactory {
private static final Logger LOG = LoggerFactory.getLogger(InfluxDbWriterFactory.class);
/**
* The deault
* The retention policy for each measuremen where no retentionPolicy
* setting is provided in the json config
*/
@VisibleForTesting
static final String DEFAULT_RETENTION_POLICY = "autogen";
@Getter(AccessLevel.PACKAGE)
private final String database;
@Getter(AccessLevel.PACKAGE)
private final InfluxDB.ConsistencyLevel writeConsistency;
@Getter(AccessLevel.PACKAGE)
private final ImmutableMap tags;
@Getter(AccessLevel.PACKAGE)
private final String retentionPolicy;
private final InfluxDB influxDB;
@Getter(AccessLevel.PACKAGE)
private final ImmutableSet resultAttributesToWriteAsTags;
@Getter(AccessLevel.PACKAGE)
private final boolean booleanAsNumber;
@Getter(AccessLevel.PACKAGE)
private final boolean typeNamesAsTags;
@Getter(AccessLevel.PACKAGE)
private final boolean createDatabase;
@Getter(AccessLevel.PACKAGE)
private final boolean reportJmxPortAsTag;
@Getter(AccessLevel.PACKAGE)
private final ImmutableList typeNames;
@Getter(AccessLevel.PACKAGE)
private final boolean allowStringValues;
/**
* @param typeNames - List of typeNames keys to use in fields by default
* @param booleanAsNumber - output boolean attributes as number
* @param url - The url e.g http://localhost:8086 to InfluxDB
* @param username - The username for InfluxDB
* @param password - The password for InfluxDB
* @param database - The name of the database (created if does not exist) on
* @param tags - Map of custom tags with custom values
* @param writeConsistency - The write consistency for InfluxDB.
* Valid values :
* - "ALL" (by default)
* - "ANY"
* - "ONE"
* - "QUORUM"
*
* @param retentionPolicy - The retention policy for InfluxDB
* @param resultTags - A list of meta-data from the result to add as tags. Sends all meta-data by default
* Available data :
* - "typeName"
* - "objDomain"
* - "className"
* - "attributeName"
*
* @param createDatabase - Creates the database in InfluxDB if not found
* @param reportJmxPortAsTag - Sends the JMX server port as tag instead of field
* @param typeNamesAsTags - Sends the given list of typeNames as tags instead of fields keys
* @param allowStringValues - Allows the OutputWriter to send String Values
*/
@JsonCreator
public InfluxDbWriterFactory(
@JsonProperty("typeNames") ImmutableList typeNames,
@JsonProperty("booleanAsNumber") boolean booleanAsNumber,
@JsonProperty("url") String url,
@JsonProperty("username") String username,
@JsonProperty("password") String password,
@JsonProperty("database") String database,
@JsonProperty("tags") ImmutableMap tags,
@JsonProperty("writeConsistency") String writeConsistency,
@JsonProperty("retentionPolicy") String retentionPolicy,
@JsonProperty("resultTags") List resultTags,
@JsonProperty("createDatabase") Boolean createDatabase,
@JsonProperty("reportJmxPortAsTag") Boolean reportJmxPortAsTag,
@JsonProperty("typeNamesAsTags") Boolean typeNamesAsTags,
@JsonProperty("allowStringValues") Boolean allowStringValues) {
this.typeNames = firstNonNull(typeNames,ImmutableList.of());
this.booleanAsNumber = booleanAsNumber;
this.database = database;
this.createDatabase = firstNonNull(createDatabase, TRUE);
this.typeNamesAsTags = firstNonNull(typeNamesAsTags, FALSE);
this.allowStringValues = firstNonNull(allowStringValues, FALSE);
this.writeConsistency = StringUtils.isNotBlank(writeConsistency)
? InfluxDB.ConsistencyLevel.valueOf(writeConsistency) : InfluxDB.ConsistencyLevel.ALL;
this.retentionPolicy = StringUtils.isNotBlank(retentionPolicy) ? retentionPolicy : DEFAULT_RETENTION_POLICY;
this.resultAttributesToWriteAsTags = initResultAttributesToWriteAsTags(resultTags);
this.tags = initCustomTagsMap(tags);
LOG.debug("Connecting to url: {} as: username: {}", url, username);
influxDB = InfluxDBFactory.connect(url, username, password);
this.reportJmxPortAsTag = firstNonNull(reportJmxPortAsTag, FALSE);
}
private ImmutableMap initCustomTagsMap(ImmutableMap tags) {
return ImmutableMap.copyOf(firstNonNull(tags, Collections.emptyMap()));
}
private ImmutableSet initResultAttributesToWriteAsTags(List resultTags) {
ImmutableSet result;
if (resultTags == null) {
result = ImmutableSet.copyOf(ResultAttributes.values());
} else {
result = ResultAttributes.forNames(resultTags);
}
LOG.debug("Result Tags to write set to: {}", result);
return result;
}
@Override
public ResultTransformerOutputWriter create() {
return ResultTransformerOutputWriter.booleanToNumber(booleanAsNumber, new InfluxDbWriter(influxDB, database,
writeConsistency, retentionPolicy, tags, resultAttributesToWriteAsTags, typeNames, createDatabase, reportJmxPortAsTag, typeNamesAsTags, allowStringValues));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy