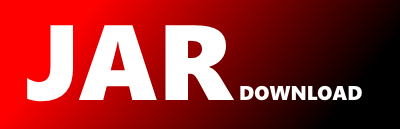
org.jnario.doc.HtmlFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/**
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.jnario.doc;
import java.util.List;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.jnario.doc.HtmlAssets;
@SuppressWarnings("all")
public class HtmlFile {
public static HtmlFile EMPTY_FILE = new HtmlFile();
public static HtmlFile newHtmlFile(final Procedure1 initializer) {
final HtmlFile htmlFile = new HtmlFile();
initializer.apply(htmlFile);
return htmlFile;
}
private HtmlAssets _assets = new HtmlAssets();
public HtmlAssets getAssets() {
return this._assets;
}
public void setAssets(final HtmlAssets assets) {
this._assets = assets;
}
private CharSequence _name = "";
public CharSequence getName() {
return this._name;
}
public void setName(final CharSequence name) {
this._name = name;
}
private CharSequence _title = "";
public CharSequence getTitle() {
return this._title;
}
public void setTitle(final CharSequence title) {
this._title = title;
}
private CharSequence _content = "";
public CharSequence getContent() {
return this._content;
}
public void setContent(final CharSequence content) {
this._content = content;
}
private String _rootFolder = "";
public String getRootFolder() {
return this._rootFolder;
}
public void setRootFolder(final String rootFolder) {
this._rootFolder = rootFolder;
}
private CharSequence _sourceCode = "";
public CharSequence getSourceCode() {
return this._sourceCode;
}
public void setSourceCode(final CharSequence sourceCode) {
this._sourceCode = sourceCode;
}
private CharSequence _fileName = "";
public CharSequence getFileName() {
return this._fileName;
}
public void setFileName(final CharSequence fileName) {
this._fileName = fileName;
}
private String _executionStatus = "";
public String getExecutionStatus() {
return this._executionStatus;
}
public void setExecutionStatus(final String executionStatus) {
this._executionStatus = executionStatus;
}
public CharSequence toText() {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.append("");
CharSequence _title = this.getTitle();
_builder.append(_title, "");
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append("");
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.newLine();
{
HtmlAssets _assets = this.getAssets();
List _cssFiles = _assets.getCssFiles();
for(final String cssFile : _cssFiles) {
_builder.append("");
_builder.newLineIfNotEmpty();
}
}
{
HtmlAssets _assets_1 = this.getAssets();
List _jsFiles = _assets_1.getJsFiles();
for(final String jsFile : _jsFiles) {
_builder.append("");
_builder.newLineIfNotEmpty();
}
}
_builder.append("");
_builder.newLine();
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t");
_builder.append("");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t\t\t");
_builder.append("");
CharSequence _title_1 = this.getTitle();
_builder.append(_title_1, "\t\t\t\t\t");
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t\t\t ");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t\t\t ");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t\t\t\t \t");
_builder.append("");
_builder.newLine();
CharSequence _content = this.getContent();
_builder.append(_content, "");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t\t\t\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t\t\t ");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t\t\t \t");
_builder.append("");
CharSequence _fileName = this.getFileName();
_builder.append(_fileName, "\t\t\t\t\t\t \t");
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t\t\t\t \t");
_builder.append("");
_builder.newLine();
CharSequence _sourceCode = this.getSourceCode();
_builder.append(_sourceCode, "");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t\t\t\t ");
_builder.append("
");
_builder.newLine();
_builder.append("\t\t\t\t\t\t ");
_builder.append("");
_builder.newLine();
_builder.append("\t\t\t\t\t");
_builder.append(" ");
_builder.newLine();
_builder.append("\t\t\t\t");
_builder.append(" ");
_builder.newLine();
_builder.append("\t\t\t");
_builder.append(" ");
_builder.newLine();
_builder.append("\t\t");
_builder.append(" ");
_builder.newLine();
_builder.append("\t\t");
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append(" ");
_builder.newLine();
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.append("");
_builder.newLine();
return _builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy