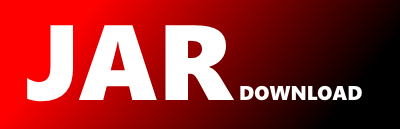
org.jnario.compiler.JnarioDocCompiler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/**
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.jnario.compiler;
import com.google.common.base.Predicate;
import com.google.inject.Inject;
import java.io.File;
import java.util.List;
import java.util.Map;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.common.util.URI;
import org.eclipse.emf.ecore.resource.Resource;
import org.eclipse.emf.ecore.resource.ResourceSet;
import org.eclipse.emf.ecore.util.EcoreUtil;
import org.eclipse.xtend.core.compiler.batch.XtendBatchCompiler;
import org.eclipse.xtext.generator.JavaIoFileSystemAccess;
import org.eclipse.xtext.mwe.NameBasedFilter;
import org.eclipse.xtext.mwe.PathTraverser;
import org.eclipse.xtext.parser.IEncodingProvider;
import org.eclipse.xtext.resource.XtextResource;
import org.jnario.doc.AbstractDocGenerator;
import org.jnario.doc.DocOutputConfigurationProvider;
import org.jnario.report.Executable2ResultMapping;
@SuppressWarnings("all")
public class JnarioDocCompiler extends XtendBatchCompiler {
private Executable2ResultMapping resultMapping;
private String _resultFolder;
public String getResultFolder() {
return this._resultFolder;
}
public void setResultFolder(final String resultFolder) {
this._resultFolder = resultFolder;
}
@Inject
private AbstractDocGenerator docGenerator;
@Inject
private IEncodingProvider.Runtime encodingProvider;
private ResourceSet resourceSet;
public boolean compile() {
this.loadResources();
this.generateDocumentation(this.resultMapping);
return true;
}
@Inject
public Executable2ResultMapping setExecutable2ResultMapping(final Executable2ResultMapping resultMapping) {
return this.resultMapping = resultMapping;
}
public ResourceSet loadResources() {
ResourceSet _xblockexpression = null;
{
ResourceSet _get = this.resourceSetProvider.get();
this.resourceSet = _get;
String _fileEncoding = this.getFileEncoding();
this.encodingProvider.setDefaultEncoding(_fileEncoding);
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy