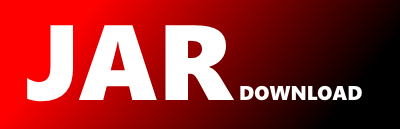
org.jnario.impl.ExampleColumnImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jnario.standalone Show documentation
Show all versions of org.jnario.standalone Show documentation
The required libraries to execute Jnario specifications without Eclipse.
The newest version!
/*******************************************************************************
* Copyright (c) 2012 BMW Car IT and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.jnario.impl;
import java.util.Collection;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.EObjectImpl;
import org.eclipse.emf.ecore.util.EObjectResolvingEList;
import org.eclipse.emf.ecore.util.EcoreUtil;
import org.eclipse.xtext.common.types.JvmTypeReference;
import org.jnario.ExampleCell;
import org.eclipse.xtext.xbase.XExpression;
import org.jnario.ExampleColumn;
import org.jnario.ExampleRow;
import org.jnario.ExampleTable;
import org.jnario.JnarioPackage;
/**
*
* An implementation of the model object 'Example Column'.
*
*
* The following features are implemented:
*
* - {@link org.jnario.impl.ExampleColumnImpl#getTable Table}
* - {@link org.jnario.impl.ExampleColumnImpl#getCells Cells}
* - {@link org.jnario.impl.ExampleColumnImpl#getName Name}
* - {@link org.jnario.impl.ExampleColumnImpl#getType Type}
*
*
*
* @generated
*/
public class ExampleColumnImpl extends EObjectImpl implements ExampleColumn {
/**
* The default value of the '{@link #getName() Name}' attribute.
*
*
* @see #getName()
* @generated
* @ordered
*/
protected static final String NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getName() Name}' attribute.
*
*
* @see #getName()
* @generated
* @ordered
*/
protected String name = NAME_EDEFAULT;
/**
* The cached value of the '{@link #getType() Type}' containment reference.
*
*
* @see #getType()
* @generated
* @ordered
*/
protected JvmTypeReference type;
/**
*
*
* @generated
*/
protected ExampleColumnImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return JnarioPackage.Literals.EXAMPLE_COLUMN;
}
/**
*
*
* @generated
*/
public ExampleTable getTable() {
if (eContainerFeatureID() != JnarioPackage.EXAMPLE_COLUMN__TABLE) return null;
return (ExampleTable)eInternalContainer();
}
/**
*
*
* @generated
*/
public NotificationChain basicSetTable(ExampleTable newTable, NotificationChain msgs) {
msgs = eBasicSetContainer((InternalEObject)newTable, JnarioPackage.EXAMPLE_COLUMN__TABLE, msgs);
return msgs;
}
/**
*
*
* @generated
*/
public void setTable(ExampleTable newTable) {
if (newTable != eInternalContainer() || (eContainerFeatureID() != JnarioPackage.EXAMPLE_COLUMN__TABLE && newTable != null)) {
if (EcoreUtil.isAncestor(this, newTable))
throw new IllegalArgumentException("Recursive containment not allowed for " + toString());
NotificationChain msgs = null;
if (eInternalContainer() != null)
msgs = eBasicRemoveFromContainer(msgs);
if (newTable != null)
msgs = ((InternalEObject)newTable).eInverseAdd(this, JnarioPackage.EXAMPLE_TABLE__COLUMNS, ExampleTable.class, msgs);
msgs = basicSetTable(newTable, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, JnarioPackage.EXAMPLE_COLUMN__TABLE, newTable, newTable));
}
/**
*
*
* @generated NOT
*/
public EList getCells() {
EList cells = new EObjectResolvingEList(ExampleCell.class, this, JnarioPackage.EXAMPLE_COLUMN__CELLS);
int columnIndex = getTable().getColumns().indexOf(this);
for (ExampleRow row : getTable().getRows()) {
if(row.getCells().size() > columnIndex){
cells.add(row.getCells().get(columnIndex));
}
}
return cells;
}
/**
*
*
* @generated
*/
public String getName() {
return name;
}
/**
*
*
* @generated
*/
public void setName(String newName) {
String oldName = name;
name = newName;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, JnarioPackage.EXAMPLE_COLUMN__NAME, oldName, name));
}
/**
*
*
* @generated
*/
public JvmTypeReference getType() {
return type;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetType(JvmTypeReference newType, NotificationChain msgs) {
JvmTypeReference oldType = type;
type = newType;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, JnarioPackage.EXAMPLE_COLUMN__TYPE, oldType, newType);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setType(JvmTypeReference newType) {
if (newType != type) {
NotificationChain msgs = null;
if (type != null)
msgs = ((InternalEObject)type).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - JnarioPackage.EXAMPLE_COLUMN__TYPE, null, msgs);
if (newType != null)
msgs = ((InternalEObject)newType).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - JnarioPackage.EXAMPLE_COLUMN__TYPE, null, msgs);
msgs = basicSetType(newType, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, JnarioPackage.EXAMPLE_COLUMN__TYPE, newType, newType));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseAdd(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
if (eInternalContainer() != null)
msgs = eBasicRemoveFromContainer(msgs);
return basicSetTable((ExampleTable)otherEnd, msgs);
}
return super.eInverseAdd(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
return basicSetTable(null, msgs);
case JnarioPackage.EXAMPLE_COLUMN__TYPE:
return basicSetType(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eBasicRemoveFromContainerFeature(NotificationChain msgs) {
switch (eContainerFeatureID()) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
return eInternalContainer().eInverseRemove(this, JnarioPackage.EXAMPLE_TABLE__COLUMNS, ExampleTable.class, msgs);
}
return super.eBasicRemoveFromContainerFeature(msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
return getTable();
case JnarioPackage.EXAMPLE_COLUMN__CELLS:
return getCells();
case JnarioPackage.EXAMPLE_COLUMN__NAME:
return getName();
case JnarioPackage.EXAMPLE_COLUMN__TYPE:
return getType();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
setTable((ExampleTable)newValue);
return;
case JnarioPackage.EXAMPLE_COLUMN__CELLS:
getCells().clear();
getCells().addAll((Collection extends ExampleCell>)newValue);
return;
case JnarioPackage.EXAMPLE_COLUMN__NAME:
setName((String)newValue);
return;
case JnarioPackage.EXAMPLE_COLUMN__TYPE:
setType((JvmTypeReference)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
setTable((ExampleTable)null);
return;
case JnarioPackage.EXAMPLE_COLUMN__CELLS:
getCells().clear();
return;
case JnarioPackage.EXAMPLE_COLUMN__NAME:
setName(NAME_EDEFAULT);
return;
case JnarioPackage.EXAMPLE_COLUMN__TYPE:
setType((JvmTypeReference)null);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case JnarioPackage.EXAMPLE_COLUMN__TABLE:
return getTable() != null;
case JnarioPackage.EXAMPLE_COLUMN__CELLS:
return !getCells().isEmpty();
case JnarioPackage.EXAMPLE_COLUMN__NAME:
return NAME_EDEFAULT == null ? name != null : !NAME_EDEFAULT.equals(name);
case JnarioPackage.EXAMPLE_COLUMN__TYPE:
return type != null;
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString() {
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (name: ");
result.append(name);
result.append(')');
return result.toString();
}
} //ExampleColumnImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy